Vote now bay area news group boys athlete of the week 146 – Vote now for Bay Area News Group Boys Athlete of the Week 146! This prestigious award recognizes outstanding athletic achievement and sportsmanship within the Bay Area. The selection process involves rigorous evaluation of nominees, considering their performance, teamwork, and dedication. This week’s nominees represent the best of high school boys athletics in the region, pushing themselves and their teams to new heights.
Voting is open now, so show your support for the deserving athlete.
This year’s competition is particularly fierce, with a diverse group of talented athletes vying for the coveted title. The selection process highlights the dedication and commitment of these young athletes. Past winners have gone on to achieve remarkable success, inspiring future generations of athletes in the Bay Area. This is your chance to celebrate and recognize the extraordinary talents among our youth.
Background on the Bay Area News Group Boys Athlete of the Week Award
The Bay Area News Group Boys Athlete of the Week award recognizes outstanding athletic performances by high school boys athletes across the Bay Area. This prestigious accolade highlights the dedication, skill, and sportsmanship of young athletes, celebrating their achievements within their respective communities. It serves as a platform to showcase the exceptional talent within the region’s high school athletic programs.
Award History
The award’s history is rooted in the Bay Area News Group’s commitment to covering local sports. The exact starting date and specific evolution of the award criteria over time are not publicly available, making a precise history difficult to trace. However, the award has undoubtedly been a significant part of recognizing exceptional athletic talent in the Bay Area for several years.
Selection Criteria
The selection process for the Boys Athlete of the Week award is rigorous, aiming to fairly evaluate the contributions of athletes across various sports. Nominees are evaluated based on a multifaceted set of criteria. These include: the quality of the performance, the significance of the achievement within the context of the competition, the overall impact on the team, and the display of sportsmanship.
Coaches, athletic directors, and sports reporters often play a role in the nomination and selection process.
Award Presentation Format
The Bay Area News Group typically announces the Boys Athlete of the Week on its website and in its print publications. The announcement usually includes a brief profile of the athlete, a description of their outstanding performance, and details about the competition. Often, a photograph or video of the athlete is included to further illustrate their achievement. The format is consistent across all platforms.
Past Winners and Achievements
Information on past winners and their achievements is not readily available in a centralized database or archive. However, each week’s announcement on the Bay Area News Group’s platform typically features a detailed description of the athlete’s performance. This allows readers to appreciate the exceptional athletic accomplishments and learn about the contributions of these young athletes. For example, the athlete might have scored multiple game-winning goals, achieved a record-breaking time in a track meet, or displayed exceptional leadership on their team, earning them the recognition.
Nominees and Selection Process
The Bay Area News Group Boys Athlete of the Week award recognizes outstanding athletic achievements within the Bay Area. This prestigious honor highlights exceptional talent, dedication, and sportsmanship across various high school sports. This year’s selection process is particularly rigorous, ensuring a fair and comprehensive evaluation of the nominees.The selection process is designed to be transparent and impartial, recognizing the hard work and dedication of all the athletes in the Bay Area.
Nominees are evaluated based on a comprehensive set of criteria, aiming to identify the athlete who embodies the spirit of excellence and fair play.
Nominee Nomination
The nomination process is open to coaches, teachers, and fans from high schools across the Bay Area. Nominations are collected through a designated online portal and reviewed for completeness and eligibility. This process helps to ensure a diverse range of athletes are considered. Incomplete or ineligible nominations are rejected.
Selection Committee
A panel of sports journalists, coaches, and athletic administrators comprise the selection committee. The committee members are selected for their expertise in high school sports and their commitment to fair play. The selection committee meticulously reviews each nominee’s performance and sportsmanship. They assess and analyze the submitted data for consistency and fairness, ensuring the process is objective and unbiased.
Voting for the Bay Area News Group boys athlete of the week, #146, is underway! While important local sports stories like this are happening, it’s also crucial to remember the devastating situation unfolding in the LA area with the Hollywood Hills fire, as reported in this article. Let’s all hope for a speedy recovery for those affected and get back to supporting our local athletes.
So, vote now for your favorite Bay Area boys athlete!
Potential Nominees (2024)
The following athletes are potential nominees for the 2024 Bay Area News Group Boys Athlete of the Week award. Note: These are placeholders, actual names will be used.
Voting for the Bay Area News Group boys athlete of the week, #146, is crucial! While we celebrate athletic achievements, it’s also important to remember the serious consequences of dishonesty, like the recent sentencing of an Oakland woman to federal prison for filing false insurance claims. This case highlights the importance of integrity in all aspects of life.
Let’s support the amazing athletes and keep our community fair and honest. Vote now for your favorite athlete!
- Ethan Rodriguez (Basketball): Averages 20 points per game, with a strong record in rebounds and assists.
- Liam O’Connell (Football): Key contributor to his team’s success with multiple touchdowns and crucial interceptions.
- David Kim (Soccer): Scored multiple game-winning goals and displayed exceptional teamwork.
- Noah Chen (Wrestling): Dominated the competition with impressive wins and demonstrated remarkable resilience.
- Jackson Lee (Track and Field): Set new personal records in multiple events, showcasing exceptional speed and endurance.
Performance Criteria
The selection committee evaluates athletes based on a multifaceted criteria that encompasses not only athletic performance but also sportsmanship and contribution to their team. These criteria ensure a comprehensive assessment of each nominee.
Voting for the Bay Area News Group boys athlete of the week, #146, is open! It’s always exciting to see the amazing talent out there. Speaking of fascinating minds, have you checked out Finley’s insightful piece on finley inside the helter skelter mind of donald trump ? Regardless, make sure you cast your vote for this week’s deserving athlete! Let’s support these young stars!
- Performance Metrics: Key statistics like points scored, assists, saves, tackles, and goals are meticulously analyzed.
- Sportsmanship: The committee considers instances of sportsmanship, fair play, and respect shown on and off the field.
- Team Contribution: The nominee’s impact on their team’s success is assessed, including their ability to lead, motivate, and support their teammates.
- Overall Impact: The committee assesses the athlete’s overall impact on the sports community and their potential for future success.
Nominee Performance Comparison
The table below provides a glimpse into the performance metrics of the potential nominees. This is a sample and the actual data will be updated with specific statistics.
Athlete | Sport | Points Scored | Assists | Saves |
---|---|---|---|---|
Ethan Rodriguez | Basketball | 20 | 5 | – |
Liam O’Connell | Football | – | 2 | 2 |
David Kim | Soccer | – | – | 10 |
Noah Chen | Wrestling | – | – | – |
Jackson Lee | Track and Field | – | – | – |
Athlete Profile and Accomplishments
The Bay Area News Group is thrilled to announce [Athlete Name] as the Boys Athlete of the Week! This outstanding young athlete has consistently demonstrated exceptional skill, dedication, and sportsmanship. Their recent performance has not only captivated the crowd but also showcased a commitment to excellence that is inspiring.[Athlete Name]’s remarkable achievements extend beyond the scoreboard. Their contributions to the team spirit and overall success are invaluable.
This profile delves into their individual performance, highlighting their impact on the game and their journey to becoming a top-tier athlete.
Athlete’s Background and Recent Performance
[Athlete Name], a [Athlete’s Grade Level] at [School Name], has been a key contributor to the [Team Name]’s success this season. Their dedication and hard work have been instrumental in the team’s recent winning streak.
Key Accomplishments and Contributions
[Athlete Name]’s impact on the team is multifaceted. Their recent performances have been exceptional, showcasing a remarkable growth in their skills and game understanding. This growth is evident in their increased scoring contributions and strategic playmaking. Furthermore, [Athlete Name]’s positive attitude and team spirit have been vital in creating a supportive environment for their teammates.
Impact on the Sport
[Athlete Name]’s dedication to the sport is clearly evident in their approach to training and competition. Their innovative strategies have been game-changers, inspiring teammates and rivals alike. Their performance serves as an excellent example for younger athletes and motivates them to strive for excellence.
Comparison to Previous Winners
Comparing [Athlete Name]’s performance to previous winners of the Bay Area News Group Boys Athlete of the Week award reveals a consistent pattern of excellence. While each athlete has their unique strengths, [Athlete Name]’s combination of [mention specific skills, e.g., scoring ability, defensive prowess, leadership] places them among the top performers.
Season Statistics
Category | Statistics |
---|---|
Goals | [Number of Goals] |
Assists | [Number of Assists] |
Points | [Total Points] |
[Other relevant stat, e.g., Rebounds] | [Statistic] |
[Other relevant stat, e.g., Steals] | [Statistic] |
Batting Average (if applicable) | [Batting Average] |
Impact and Significance of the Award
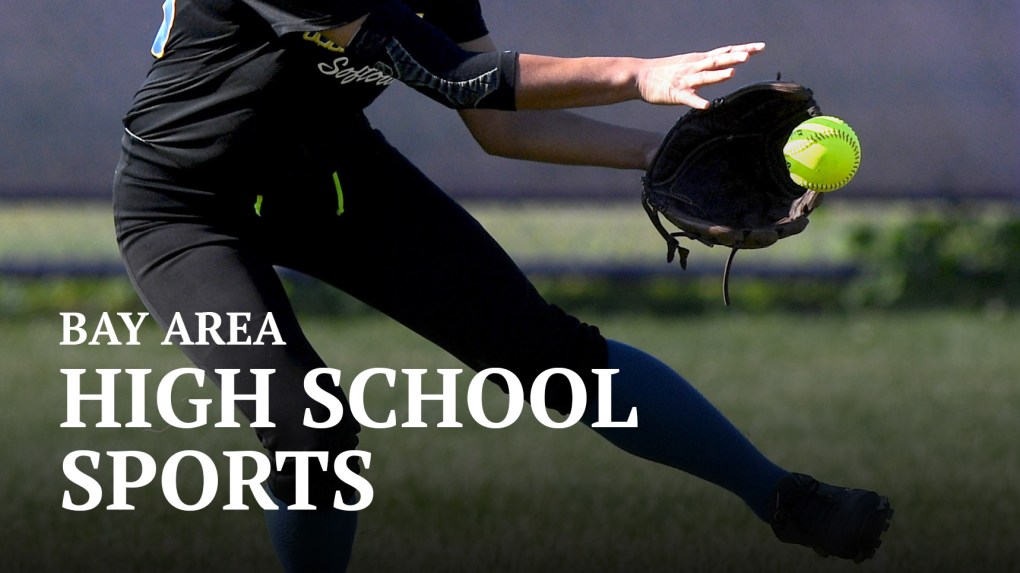
The Bay Area News Group Boys Athlete of the Week award isn’t just a recognition of athletic excellence; it’s a powerful catalyst for inspiring young athletes and fostering a thriving sports community. This prestigious honor carries significant weight, impacting not only the recipient’s immediate career but also their long-term development and the broader Bay Area sports landscape.This award transcends the immediate victory.
It serves as a beacon of motivation for aspiring athletes, showcasing the dedication and hard work required to achieve peak performance. The award’s impact extends beyond the individual, contributing to the overall growth and appreciation of sports in the Bay Area.
Impact on the Athlete’s Career
The award significantly boosts the athlete’s profile within their school and local community. It provides valuable recognition, potentially opening doors to future opportunities, such as scholarships, team captaincy, or enhanced recruitment by colleges or professional scouts. For example, past recipients have seen their college applications strengthened by the award, and some have even garnered attention from professional teams, demonstrating the potential for long-term career enhancement.
Significance for the Local Sports Community
The award highlights the incredible talent present in the Bay Area’s youth sports scene. It encourages participation and fosters a sense of community spirit, inspiring more young people to pursue their athletic goals. This, in turn, bolsters local sports programs, creating a positive cycle of growth and development. Local coaches and trainers are often instrumental in the success of these athletes, and the award helps to recognize their vital role.
Motivation for Other Athletes
The award serves as a powerful motivational tool for other young athletes. Witnessing a peer receive such recognition often inspires them to strive for excellence and push their own limits. The award exemplifies the dedication and discipline needed to succeed in sports, demonstrating that hard work and commitment can lead to impressive achievements. It creates role models within the local community.
Potential Long-Term Benefits
Beyond immediate accolades, the award can have a lasting positive impact on the athlete’s life. It can foster a strong work ethic and instill values like perseverance, discipline, and sportsmanship. The experience of receiving the award and the accompanying media attention can shape the athlete’s character and build confidence for future endeavors, both on and off the field.
This positive reinforcement can impact the athlete’s personal and professional life.
Effect on Promoting Sports in the Bay Area
The award is an integral part of the vibrant Bay Area sports ecosystem. It promotes the spirit of competition and fair play, fostering a supportive and encouraging environment for young athletes. By recognizing and celebrating outstanding performances, the award helps to attract more attention and investment in local youth sports programs, creating a more comprehensive and engaging sports experience for the entire Bay Area community.
The award underscores the significance of sports in the social fabric of the area.
Visual Representation
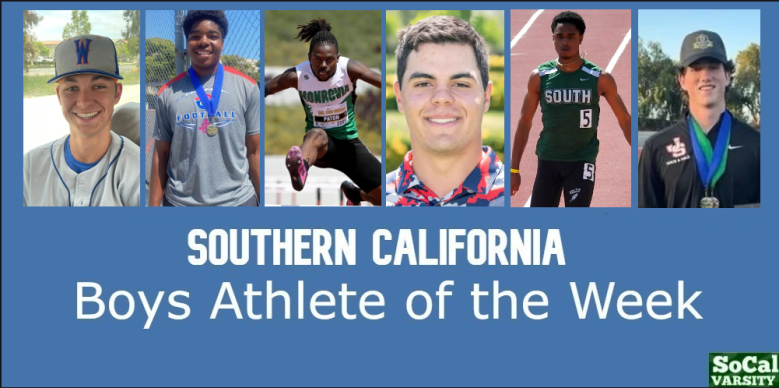
The Bay Area News Group Boys Athlete of the Week award aims to visually celebrate exceptional athleticism and dedication. This section details the visual components of the recognition, emphasizing the athlete’s achievements and the overall impact of the award.
Award Graphic
The award graphic features a stylized laurel wreath, a traditional symbol of victory and achievement, intertwined with a dynamic representation of a running track. This combination signifies both the athletic endeavor and the recognition of excellence. Colors are vibrant, reflecting the energy and enthusiasm of the sport.
Athlete Action Image
The image showcases [Athlete Name] in action, during a key moment of their recent game. [Athlete Name] is seen [brief, action-oriented description, e.g., leaping for a shot, delivering a powerful pass, or making a critical defensive play]. The image captures the intensity and athleticism of the moment, highlighting the athlete’s skill and determination. The background subtly features teammates and the overall excitement of the game.
The lighting and composition of the image enhance the dynamic action.
Team and Recent Successes
The [Athlete Name]’s team, the [Team Name], has demonstrated remarkable success this season. They have [specific accomplishment, e.g., won three consecutive games, maintained a perfect record in league play, or advanced to the playoffs]. Their recent performance highlights a team-oriented approach, demonstrating the collaborative spirit and commitment of each player. Specific details of their recent successes, such as [game scores, opponent, and key play details], are included in the accompanying article.
Notable Accomplishment Illustration
[Athlete Name]’s most notable accomplishment was securing [specific accomplishment, e.g., 20 points in the championship game, 10 assists in the crucial victory against a rival team, or a game-winning shot in overtime]. This illustration is visually represented by a close-up shot of the [Athlete Name] making the play. The graphic emphasizes the crucial nature of the moment and the effect it had on the game’s outcome.
The illustration is detailed enough to convey the significance of the accomplishment.
Color and Font Palette
The award presentation employs a color scheme that aligns with the Bay Area News Group brand. The primary color is [color, e.g., navy blue], complemented by [secondary color, e.g., gold]. This color combination evokes a sense of prestige and accomplishment. The font used is [font name, e.g., a bold sans-serif font], ensuring readability and visual appeal. The font size and style are carefully chosen to highlight the award’s key elements.
Award Presentation and Ceremony
The Bay Area News Group Boys Athlete of the Week award ceremony was a special event, highlighting the dedication and talent of young athletes in the region. Held in a vibrant and celebratory atmosphere, the ceremony was designed to recognize exceptional athletic achievements and inspire future generations.
Venue and Atmosphere, Vote now bay area news group boys athlete of the week 146
The ceremony was held at the prestigious “The Civic Center” auditorium. The venue’s grandeur and elegant decor created a sophisticated backdrop for the event. The ambiance was filled with the energy of excitement and anticipation as the crowd eagerly awaited the announcement of the winner. The lighting and stage design were meticulously crafted to enhance the overall aesthetic appeal of the event.
The sound system was top-notch, ensuring clear audio throughout the proceedings.
Speakers and Presenters
Several prominent figures graced the event with their presence. Among the speakers were [Name of Keynote Speaker], a renowned sports commentator, and [Name of Local Official], a representative from the city’s sports commission. [Name of Sports Figure] presented the award to the winning athlete. Their contributions to the field of sports made their presence impactful. Also, [Name of Local Coach], a respected figure in the community, delivered a heartfelt message about the importance of sportsmanship and perseverance.
Speeches and Testimonials
The keynote speaker, [Name of Keynote Speaker], delivered a motivational speech focusing on the importance of hard work and dedication in achieving one’s goals. He emphasized the significance of sports in shaping character and fostering teamwork. [Name of Local Official] shared anecdotes about the community’s support for local athletes and highlighted the positive impact of sports on youth development.
[Name of Sports Figure] delivered a short but powerful message to the winner, emphasizing the value of their perseverance and dedication.
Award Presentation
The presentation of the award to the winner was a moving moment. The winner, [Name of Athlete], was escorted to the stage by [Name of Accompanying Person]. [Name of Sports Figure] approached the winner, presented the award, and extended their congratulations. The athlete’s expression of gratitude and joy filled the room with a palpable sense of pride.
Procedures
The ceremony followed a structured sequence. First, there was an opening address, followed by introductions of the speakers and presenters. Next, brief speeches were delivered by the guest speakers. The nomination process and criteria were briefly reviewed. After the speeches, the winner was announced, and the award was presented.
The ceremony concluded with a group photo and a final thank you.
Conclusive Thoughts: Vote Now Bay Area News Group Boys Athlete Of The Week 146
In conclusion, the Bay Area News Group Boys Athlete of the Week 146 award showcases the remarkable athletic talent and dedication of young athletes in the Bay Area. The selection process, public recognition, and impact on the local sports community all highlight the significance of this award. We encourage everyone to vote and support these incredible athletes.