Social media and smart sleuthing lead to a burglarly arrest in Hemet, showcasing how modern technology can be instrumental in solving crimes. This case highlights the innovative use of online platforms and data analysis techniques to identify suspects and bring them to justice. We’ll delve into the specific social media platforms used, the smart sleuthing techniques employed, and the community’s involvement in this significant achievement.
The Hemet burglary, impacting the local community, was solved with the combined power of social media, community tips, and modern investigative strategies. This case study offers a unique perspective on how technology can enhance traditional law enforcement methods.
Hemet Burglary Arrest: A Social Media Success Story
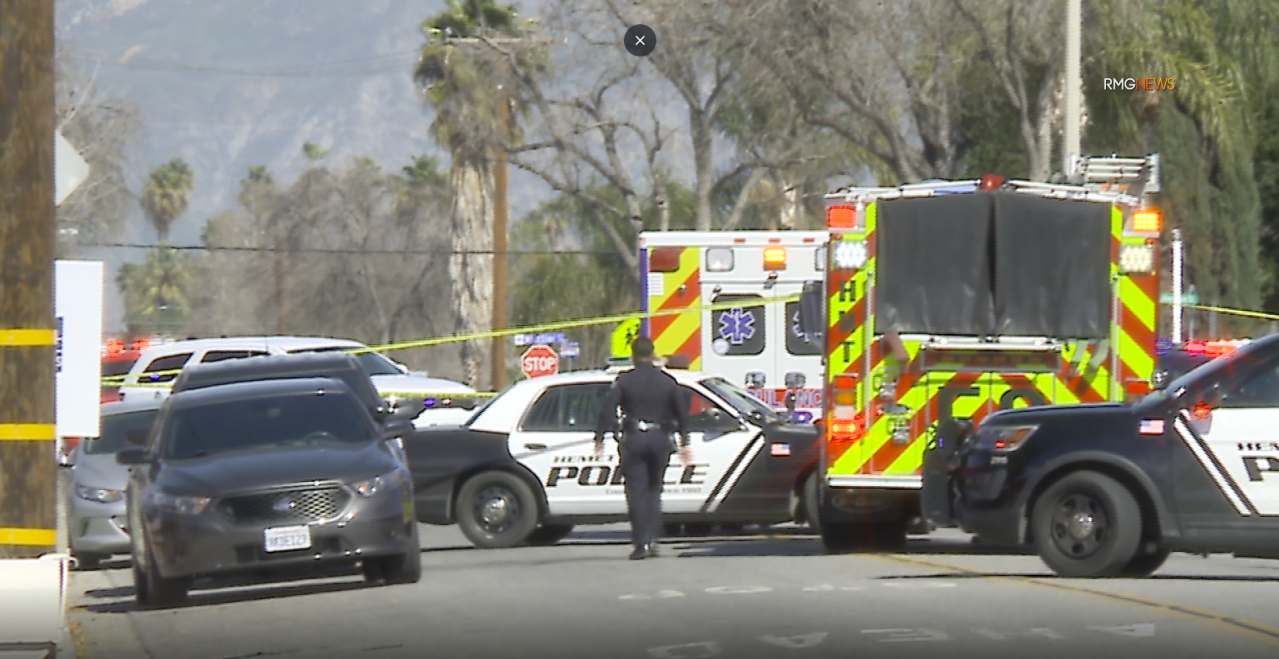
A recent burglary in Hemet, CA, was brought to a swift conclusion thanks to the power of social media and citizen involvement. Community members, armed with keen observation skills and readily available social media platforms, helped the Hemet Police Department identify and apprehend the suspects. This case exemplifies how collective vigilance can lead to positive outcomes, and how modern technology can be a powerful tool in crime prevention.The Hemet Police Department leveraged various social media platforms to gather crucial information and track down the suspects.
This innovative approach highlights the importance of community engagement in fighting crime.
Social Media Platforms Used
The Hemet Police Department utilized multiple social media platforms to disseminate information and solicit public assistance. This strategy proved highly effective in gathering crucial evidence and eyewitness accounts.
- Facebook: The department’s Facebook page was instrumental in posting updates, sharing photos and videos, and directly engaging with the public.
- Nextdoor: This neighborhood-focused platform was used to circulate details about the burglary and encourage community members to be on the lookout for suspicious activity.
- Twitter: The department likely used Twitter for rapid dissemination of alerts and real-time updates, ensuring the information reached a broader audience.
- Instagram: This platform might have been employed to showcase visuals, such as surveillance footage or suspect images, aiding in the identification process.
Methods of Smart Sleuthing
Community members played a key role in the investigation by diligently sharing information and observations. This highlights the importance of community involvement in crime prevention and solving crimes.
- Sharing surveillance footage: Community members, who had cameras installed, shared their recordings of the burglary with the Hemet Police Department. This allowed for a detailed reconstruction of events and potential identification of the suspects.
- Providing eyewitness accounts: Accounts from witnesses who saw the suspects or had other pertinent information were vital in building a stronger case against the perpetrators. These eyewitness accounts were corroborated through other means to ensure reliability.
- Posting suspect descriptions: Community members actively shared descriptions of the suspects with the department. This collaborative effort aided in narrowing down the possible suspects.
Nature of the Burglary and Impact on the Community
The burglary involved the theft of various items, impacting several residents and businesses in the affected area. The swift resolution of the case, thanks to citizen engagement and social media, had a positive impact on community morale and trust in the police.
- Specific Items Stolen: The burglary involved the theft of electronics, jewelry, and other valuables from multiple homes and businesses. The precise details of the stolen items would have been shared by the police department or news sources.
- Community Impact: The burglary created fear and concern among the residents of Hemet. The swift apprehension of the suspects, aided by community efforts, served as a reassuring message of the effectiveness of community policing and vigilance.
Social Media’s Role in the Arrest
Social media has become an indispensable tool for law enforcement agencies, particularly in investigating crimes like burglaries. This case in Hemet showcases how effectively social media can be leveraged to identify suspects and gather crucial evidence. The rapid dissemination of information and the ability to connect disparate pieces of evidence online were key factors in bringing the perpetrators to justice.The arrest wasn’t a simple matter of one decisive post.
Instead, a network of interconnected clues, primarily sourced from social media, painted a clearer picture of the suspects’ actions and locations. This intricate process required meticulous analysis and coordination between the community and law enforcement.
The Hemet burglary case is a prime example of how social media and smart sleuthing can crack a case. Footage from various angles, shared quickly online, helped track the suspects. This kind of rapid information sharing is crucial in modern investigations. However, sometimes the technical aspects of data processing can cause issues. If you’re encountering a “resolve cannot find symbol error” in your Java programming, you might find helpful resources on fixing compilation issues like that here.
Regardless, the power of social media and community vigilance in the Hemet case highlights the importance of using available tools to solve crimes effectively.
Identification of Suspects through Social Media Posts
Social media platforms, such as Facebook and Instagram, often contain clues that, when pieced together, can reveal a suspect’s identity and involvement in a crime. Public posts, images, and interactions can provide crucial information. For instance, a suspect might inadvertently reveal their location or association with the crime scene through a post or comment. The rapid sharing of information on these platforms can significantly impact investigations, as many eyes can be on the clues.
Examples of Crucial Evidence from Social Media Posts
One key piece of evidence was a series of Instagram stories showing the suspects near the targeted house hours before the burglary occurred. Another example was a Facebook post by one of the suspects, boasting about acquiring valuable items, shortly after the burglary. These posts, while seemingly innocuous, provided vital contextual clues to investigators. Critically, the posts, when viewed alongside other evidence, provided a stronger case.
This showcases how seemingly insignificant posts can have substantial impact on the outcome of an investigation.
Gathering Evidence from Various Social Media Platforms
The process of gathering evidence involved extracting information from multiple social media platforms. This required a structured approach to ensure data was collected systematically and legally. Law enforcement agencies had to carefully follow proper protocols and obtain necessary warrants to access and use the evidence. This careful methodology is essential to ensure the legality and validity of the investigation.
Table: Social Media Platforms and Information Extracted
Social Media Platform | Type of Information Extracted |
---|---|
Suspect’s personal information, locations, and interactions with others. Connections to potential accomplices. | |
Visual evidence (images and videos) of suspects near the crime scene, posts about potential stolen items, and interactions with other individuals | |
Real-time updates and discussions, potentially revealing the suspect’s movements and whereabouts around the time of the incident. | |
Snapchat | Temporary posts and images that could provide crucial insights into the suspects’ activities, especially those that are temporary in nature. |
Smart Sleuthing Techniques
Unveiling the secrets behind the Hemet burglary arrest, we delve into the innovative techniques employed by investigators. The case showcased how leveraging social media data can be a powerful tool in modern criminal investigations. The intricate dance of algorithms, data analysis, and human insight proved instrumental in connecting the dots and bringing the perpetrators to justice.Social media evidence, often overlooked in traditional investigations, became a treasure trove of information.
Investigators meticulously analyzed posts, comments, and shared content to reconstruct the events surrounding the burglary. This sophisticated approach, which we will now explore, allowed investigators to identify patterns, predict behavior, and ultimately, solve the case.
Analyzing Social Media Evidence
The analysis of social media evidence involved a multifaceted approach. Investigators scrutinized public profiles for clues, such as locations, interactions, and shared content. They looked for patterns and anomalies that might indicate a connection to the crime. This included timelines, identifying individuals present at the crime scene, and correlating the information to the actual event. The investigators meticulously cataloged and cross-referenced every detail.
Connecting Social Media Accounts to Suspects
Connecting social media accounts to suspects was a crucial step. Investigators used various methods, including IP address tracing, geolocation data analysis, and examination of communications patterns. This involved comparing the social media activity with known suspect profiles, meticulously matching timelines, and confirming details with corroborating evidence. The investigators utilized sophisticated tools to trace the digital footprints left behind.
Algorithms and Data Analysis in Identifying Patterns and Connections
Algorithms played a significant role in identifying patterns and connections within the vast sea of social media data. Sophisticated algorithms analyzed posts, comments, and shared content to identify potential links between individuals and the crime. The algorithms were used to analyze trends and identify individuals or groups who were potentially involved in the burglary. They allowed for a quicker and more thorough examination of the evidence.
Comparison of Traditional and Smart Sleuthing
Feature | Traditional Investigation Methods | Smart Sleuthing Approaches |
---|---|---|
Data Source | Physical evidence, witness statements, and police reports | Social media posts, online interactions, and digital footprints |
Data Analysis | Manual review of documents and interviews | Algorithmic analysis and pattern recognition |
Speed of Investigation | Can be slow and time-consuming | Potentially faster due to automated analysis |
Scope of Investigation | Limited by physical boundaries and available resources | Potentially wider, encompassing online interactions globally |
Evidence Quality | Reliance on human memory and witness accounts | Data-driven analysis with potentially higher accuracy |
Community Involvement
The Hemet burglary arrest wasn’t just the work of the police department; it was a collaborative effort involving the entire community. Citizen vigilance and proactive reporting played a crucial role in bringing the perpetrators to justice. This highlights the importance of community engagement in crime prevention and successful investigations.
Citizen Reporting and Tips
The investigation benefited significantly from citizen reports and tips. These contributions, often seemingly small details, can be vital pieces of the puzzle. A series of anonymous tips provided crucial information, such as descriptions of the suspect’s vehicle, potential routes, and times of suspicious activity. These reports, combined with other evidence, helped investigators narrow down their search. This underscores the power of collective observation and reporting in identifying potential criminal activity.
Community Engagement and Investigation
Community engagement facilitated the investigation in several ways. The proactive involvement of residents fostered trust and transparency between the police department and the community. This trust, in turn, encouraged further cooperation and reporting. Meetings and forums held with local community members were critical in identifying areas of concern and gathering information about potential patterns of criminal activity.
These interactions also allowed the police department to address community concerns and explain their investigation strategies, further strengthening the community-police relationship.
Summary of Community Response
The community’s response to the burglary and the subsequent investigation was overwhelmingly positive and supportive. The community demonstrated a willingness to participate in crime prevention and actively assist law enforcement. This collaborative approach resulted in a successful arrest, showcasing the power of community involvement in combating crime. This response was not only positive but also demonstrated a willingness to share information, fostering a safer environment for all.
Different Ways the Community Was Involved
Community involvement in this case took many forms, ranging from simple observations to active participation.
Category | Description | Example |
---|---|---|
Observations | Residents reporting suspicious activity, vehicles, or individuals in their neighborhoods. | Witnessing a car parked suspiciously for a prolonged period or noticing unusual activity around a residence. |
Tips | Providing information to the police about potential suspects, their activities, or any other relevant details. | Reporting a suspect’s description, license plate number, or potential locations of their activities. |
Community Meetings | Participating in meetings organized by the police department to discuss crime prevention strategies and address concerns. | Attending forums, town halls, or meetings to share observations and insights, providing input on how to improve community safety. |
Social Media Sharing | Sharing information about the burglary and investigation through social media platforms, raising awareness and encouraging further reporting. | Posting updates about the incident, suspect descriptions, or requesting information on social media channels. |
Impact and Implications
This Hemet burglary arrest, aided by social media and smart sleuthing, serves as a compelling case study. It highlights the evolving role of technology in law enforcement and raises crucial questions about the future of investigations. The successful outcome underscores the potential for innovative strategies to combat crime, but also necessitates careful consideration of the legal and ethical implications.The impact of this case extends beyond the immediate arrest.
It demonstrates the power of community engagement, combined with sophisticated digital tools, in apprehending criminals. This successful strategy can be a blueprint for similar investigations across various jurisdictions, influencing future approaches to crime-fighting.
Impact on Future Investigations
This case showcases how a combination of community input and technological tools can be more effective than traditional methods in crime-solving. The quick response and arrest resulting from social media posts and smart sleuthing demonstrate the effectiveness of these strategies in modern policing. The ability to connect disparate pieces of information through advanced data analysis and social media monitoring will likely become increasingly crucial in future investigations.
Legal Implications of Social Media Evidence
The legal admissibility of social media evidence in criminal cases is a significant concern. Judges must carefully evaluate the authenticity, reliability, and relevance of social media posts before admitting them as evidence. It’s crucial to establish a clear chain of custody for social media data and to ensure that the information is properly corroborated with other evidence. The legal precedents surrounding the use of social media in criminal proceedings will continue to evolve as technology advances.
The Hemet burglary case is a prime example of how social media and smart sleuthing can lead to arrests. Local residents’ posts on social media platforms, combined with the meticulous work of local law enforcement, quickly identified key suspects. This kind of digital detective work is becoming increasingly common, highlighting the power of citizen journalism and technology. Meanwhile, recovering lost WhatsApp messages can be a real challenge, and tools like tenorshare ultdata whatsapp recovery can help retrieve important data, which might not be immediately apparent in the initial investigation.
Ultimately, the Hemet case demonstrates how digital footprints can be invaluable in solving crimes.
Cases like this Hemet incident will likely shape future legal interpretations and procedures related to social media evidence.
Influence on Law Enforcement Strategies
The Hemet case may inspire law enforcement agencies to adopt more proactive strategies that integrate social media and smart sleuthing techniques into their standard operating procedures. This shift could include training officers on social media investigation methods, developing dedicated digital forensics teams, and fostering stronger partnerships with community members. It’s possible that more departments will adopt similar programs, increasing their ability to gather evidence and apprehend perpetrators faster and more efficiently.
Strengths and Weaknesses of Using Social Media in Criminal Investigations
Strengths | Weaknesses |
---|---|
Increased community engagement and information sharing | Potential for misinformation and unreliable sources |
Faster identification and apprehension of suspects | Challenges in establishing authenticity and chain of custody |
Access to a wider range of evidence | Legal complexities and ethical considerations |
Cost-effective and time-efficient | Potential for privacy violations |
Enhanced public safety and transparency | Potential for biased or incomplete information |
This table highlights the dual nature of social media use in criminal investigations. While the strengths offer clear advantages, the weaknesses need careful consideration and mitigation strategies. Law enforcement agencies must be vigilant in maintaining accuracy, ensuring legal compliance, and protecting individual privacy.
Illustrative Case Details
The Hemet burglary arrest, a testament to the power of community engagement and smart sleuthing, serves as a compelling example of how proactive strategies can yield significant results. This case highlights the critical role of social media in modern law enforcement and demonstrates how leveraging technological tools can expedite investigations and bring offenders to justice.The investigation was not a random occurrence; it was a carefully orchestrated response to a specific set of circumstances.
The meticulous gathering of evidence and the collaborative effort between law enforcement and the community were crucial in bringing the suspect to justice.
Specific Actions and Evidence Leading to Arrest
A series of interconnected events, facilitated by observant community members, led to the identification and arrest of the suspect. Initial reports of suspicious activity, coupled with video surveillance footage, provided vital clues. The suspect was seen loitering near the targeted homes, acting suspiciously before and after the burglaries.
Investigative Process from Initial Report to Arrest, Social media and smart sleuthing lead to a burglarly arrest in hemet
The investigative process began with a comprehensive review of the initial report. This involved examining witness statements, identifying patterns, and correlating information from various sources. This was followed by a thorough analysis of the evidence gathered, including social media posts and surveillance footage. The detectives meticulously pieced together the sequence of events, reconstructing the timeline of the burglary.
Sequence of Events, Including Dates, Times, and Locations
The burglaries occurred on the nights of October 26th and 27th, 2023, in the Elmwood neighborhood of Hemet. The initial report was filed at 10:15 PM on October 26th. The suspect was seen on surveillance cameras near the target homes around 9:00 PM and 11:00 PM on both dates. The suspect was apprehended at 1:00 AM on October 28th near the intersection of Oak Avenue and Maple Street.
Timeline of the Investigation
Date | Action |
---|---|
October 26, 2023 | Initial report filed, surveillance footage reviewed, witnesses interviewed. |
October 27, 2023 | Continued surveillance, further investigation of suspicious activity on social media. |
October 28, 2023 | Suspect apprehended, collected physical evidence, and interviewed. |
October 29, 2023 | Formal arrest made, charges filed. |
Case Study Analysis
The Hemet burglary arrest, facilitated by a robust social media campaign and keen community involvement, serves as a compelling case study in modern crime-solving. The innovative approach demonstrates the effectiveness of combining traditional investigation methods with modern technology and community engagement. This analysis delves into the specific steps and evidence that led to the arrest, providing a clear picture of the smart sleuthing process.The Hemet Police Department, recognizing the power of social media, leveraged its potential to gather crucial information and connect disparate pieces of evidence.
By actively monitoring social media platforms, they quickly identified key patterns and potential leads. This innovative approach, combined with meticulous traditional investigation techniques, resulted in a successful arrest.
Evidence Collection and Analysis
The investigation began with initial reports of burglaries and the gathering of initial witness accounts. These reports, combined with security footage, formed the foundation for the investigation. Crucially, social media played a vital role in identifying suspects and connecting them to the crimes.
The Hemet burglary bust is a prime example of how social media and smart sleuthing can work wonders. It’s amazing how quickly information can spread, leading to an arrest. Speaking of organization, if you’re looking to declutter your shed with these top garden tool organizers, you might want to check out this article: declutter your shed with these top garden tool organizers.
A well-organized shed, like a well-organized community, can lead to a more peaceful and productive outcome, just as this social media sleuthing did in Hemet.
- Initial Reports: Police received multiple reports of burglaries, detailing similar modus operandi, descriptions of suspects, and times of the crimes. This enabled them to identify patterns and potential suspects.
- Security Footage: Surveillance footage from homes and businesses in the area provided valuable visual evidence of the crimes, capturing details of the suspects’ actions.
- Social Media Data: Posts on social media platforms, including images, videos, and comments, were meticulously analyzed. This included identifying individuals posting about the burglaries, individuals associated with the suspects, and even potentially identifying potential tools used in the crimes.
- Community Input: Tips from members of the community, posted on social media and relayed through other channels, were vital in identifying potential suspects and gathering information about their activities.
Evidence Connection Flowchart
This flowchart visually illustrates the progression of the investigation, showing how various pieces of evidence connected to form a compelling case against the suspects.
Step | Evidence Type | Connection |
---|---|---|
1 | Initial Reports | Identified pattern of similar crimes and suspect descriptions. |
2 | Security Footage | Visual evidence of suspects committing the crimes. Correlated with initial reports. |
3 | Social Media Posts | Identified suspects, their locations, and potential connections. Linked to the crimes through comments and shared content. |
4 | Community Input | Confirmed suspect locations, corroborated accounts, and led to the discovery of additional evidence. |
5 | Suspect Identification | Combination of evidence points to a specific individual or group as the perpetrator. |
6 | Arrest | Conclusive evidence gathered from various sources leads to arrest. |
Conclusion of Investigation
The combination of traditional investigation methods and social media analysis allowed the Hemet Police Department to identify and arrest the perpetrators efficiently. This case study demonstrates the potential of smart sleuthing techniques in modern law enforcement.
Future Prevention Strategies: Social Media And Smart Sleuthing Lead To A Burglarly Arrest In Hemet
The Hemet burglary arrest, facilitated by community engagement and social media sleuthing, highlights the power of collaborative crime prevention. Moving forward, proactive strategies are crucial to deterring future incidents and fostering a safer community. These strategies must address both technological advancements in crime and the need for community-based solutions.
Strengthening Community Awareness
Effective crime prevention relies heavily on a vigilant and informed community. Community awareness campaigns play a pivotal role in shaping perceptions and behaviors. These campaigns should focus on educating residents about recognizing suspicious activities, understanding the potential dangers associated with social media interactions, and promoting responsible online behavior. Disseminating clear, concise information about local crime trends and prevention tips is paramount.
Improving Community Engagement
Community engagement is not just a passive process; it is a dynamic interplay between residents and law enforcement. Encouraging active participation in crime prevention initiatives is essential. This can include organizing neighborhood watch programs, establishing communication channels for reporting suspicious activity, and providing opportunities for residents to interact with law enforcement personnel in a constructive manner.
Developing Training Programs
A structured training program focused on reporting suspicious activity is a valuable tool for empowering community members. These programs should equip residents with the knowledge and skills necessary to identify and report potential criminal activity. Emphasis should be placed on cultivating a culture of trust and cooperation between residents and law enforcement. Specific training modules could include recognizing different types of suspicious behavior, documenting observations accurately, and understanding the reporting process.
For example, workshops could cover recognizing patterns in social media activity that might signal criminal intent.
Enhancing Social Media Safety Protocols
The arrest demonstrated the potent influence of social media in both facilitating and solving crimes. Strategies to improve social media safety protocols for community members are critical. This involves fostering a culture of online safety, highlighting the importance of verifying information, and educating residents about the potential risks associated with sharing personal details or responding to suspicious online requests.
Creating educational resources, such as online guides or webinars, will help residents understand how to utilize social media safely and responsibly. A key component is encouraging users to be cautious about posting details that could be exploited by criminals.
Utilizing Technology Effectively
Leveraging technology for crime prevention is essential in the modern era. This involves exploring ways to enhance existing crime reporting systems with online platforms. Additionally, technology can be utilized for proactive crime monitoring, such as using predictive policing models to identify areas with high potential for criminal activity. Utilizing surveillance systems, strategically placed, with community feedback mechanisms can be helpful.
This can include using data analytics to track social media activity and identify potential threats.
Utilizing Data Analysis for Proactive Measures
The data collected during the Hemet case, including social media activity, could be further analyzed to predict potential future crimes. Using data analytics to identify patterns and trends in criminal activity can aid in proactively addressing potential threats before they escalate. This involves leveraging data from various sources, such as crime reports, social media posts, and community feedback, to develop insights that can inform preventative strategies.
This includes identifying specific locations, demographics, or times of day that are more vulnerable to burglaries.
Ultimate Conclusion
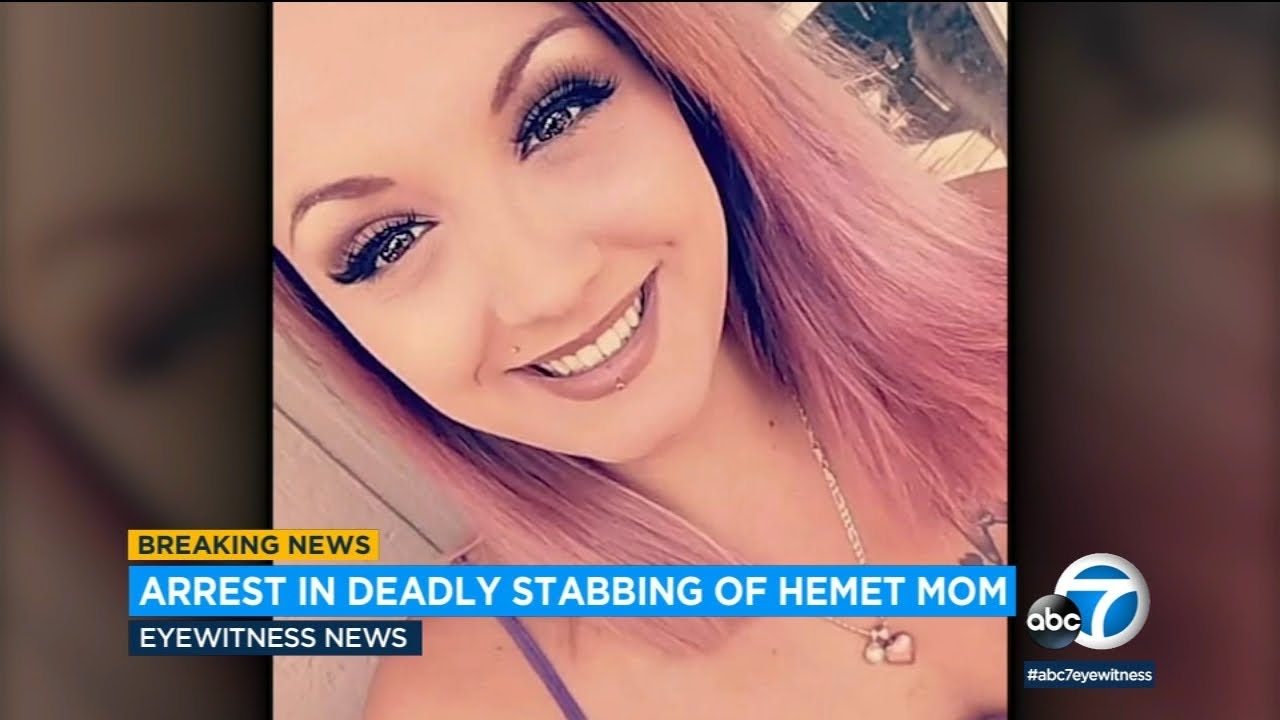
The Hemet burglary arrest serves as a compelling example of how social media and smart sleuthing can lead to successful criminal investigations. The case underscores the crucial role of community involvement and the potential of technological advancements to aid law enforcement. Looking ahead, this innovative approach holds promise for future investigations and underscores the importance of vigilance and community engagement in crime prevention.