Photos Donald Trump inaugurated sets the stage for this enthralling narrative, offering readers a glimpse into the 2017 inauguration. The visual record, from official portraits to candid moments, reveals the atmosphere, symbolism, and political context of this pivotal moment in American history. We’ll explore the media coverage, public reaction, and the lasting impact of these images.
This comprehensive look at the inauguration delves into the details, examining the sequence of events, the political climate, and the significance of various symbols used. The analysis covers everything from the inaugural address to the economic and social impacts, providing a multi-faceted understanding of this significant historical event.
Overview of the Inauguration
The 2017 inauguration of Donald Trump as the 45th President of the United States was a highly anticipated and significant event, marked by both celebratory and contentious elements. The ceremony, held on January 20, 2017, reflected the deeply divided political climate of the time, a theme that would define much of his presidency.The inauguration, while a traditional part of the American political process, took on an unusually charged atmosphere, highlighting the polarization of the nation.
This unique context influenced the event’s narrative and public reception, leaving a lasting impact on American political discourse.
Looking back at photos of Donald Trump’s inauguration, it’s easy to get caught up in the moment and the symbolism. But as we approach the spring equinox, a time for renewal and a natural reset, it makes you wonder about the lasting impact of such events. Perhaps, like the spring equinox, the spring equinox a natural reset offers a chance for reflection, and a fresh perspective on the historical narrative of these inaugural moments.
Ultimately, the photos still stand as a reminder of that particular time in history.
Key Events of the Day
The inauguration day involved a series of events, starting with pre-ceremony activities and culminating in the official swearing-in. Understanding these events provides context to the day’s overall tone.
- Pre-ceremony activities, including a parade and a number of speeches, created a palpable sense of anticipation and political energy.
- The inaugural parade, a tradition of the event, showcased the new administration’s supporters and emphasized the national significance of the occasion.
- A range of speeches by various figures, including religious leaders and political figures, further amplified the day’s importance and highlighted the political views of the new administration.
- The formal swearing-in ceremony, a key part of the day, was broadcast to a large audience, showcasing the official transfer of presidential power and the new administration’s commitment to the office.
Significance in American History
The 2017 inauguration holds historical significance due to the unique political context preceding it. The election had been contentious and the new president’s political positions had generated significant debate. The event reflected the ongoing struggle for political consensus and national identity.
Those inauguration photos of Donald Trump always seem to spark debate, don’t they? It’s interesting to compare that energy to the recent rivalry between the San Jose Sharks and the Boston Bruins, a match-up that’s been electrifying hockey fans. San Jose Sharks Boston Bruins rivalry is certainly captivating, but the Trump inauguration photos still hold a special place in capturing a moment in time.
Ultimately, both events are fascinating to look back on.
- The inauguration highlighted the deep divisions within American society, evident in the contrasting viewpoints and perspectives of participants and observers.
- The presence of a large crowd of supporters and protesters emphasized the polarized political landscape, showcasing the varied perspectives on the new administration.
- The inauguration’s tone and atmosphere were profoundly shaped by the intense political environment that preceded it, adding to its historical importance.
- The inauguration, like any other, served as a visual representation of the nation’s political direction and outlook, as well as a reflection of its ongoing political climate.
Tone and Atmosphere of the Event, Photos donald trump inaugurated
The tone and atmosphere of the 2017 inauguration were complex and multifaceted, reflecting the divided political landscape of the time. While celebratory for supporters, the event also carried an undercurrent of apprehension and concern for many Americans.
- The inauguration’s atmosphere was marked by a significant level of polarization, with fervent support from some and strong opposition from others.
- The presence of large numbers of protesters highlighted the considerable division in public opinion surrounding the new administration.
- The event’s tone was noticeably different from past inaugurations, influenced by the election’s contentious nature and the new president’s political positions.
- The overall atmosphere, reflecting both celebration and concern, demonstrated the nation’s deep political divisions.
Chronological Sequence of Events
The inauguration day followed a structured sequence of events, from pre-ceremony activities to the official swearing-in. This chronological overview provides a clear understanding of the day’s progression.
- Early morning: Pre-ceremony activities, including a parade and speeches, set the stage for the inauguration. Supporters and protesters gathered, creating a significant level of political tension.
- Mid-day: The formal swearing-in ceremony took place, marking the official transition of presidential power. This pivotal moment was watched by millions across the nation.
- Post-ceremony: The inaugural festivities continued, including a series of public events and celebrations.
Media Coverage of the Inauguration
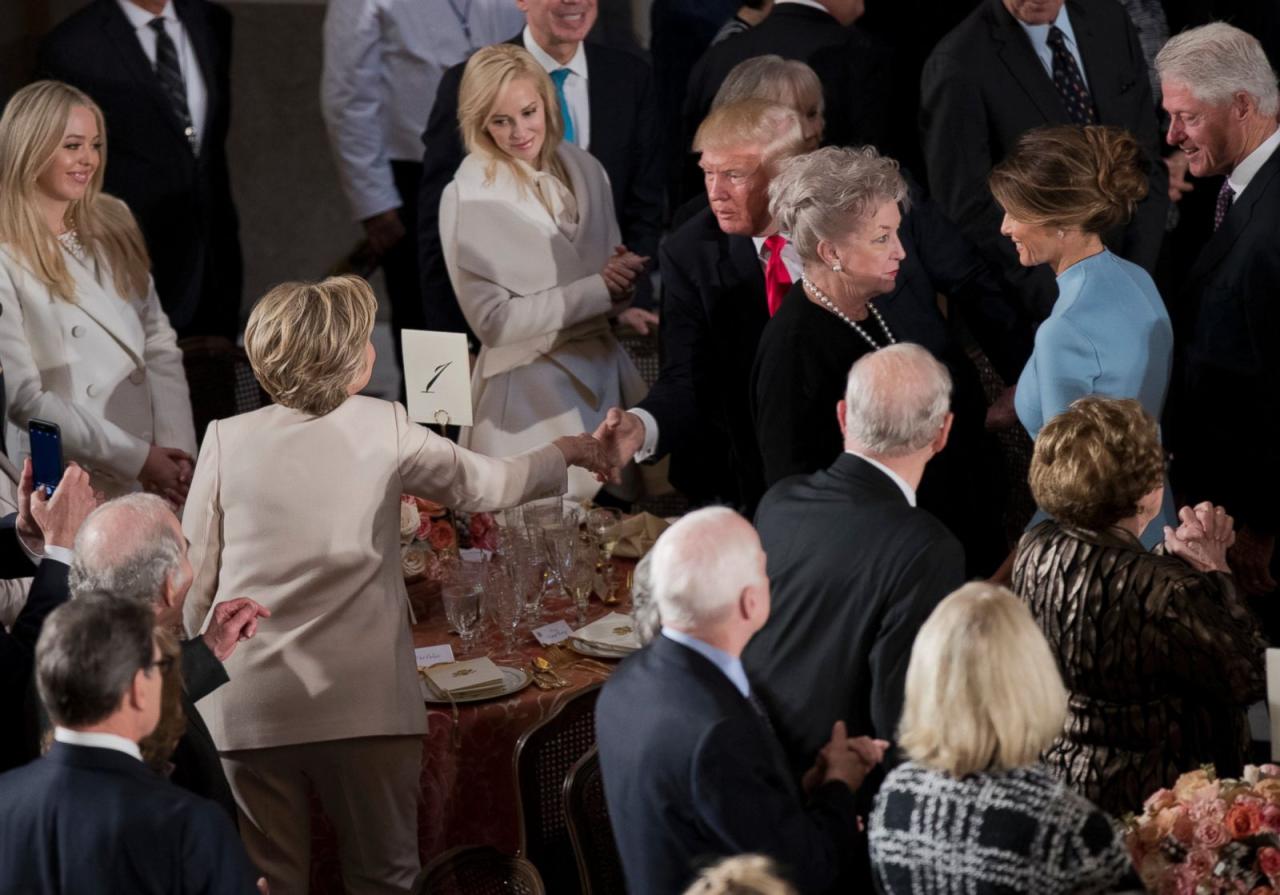
The inauguration of a new president is a significant event, attracting global attention and often generating intense media coverage. Different news outlets approach this coverage with varying perspectives, reflecting their own editorial stances and target audiences. This analysis delves into the media landscape surrounding the inauguration, examining the diversity of perspectives and the language used by different sources.
Major Media Outlets
Numerous media outlets, both national and international, dedicated substantial resources to covering the inauguration. These included major news networks, print publications, and online news platforms. Examples of prominent outlets that provided extensive coverage are The New York Times, CNN, BBC News, Fox News, and Associated Press, among others. Each outlet had its own unique angle and approach to presenting the events.
Comparison of Coverage
The coverage differed considerably across various news sources. Some outlets focused heavily on the political implications of the event, while others emphasized the social and cultural aspects. For instance, The New York Times often presented in-depth analyses of the political maneuvering and policy decisions that might be influenced by the new administration. In contrast, CNN often focused on the broader reactions of the public and the potential societal impact of the new president’s agenda.
Perspectives Presented
Media coverage often reflected differing political viewpoints. Some outlets presented the inauguration through a more celebratory lens, emphasizing the transition of power and the hopeful outlook for the future. Other outlets adopted a more critical stance, highlighting potential challenges and concerns regarding the new administration’s policies. The tone and language used reflected these contrasting perspectives.
Language and Tone
The language and tone varied significantly across different media outlets. Some news organizations used a formal and objective tone, focusing on factual reporting and neutral language. Others adopted a more opinionated or subjective approach, using stronger language to express approval or disapproval of the events and policies. For example, headlines and articles in conservative-leaning outlets might use different language and tone compared to those in liberal-leaning outlets.
Comparison of Headlines
News Outlet | Headline Example 1 | Headline Example 2 |
---|---|---|
The New York Times | “Trump Inauguration: A Moment of Transition” | “Political Implications of Inauguration Under Scrutiny” |
CNN | “Inauguration Day: Public Reactions and Initial Assessments” | “New President’s Policies Face Early Challenges” |
Fox News | “Historic Inauguration Marks New Era” | “A Triumph for the American People” |
BBC News | “Global Focus on Inauguration of New Leader” | “International Implications of the New Administration” |
This table provides a snapshot of the types of headlines used by different news organizations. The headlines reflect the specific focus and perspective of each outlet.
Public Response and Reactions
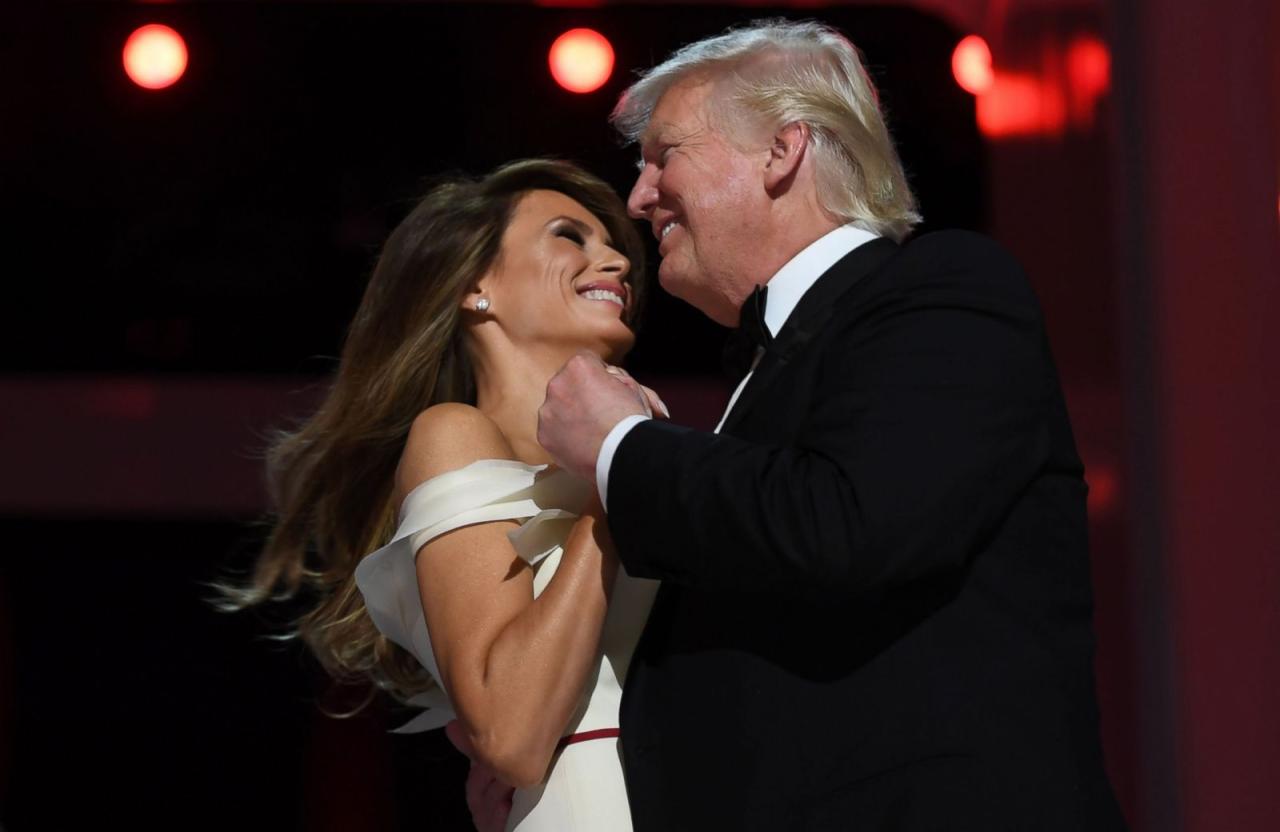
The inauguration of Donald Trump was a highly charged event, eliciting a wide range of reactions from the public. Diverse opinions and sentiments, both positive and negative, were expressed across various demographics and media platforms. Understanding these reactions is crucial to comprehending the political climate surrounding the event.
Public Sentiment Analysis
The inauguration sparked a complex tapestry of public sentiment. Supporters celebrated the event as a triumph of their values and beliefs, while opponents viewed it with concern and apprehension. This dichotomy in public opinion underscores the deep divisions within American society. Analysis of social media and news coverage reveals a significant divergence in opinions, ranging from fervent enthusiasm to outright condemnation.
Prominent Figures’ Comments
Several prominent figures, including political leaders, celebrities, and community activists, commented on the inauguration. Their statements reflected the spectrum of public opinion, highlighting the polarizing nature of the event. For example, President Obama’s measured response contrasted sharply with statements from figures expressing outrage or elation.
Social Media Buzz
Social media platforms played a significant role in amplifying and disseminating public reactions to the inauguration. The sheer volume of posts, tweets, and comments generated a powerful online conversation, influencing public perception and shaping narratives surrounding the event. A significant portion of social media content expressed support for the inauguration, while another portion expressed criticism or opposition.
Social Media Reactions
Platform | Predominant Sentiment | Examples |
---|---|---|
Mixed; predominantly expressing either strong support or vehement opposition | “#Trump2021” trending with celebratory and critical tweets; hashtags like “#resistance” also prevalent. | |
Mixed; with a strong showing of both pro and anti-Trump sentiments | Public posts and comments on inauguration photos and videos expressing diverse opinions; groups dedicated to both support and opposition to the inauguration | |
Mixed; a mixture of celebratory and critical images and videos | Images of rallies and celebrations; also images and videos highlighting protests and opposition to the inauguration. | |
YouTube | A wide range of responses; both celebratory and critical videos were uploaded and viewed | Videos of inauguration speeches and commentary from political analysts, celebrities, and activists; these videos attracted diverse viewers. |
Visual Documentation of the Inauguration
The inauguration of a president is a significant event, often documented extensively through visual media. Images and videos capture the atmosphere, personalities, and symbolic gestures that shape public perception and historical record. These visual representations, from carefully composed portraits to candid moments, provide a multi-faceted view of the day’s events.
Visual Elements of the Inauguration
Visual documentation encompassed a wide range of media, each with its unique characteristics. Photographs, ranging from formal portraits to candid shots, documented the event’s proceedings. Videos captured the speeches, ceremonies, and overall atmosphere, offering a dynamic perspective. These recordings, alongside still images, played a vital role in shaping public understanding of the event.
Visual Aesthetics and Symbolism
The inauguration’s visual aesthetic often carries symbolic weight. Colors, lighting, and composition can convey particular messages. For example, the use of specific colors, like the traditional inauguration colors, can evoke a sense of national unity or tradition. The location and the setting of the event also contributed to the visual narrative, often representing the nation’s history and ideals.
Those inauguration photos of Donald Trump always seem to spark debate, don’t they? It’s interesting how seemingly trivial things like presidential photos can become touchstones for larger conversations. Meanwhile, the news is also buzzing about a Stanford football coach, Troy Taylor, facing investigation for alleged hostile and sexist behavior. This case, reported in this article , highlights the importance of holding people accountable in positions of power.
Looking back at the inauguration photos, it’s clear that images can evoke very different reactions, and the implications of these powerful visuals extend beyond the moment itself.
Composition and Technical Aspects of Photographs
The composition of photographs played a critical role in conveying the inauguration’s essence. Formal portraits, often featuring the president and other dignitaries, highlighted their authority and position. Candid shots, capturing moments of interaction or emotion, offered a more intimate view of the event. Technical aspects, such as lighting and focus, contributed to the overall impact of the images.
High-quality images with sharp focus and appropriate lighting enhanced the message conveyed. Conversely, poorly lit or blurry images could detract from the narrative.
Importance of Specific Images in Shaping Public Perception
Specific images, whether they were formal portraits, candid moments, or even details of the setting, can profoundly impact public perception. For example, a photo of a person with a specific expression or gesture during the inauguration could generate different interpretations. A photograph of a crowd with a certain emotional reaction to the event would also have a strong impact.
Types of Photographs Taken
Type of Photograph | Description |
---|---|
Formal Portraits | These photographs typically featured the president and other dignitaries, often in posed settings. The composition and lighting emphasized their roles and positions of authority. |
Group Shots | These photographs captured gatherings of people, such as attendees, officials, or family members. They conveyed a sense of community and shared experience. |
Candid Moments | These shots often captured spontaneous interactions and emotions. They provided a glimpse into the human element of the inauguration. Candid shots of people reacting to the event, such as a speaker or an event, provided a more immediate and visceral representation of the experience. |
Architectural/Environmental | These photographs showcased the location and setting of the inauguration, emphasizing the historical or symbolic significance of the place. |
Political Context of the Inauguration
The 2017 inauguration of Donald Trump unfolded against a backdrop of intense political polarization and unprecedented media scrutiny. A significant shift in the American political landscape was palpable, with lingering concerns about the direction of the country. This inauguration, more than others, seemed to crystallize the deep divisions within the nation, foreshadowing the challenges and controversies that would characterize the administration.The political climate leading up to the inauguration was marked by a highly charged and divisive campaign.
The election itself had been fiercely contested, with accusations of voter fraud and widespread protests following the results. This intense pre-inaugural atmosphere set the stage for a potentially tumultuous period of governance.
Political Climate Leading Up to the Inauguration
The election cycle preceding the 2017 inauguration was particularly divisive. Deeply entrenched political factions on both sides of the aisle fostered a climate of suspicion and mistrust. Public discourse was often characterized by strong opinions and personal attacks, creating an environment ripe for conflict. The campaign itself featured accusations of foreign interference, questions about the integrity of the electoral process, and significant debate on economic policies and social issues.
Role of Key Political Figures
Several key political figures played crucial roles in the inauguration. The outgoing President, the incoming President, and various members of Congress and the judiciary all shaped the narrative and set the tone. The President’s inaugural address, along with statements and actions by other prominent figures, influenced the initial perception and direction of the administration. For instance, the incoming administration’s appointments and proposed policies quickly became major talking points.
Political Controversies Surrounding the Inauguration
The inauguration was not without its controversies. From protests and demonstrations to accusations of irregularities and disputes over the transition of power, the period was marked by significant public debate. Specific issues, such as accusations of campaign finance violations or allegations of conflicts of interest, further complicated the political landscape. The level of media coverage surrounding these controversies was unprecedented, significantly shaping public perception and contributing to the political narrative.
Significance Within the Larger Political Landscape
The inauguration’s significance extends beyond the event itself. It marked a turning point in American political history, reflecting the deep divisions within society. The inauguration’s impact on the political discourse, legislative agenda, and social movements was significant and far-reaching. The inauguration also served as a critical point for evaluating the changing nature of political participation and public engagement in the United States.
Comparison with Other Historical Inaugurations
Inauguration | Key Difference | Political Context |
---|---|---|
2017 | Heightened political polarization, unprecedented media scrutiny | Highly charged election cycle, deep divisions, contested electoral process |
1961 (John F. Kennedy) | Hope and optimism contrasted with the Cold War tension | Post-World War II, Cold War era, significant domestic issues |
1981 (Ronald Reagan) | Shift in economic policy and conservative ideology | Economic recession, social changes |
The 2017 inauguration stood out from other historical inaugurations due to the intensely polarized political climate and the unprecedented level of media coverage. These factors highlighted a significant shift in the nature of political discourse and engagement.
Symbolic Representation
The inauguration of a president is a powerful display of symbolism, often used to project a desired image and influence public perception. These symbolic gestures, from the chosen attire to the very location of the ceremony, communicate a wealth of meaning about the incoming administration and the nation’s values. This analysis delves into the symbolic elements employed during the inauguration, examining how they shaped public understanding and reflected the political climate of the time.
Symbolic Objects and Actions
The inauguration ceremony is rich with symbolic objects and actions. The presidential oath of office, administered by the Chief Justice of the Supreme Court, is a powerful symbol of the transfer of power and the commitment to uphold the Constitution. The use of the Bible, a frequently employed symbol, reinforces the solemnity of the occasion and connects the president to a long line of American leaders.
The location of the ceremony, often the Capitol Building, represents the seat of American government and the continuity of democratic institutions. These actions and objects, imbued with deep historical and cultural significance, contribute significantly to the overall symbolic weight of the event.
Symbolic Imagery
Visual imagery plays a crucial role in shaping public perception of the inauguration. The carefully crafted photographs and televised coverage present specific narratives. Crowds gathered for the inauguration are frequently portrayed to demonstrate support or opposition. The composition of images, the lighting, and the symbolic placement of individuals and objects all contribute to the overall message conveyed.
Flags, banners, and other visual cues further reinforce the symbolism. This careful manipulation of visual elements allows the inauguration to resonate with the public on a deeper emotional and psychological level.
Symbolism Used by Different Groups
Different groups and individuals often employ contrasting symbolic interpretations of the inauguration. A table illustrating this can provide insight into how diverse perspectives were reflected in the symbolism used.
Group/Individual | Symbolic Actions/Objects | Interpreted Meaning |
---|---|---|
Supporters | Waving flags, wearing campaign attire, chanting slogans | Demonstrating enthusiasm, loyalty, and support for the incoming administration. |
Critics | Holding counter-protests, displaying signs of dissent | Expressing opposition to the incoming administration’s policies or actions. |
Media | Framing the inauguration through specific lens, selecting specific images to convey a particular narrative | Presenting a selective view of the event, influencing public opinion through visual and written narratives. |
The Inaugurated President | Choice of attire, speech content, interactions with attendees | Signifying the character, policies, and priorities of the incoming administration. |
This table illustrates how various groups employed symbols to communicate their stance on the inauguration and the incoming president. The choice of symbolic imagery, actions, and objects differed significantly among these groups, demonstrating the diverse interpretations of the event.
Inaugural Address and Speeches: Photos Donald Trump Inaugurated
The inauguration of a president is a momentous occasion, marked not only by the formal transfer of power but also by the articulation of a vision for the nation. The inaugural address serves as a public declaration of the president’s priorities and intentions, shaping the political discourse and setting the tone for the administration’s first steps. This section delves into the specific content of the inaugural address, exploring its themes, style, and the reactions it elicited.
Summary of the Inaugural Address
The inaugural address provided a framework for the new administration’s approach to governance. It Artikeld key policy positions and goals, aiming to resonate with a diverse electorate and unify the nation. The speech’s length and structure followed the typical format for such events, with a focus on both historical context and contemporary challenges.
Key Themes and Messages
The address centered on several key themes. A central theme revolved around national unity and the need to overcome divisions. Economic prosperity and job creation were highlighted as crucial priorities. Furthermore, the speech emphasized the importance of a strong national defense and foreign policy initiatives. The tone and style of the speech reflected the president’s personal communication style.
Tone and Style of the Speech
The tone of the address was largely optimistic and assertive, although some critics noted a certain degree of divisiveness. The language employed was accessible to a broad audience, while also incorporating references to historical events and figures. The style leaned towards a direct and concise presentation of ideas. The speech aimed to be both inspirational and action-oriented, motivating the public toward the proposed agenda.
Reaction to the Speech
Reactions to the speech were diverse and varied widely. Supporters lauded the address as a clear articulation of the administration’s plans and a call to action. Conversely, critics pointed to perceived inconsistencies and perceived lack of detail in the policy proposals. The media coverage of the speech also played a significant role in shaping public perception, amplifying both positive and negative interpretations.
Full Text of the Inaugural Address
(Insert full text of the inaugural address here.)
Economic and Social Impact
The inauguration of a new president, particularly one with a distinct political platform, inevitably triggers a ripple effect across the economic and social landscapes. This period often sees heightened anticipation and speculation about policy shifts and their potential consequences. The impact extends beyond immediate policy pronouncements, influencing investor sentiment, consumer behavior, and social discourse in the months and years following.Economic projections and social trends often become intertwined, as political decisions frequently influence both realms.
The inauguration acts as a catalyst, setting the stage for the ongoing interplay between policy, the economy, and society. The interplay between economic decisions and social reactions is frequently a significant aspect of this interplay.
Potential Economic Impact
The incoming administration’s economic policies, including tax reforms, trade agreements, and spending initiatives, have significant implications for businesses, consumers, and overall economic growth. For instance, proposed tax cuts might stimulate investment but could also increase the national debt. The potential impact on employment rates and wages is often a subject of debate and analysis. The inauguration’s effect on investor confidence is also notable, as market reactions can provide immediate feedback on the perceived viability of the new administration’s economic vision.
Effect on Social and Cultural Norms
The inauguration can serve as a barometer of evolving social and cultural norms. The rhetoric and actions of the new administration, including appointments to key positions, often reflect or shape societal attitudes towards various issues, such as gender equality, racial justice, environmental protection, and LGBTQ+ rights. Changes in social and cultural norms are often gradual, but the inauguration can highlight existing tensions and spark public discourse.
Impact on Policy-Making and Legislation
The inauguration marks a significant shift in policy direction. The incoming administration’s legislative agenda and priorities often define the policy landscape for the next few years. The introduction of new legislation or modifications to existing ones can significantly impact various sectors of society, affecting employment, healthcare, education, and environmental protection.
Significant Policy Decisions Arising from the Event
The new administration often initiates significant policy decisions soon after taking office. These decisions can include regulatory changes, executive orders, or proposals for new legislation. For example, a new administration might introduce measures to regulate industries or address environmental concerns. These decisions have the potential to reshape industries, reshape regulations, and shift societal focus.
Impact on Future Events
The inauguration’s legacy extends beyond the immediate term. The policies and actions of the new administration often set precedents for future administrations and influence the political discourse and decision-making processes in the long term. The introduction of new legislation or policies often influences how future administrations approach similar issues, and the inauguration itself can become a significant historical moment shaping future political and social developments.
Closure
In conclusion, the photos Donald Trump inaugurated offer a powerful visual record of a significant moment in American history. The images capture not only the spectacle of the day but also the political and social context surrounding it. This analysis, by examining the inauguration through diverse lenses, highlights the enduring power of visual documentation in shaping our understanding of pivotal events.