Berryessa flea market san jose singleton landfill – Berryessa Flea Market, San Jose’s Singleton Landfill, a bustling marketplace near a significant waste disposal site, presents a complex interplay of economic activity and environmental concerns. This market, a cornerstone of the local community, draws a diverse clientele while existing in close proximity to a landfill. Understanding its history, impact on the environment, and the community’s perspective is crucial for a complete picture of this unique location.
The market’s evolution, from its humble beginnings to its current state, is a testament to community resilience. This examination delves into the vendors’ offerings, the market’s accessibility, and the demographics of its patrons. A detailed look at the market’s environmental footprint, including potential pollution sources and mitigation efforts, is also presented. Ultimately, this discussion explores the market’s economic contribution, its role in fostering community engagement, and the potential conflicts arising from its location.
Berryessa Flea Market: A San Jose Community Hub
The Berryessa Flea Market, nestled in the heart of San Jose, is more than just a collection of stalls; it’s a vibrant tapestry woven from the threads of local commerce, community spirit, and unique finds. This market has evolved from a humble gathering to a significant fixture in the region, reflecting the changing needs and desires of its patrons.
Its proximity to the Singleton Landfill, while sometimes a source of concern, also plays a role in shaping the market’s character.This market offers a diverse array of goods and services, catering to a wide range of tastes and budgets. Understanding the market’s history, vendor types, and customer base provides a richer appreciation for its role within the San Jose community.
The evolution of the market, the products it showcases, and its impact on the local economy are all integral parts of its story.
Market History and Significance
The Berryessa Flea Market’s origins can be traced back to [Insert Year] when a group of local entrepreneurs sought to create a space for local artisans and vendors to connect with potential customers. Initially a small gathering, the market gradually gained traction and popularity, attracting more vendors and customers. Over time, it has adapted to evolving community needs, expanding its offerings and establishing a loyal customer base.
The market’s resilience and enduring presence speak to its importance as a community hub.
Vendor Types and Products
The market is characterized by a diverse array of vendors, each offering unique goods. These vendors represent a variety of economic activities, from individual artisans to established businesses. Understanding the diverse offerings is key to appreciating the market’s role as a one-stop shop for a wide range of goods.
- Artisans: These vendors frequently display handmade crafts, paintings, jewelry, and clothing, often reflecting a unique artistic vision. Their creations often showcase intricate details and personalized touches, making them desirable to collectors and individuals seeking distinctive items.
- Resellers: This group often features pre-owned items, such as clothing, furniture, and collectibles. Their offerings reflect the secondhand economy and allow customers to find unique and affordable items.
- Food Vendors: A notable aspect of the market is the selection of food stalls, offering a variety of cuisines from local restaurants and food trucks. These food stalls add to the market’s vibrancy and cater to the diverse tastes of the community.
- Antique Dealers: These vendors specialize in vintage and antique items, offering a glimpse into the past. They often feature unique pieces with historical significance, appealing to collectors and enthusiasts of vintage items.
Market Location and Accessibility
The Berryessa Flea Market is conveniently located near the Singleton Landfill. While the proximity to the landfill may raise concerns about environmental impact, it also has practical benefits for accessibility and logistics. The market’s strategic location facilitates ease of access for both vendors and customers, attracting a diverse customer base.
Customer Demographics
The customer base of the Berryessa Flea Market is a blend of various age groups and backgrounds. It’s a place where families, young adults, and seniors alike come together to discover unique treasures, enjoy the atmosphere, and support local businesses.
Vendor and Offerings Table
Vendor Type | Typical Offerings |
---|---|
Artisans | Handmade crafts, paintings, jewelry, clothing |
Resellers | Pre-owned clothing, furniture, collectibles |
Food Vendors | Local cuisines, food trucks |
Antique Dealers | Vintage and antique items |
Environmental Impact: Berryessa Flea Market San Jose Singleton Landfill
The Berryessa Flea Market, nestled near the Singleton Landfill, presents a unique set of environmental challenges. Understanding these concerns is crucial for ensuring the market’s sustainability and minimizing its impact on the surrounding community and environment. The proximity to a significant waste disposal site introduces potential pollution pathways and necessitates careful consideration of operational practices and regulatory compliance.The proximity of the flea market to the Singleton Landfill necessitates careful environmental planning.
Potential sources of pollution, ranging from air and water contamination to the spread of harmful waste, need to be addressed. Effective management and regulation are paramount to mitigating risks and ensuring the market operates responsibly within its environmental context. This assessment will explore potential environmental risks, current regulations, and the market’s footprint compared to similar operations.
Potential Pollution Sources and Risks
The flea market, with its diverse vendors and potentially improper waste disposal practices, poses various environmental risks. Potential sources of air pollution include exhaust fumes from vehicles, dust from construction and handling of goods, and emissions from poorly maintained equipment. Runoff from improperly managed wastewater or spills could contaminate nearby water sources. Furthermore, the handling and storage of hazardous materials, such as batteries or paints, pose significant risks if not properly regulated.
Improper disposal of these materials can lead to leaching into the soil and groundwater, contaminating the surrounding environment.
Environmental Footprint Comparison
Comparing the Berryessa Flea Market to similar markets in the area provides valuable insights into its potential environmental impact. Detailed analysis of similar markets’ waste management practices, energy consumption, and water usage can be used to identify best practices and areas for improvement. Metrics such as waste generation per vendor, energy consumption per unit of sale, and water usage per transaction can be used for comparison.
Data from these assessments will provide benchmarks for evaluating the Berryessa Flea Market’s environmental footprint. Without specific data on similar markets, generalizations about the Berryessa Flea Market’s footprint are limited.
Regulations and Policies for Mitigation
Several regulations and policies are in place to mitigate environmental risks associated with the market and the landfill. These regulations often focus on waste management, air quality, and water pollution prevention. Local and state environmental agencies play a critical role in enforcing these regulations and ensuring compliance. Compliance with these regulations is vital for the market’s continued operation.
Summary of Environmental Regulations
Regulation Category | Description | Impact on Berryessa Flea Market |
---|---|---|
Waste Management | Regulations governing waste segregation, disposal, and recycling | Vendors must adhere to proper waste disposal procedures to prevent contamination of the landfill and the surrounding environment. |
Air Quality | Standards for emissions from vehicles, equipment, and activities | The market should implement measures to control emissions from vehicles and equipment to maintain air quality standards. |
Water Quality | Regulations on wastewater discharge and spill prevention | Procedures for managing wastewater and preventing spills must be strictly adhered to. |
Hazardous Materials Handling | Rules for the storage, handling, and disposal of hazardous materials | Vendors dealing with hazardous materials must adhere to strict guidelines for handling and disposal. |
Community and Economic Impact
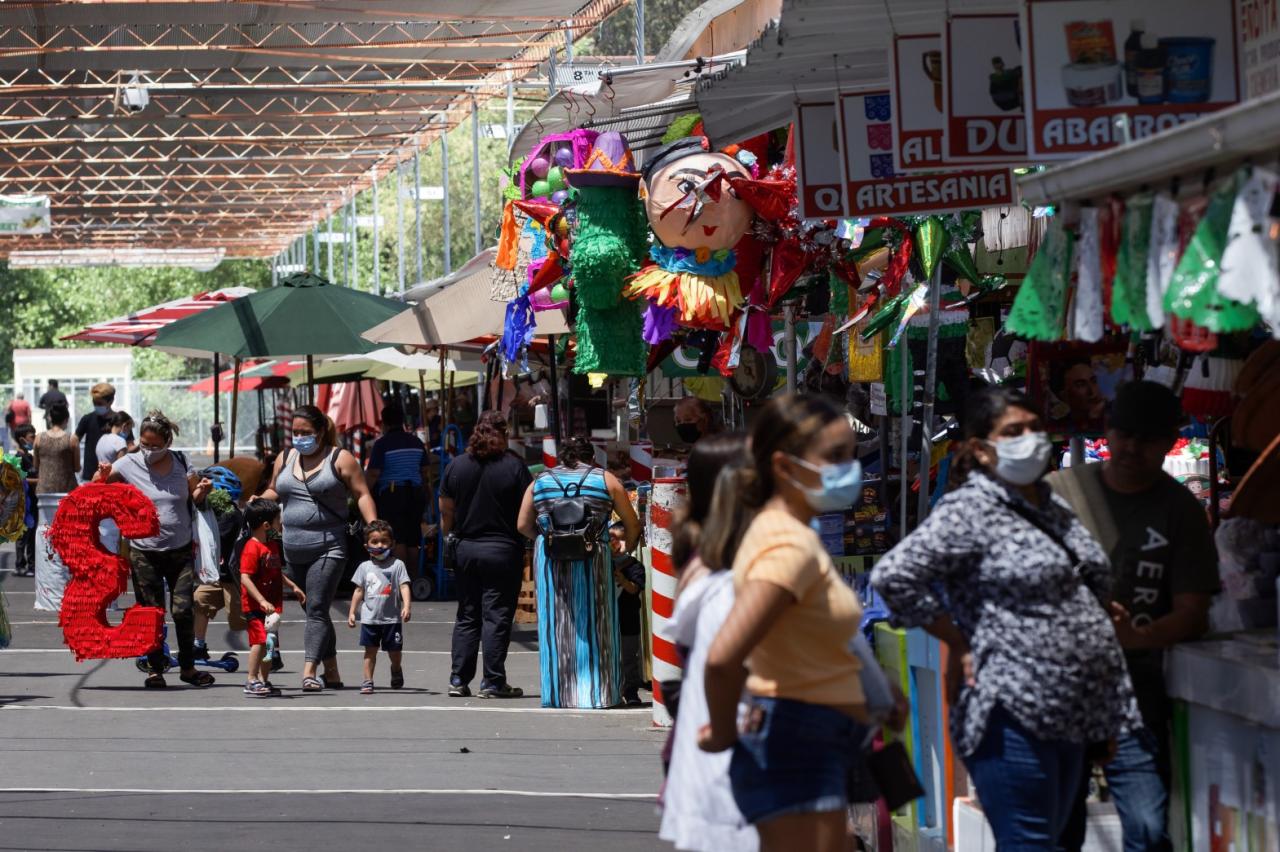
The Berryessa Flea Market, nestled in the heart of San Jose, isn’t just a place to browse; it’s a vital economic and social hub. This vibrant gathering spot offers a unique opportunity to explore the community’s creativity and entrepreneurship, fostering a sense of connection and shared experience. Its economic contributions extend beyond individual transactions, influencing local businesses and job markets.This analysis explores the various economic facets of the market, examining its role in creating employment opportunities, supporting local businesses, fostering community engagement, and providing a platform for local artisans and entrepreneurs.
Ever been to the Berryessa Flea Market in San Jose, near the Singleton Landfill? It’s a fascinating glimpse into local culture, but did you know that the same energy of entrepreneurial spirit might also be impacting broader political landscapes? Recent reports on states trump second term plans suggest a potential shift in priorities, which could ultimately influence future policy regarding local markets and waste management like the landfill.
All of this makes the flea market’s hustle and bustle all the more interesting to consider, right?
The market’s positive impact on the community is multifaceted, and this exploration highlights its significance.
Economic Contributions to the San Jose Community
The Berryessa Flea Market provides a platform for a wide array of vendors, from established local businesses to independent crafters and food trucks. This diversity directly impacts the local economy, generating income and supporting local businesses. Many vendors rely on the market as their primary source of income, and this, in turn, fuels the overall economic vitality of the San Jose area.
Jobs Created by the Market
The market’s operation creates numerous jobs, including vendor positions, support staff for setup and management, and potentially positions for local security and maintenance. These jobs contribute to the overall employment landscape of the community, providing a crucial lifeline for those seeking work. For instance, the increased demand for services like transportation and food preparation could lead to new opportunities in these related sectors.
Impact on Local Businesses
The flea market serves as a valuable promotional platform for local businesses. Vendors can showcase their products and services to a wider audience, leading to increased sales and customer engagement. The presence of the market might also attract more foot traffic to surrounding shops and restaurants, further benefiting the local business community. This can lead to a symbiotic relationship where the market and local businesses support each other.
Role in Fostering Community Engagement and Interaction
The market fosters community engagement by providing a common space for residents to interact, discover local talent, and support their neighbours. It becomes a social gathering point, creating opportunities for community members to connect with each other and experience a sense of belonging. These interactions can strengthen community bonds and promote a sense of shared identity.
Supporting Local Artisans and Entrepreneurs
The market serves as a vital platform for local artisans and entrepreneurs to showcase their unique products and services. It provides a supportive environment for individuals to launch their businesses, experiment with new ideas, and receive valuable feedback from potential customers. The market can act as a springboard for creativity and innovation, giving a boost to local talent and enabling them to reach a wider customer base.
The Berryessa Flea Market, near the San Jose Singleton Landfill, is a treasure trove of vintage finds, but lately I’ve been distracted by the drama surrounding Eric Thomas’s in-laws, which is making me question everything about my own family. Seriously, checking out this article on the topic has left me pondering the surprising parallels between family squabbles and the hunt for that perfect antique vase at the flea market.
Maybe I’ll just stick to the vintage finds at Berryessa for now.
Economic Benefits and Drawbacks
Economic Benefit | Economic Drawback |
---|---|
Increased economic activity and employment | Potential for increased traffic congestion and parking issues in the area. |
Support for local businesses and entrepreneurs | Competition for resources and customers from existing local businesses. |
Enhanced community engagement and interaction | Potential for negative impact on existing neighborhoods if not managed properly. |
Exposure to a wider customer base for local products | Potential for oversaturation of the market with similar products. |
Improved local economy and revenue generation | Potential for environmental concerns if not managed sustainably. |
Potential Conflicts and Solutions
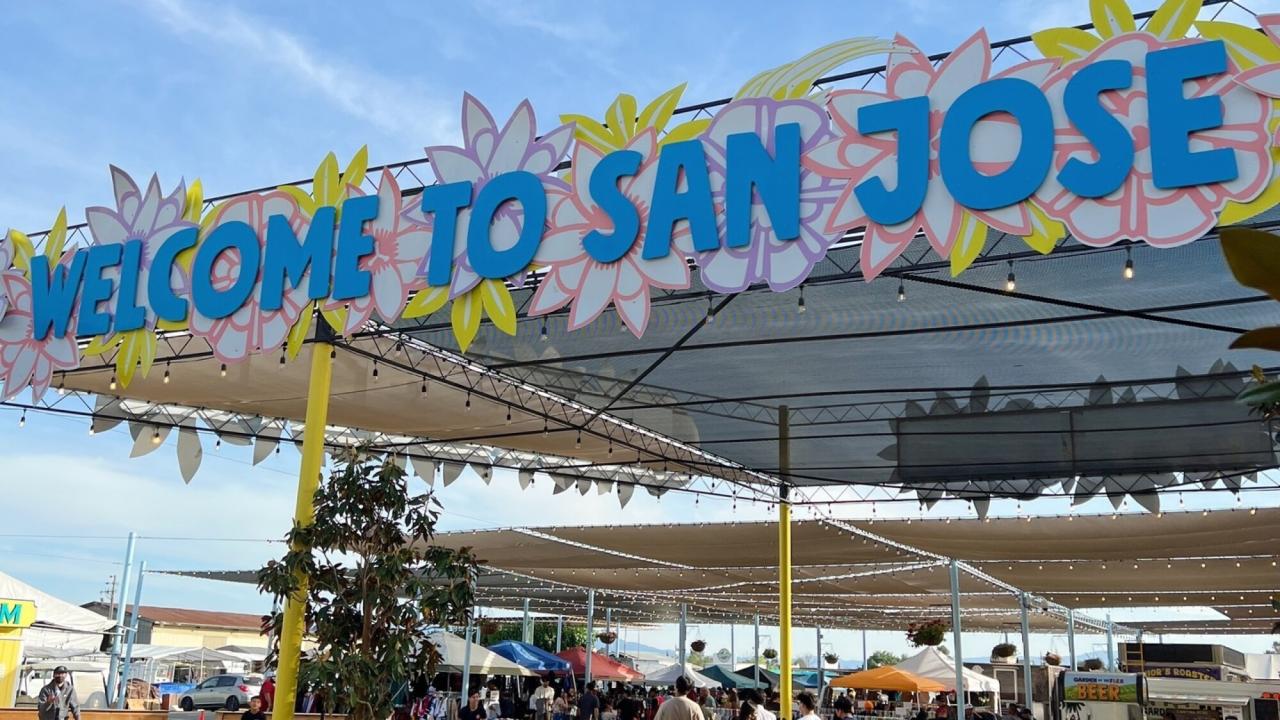
The Berryessa Flea Market, poised to become a vibrant community hub, presents unique challenges due to its proximity to the Singleton Landfill. Careful consideration of potential conflicts and proactive solutions is crucial for a successful and sustainable venture. This section explores potential friction points, Artikels strategies for mitigating negative impacts, and highlights the significance of collaborative efforts between market organizers, city officials, and community members.
Noise Pollution
Proximity to the landfill may result in noise disturbances from traffic, construction, or landfill operations. To mitigate this, market organizers can implement noise-reducing strategies, such as using quieter equipment and scheduling peak market activities during periods of reduced landfill operations. A designated quiet zone for sensitive activities, such as live music performances, should be considered. Monitoring noise levels and adjusting operations as needed is essential.
Traffic Congestion
Increased traffic volume around the flea market could lead to congestion, particularly during peak hours. Solutions include promoting alternative transportation options, like biking, walking, and ride-sharing services. Clearly marked traffic flow patterns and designated parking areas can help manage traffic effectively. Coordination with city traffic management can improve traffic flow and minimize congestion. Implementing a shuttle system to transport visitors from a nearby park-and-ride lot could reduce traffic burden.
Waste Management Challenges, Berryessa flea market san jose singleton landfill
Managing the waste generated by the flea market is a critical aspect of sustainability. A robust waste sorting and recycling program, including clear signage and designated bins for different waste streams, is essential. Educating vendors and market-goers on proper waste disposal techniques is crucial. Partnering with local recycling centers and implementing a system for composting organic waste can reduce landfill burden and promote environmental responsibility.
Providing incentives for vendors and visitors to actively participate in the waste management program can improve the effectiveness of the initiative.
Collaboration and Community Engagement
Successful management of the flea market necessitates strong collaboration between market organizers, city officials, and community members. Regular communication channels, public forums, and feedback mechanisms can foster a sense of shared responsibility. This collaborative approach will ensure that the flea market aligns with community values and expectations.
Speaking of San Jose’s unique offerings, the Berryessa Flea Market near the Singleton Landfill is always a fun adventure. While you’re browsing vintage treasures and quirky collectibles, it’s worth considering the larger housing picture in Marin County. They’re looking at a $5.2 million loan for a Habitat for Humanity housing project, which is a great step toward affordable housing solutions.
Hopefully, this kind of initiative will lead to better living conditions for those who frequent the Berryessa Flea Market and the surrounding areas. Still, the flea market is always a good time.
Table: Potential Solutions to Conflicts
Conflict | Potential Solution | Sustainability Focus | Community Engagement Strategy |
---|---|---|---|
Noise Pollution | Quiet zones, scheduling adjustments, noise-reducing equipment | Minimizing environmental impact | Public awareness campaigns, feedback mechanisms |
Traffic Congestion | Promoting alternative transportation, clear traffic flow, designated parking, shuttle system | Encouraging sustainable transportation | Collaboration with city traffic management, community surveys |
Waste Management | Robust waste sorting and recycling program, vendor and visitor education, composting | Reducing landfill burden, promoting circular economy | Incentives for participation, clear signage, educational materials |
Visual Representation
The Berryessa Flea Market, poised to become a vibrant hub in San Jose, faces a unique challenge: its proximity to the Singleton Landfill. Understanding the visual relationship between the two is crucial for assessing the potential impact of the market on the area and vice-versa. This section dives into the visual landscape of the area, from the landfill’s features to the sensory experience of the surrounding environment.The Singleton Landfill, a significant feature in the region, is a large expanse of land, its exact dimensions varying depending on the source of information.
Its visual presence is undeniable, impacting the surrounding landscape and influencing the visual relationship between the landfill and the planned flea market. The landfill’s topography is likely characterized by earth mounds and compacted waste, forming a distinctive landscape that contrasts with the proposed market’s planned layout and the natural features surrounding it.
Landfill Description
The Singleton Landfill, a significant feature of the Berryessa area, occupies a substantial portion of the landscape. Its size, while not precisely quantified, is substantial enough to be a noticeable element in the area’s visual composition. Its features include earth mounds, compacted waste piles, and potentially access roads. The landfill’s surroundings include a mixture of existing infrastructure, such as residential areas, roads, and possibly other industrial or commercial developments.
The immediate surroundings of the landfill likely consist of varying terrain and vegetation, ranging from bare land to more established natural elements.
Visual Relationship
The planned Berryessa Flea Market sits in close proximity to the Singleton Landfill. This close proximity directly affects the visual relationship between the two, creating a unique juxtaposition. The visual impact of the landfill on the market and vice-versa is a critical consideration in the planning process. The visual contrast between the market’s intended aesthetic (a vibrant community hub) and the landfill’s existing visual character (an industrial landscape) needs careful consideration.
Successful mitigation strategies will likely need to focus on visually softening the impact of the landfill on the market’s image.
Landscape Features
The landscape surrounding the Singleton Landfill and the proposed Berryessa Flea Market likely includes a variety of natural elements. These elements might range from trees, shrubs, and grasses to potentially more significant natural features like streams or hills. The presence of these natural elements could influence the overall visual impact of the area, offering opportunities for visual integration or creating necessary visual separation.
The density and types of these natural elements will play a key role in determining how the flea market can blend in with the existing environment.
Sensory Experience
The sensory experience of the area surrounding the Singleton Landfill and the proposed flea market is likely a blend of different sensations. The sights may range from the landfill’s landscape to the surrounding residential areas and natural elements. The sounds could encompass traffic noise, the sounds of nature, and potential sounds from the flea market. Smells might include those associated with the landfill, as well as aromas from the market’s food stalls.
“The visual experience of the location is a dynamic interplay between the existing industrial landscape of the landfill and the envisioned vibrant community hub of the flea market. The key is to find a balance between the visual impact of the landfill and the market’s intended positive aesthetic, through landscaping and other visual mitigation strategies.”
Final Wrap-Up
The Berryessa Flea Market, situated near the Singleton Landfill, presents a compelling case study of balancing economic growth with environmental responsibility. The market’s impact on the local economy, community engagement, and the environment is multifaceted and deserves careful consideration. Addressing potential conflicts, such as waste management and traffic concerns, requires collaboration between market organizers, city officials, and community members.
The market’s future hinges on finding sustainable solutions that benefit both the community and the environment.