How to create heatmap in python? This guide dives deep into the world of heatmaps, powerful visual tools for exploring data patterns. From basic visualizations to interactive explorations, we’ll cover everything you need to know to master heatmap creation in Python. We’ll explore the fundamental concepts, data preparation steps, and different Python libraries, including Matplotlib and Seaborn, for creating compelling and informative heatmaps.
Understanding how to create heatmaps is crucial for data analysis in various fields. Whether you’re a data scientist, analyst, or researcher, this guide provides practical examples and techniques to help you effectively visualize data relationships and trends.
Introduction to Heatmaps in Python
Heatmaps are powerful visualization tools that display data as a color-coded matrix. They are particularly useful for identifying patterns, trends, and correlations within datasets. The intensity of the color at each point in the matrix corresponds to the magnitude of the value at that location. This allows for quick visual identification of high and low values, and the overall distribution of data points.
They are widely used in various fields like finance, marketing, and scientific research to gain insights from complex data.Understanding heatmaps in Python requires familiarity with data structures like matrices or dataframes. You need to grasp the concept of mapping numerical values to colors. Python libraries like Matplotlib and Seaborn provide the tools to create and customize heatmaps effectively.
These tools often require understanding of data manipulation techniques, including aggregation and normalization, to prepare data for effective visualization.
Fundamental Concepts of Heatmaps
Heatmaps are essentially graphical representations of matrices or tables of data. Each cell in the matrix is assigned a color based on the corresponding value. The color scale, usually a gradient from light to dark, visually represents the magnitude of the data. A key aspect is the correlation between the color and the value. This allows for immediate recognition of data patterns and trends.
Use Cases for Heatmaps
Heatmaps have a broad range of applications across diverse domains. In finance, they can be used to visualize stock market correlations, identifying potential risks and opportunities. In marketing, heatmaps can reveal user behavior on websites, allowing businesses to optimize design and user experience. In scientific research, they can be employed to display gene expression patterns or spatial distribution of data.
For example, in a sales analysis, a heatmap could highlight which products are selling well in different regions.
Example: Analyzing Data Trends
Consider a dataset showing the sales of different product categories in various regions. A heatmap can visualize the sales volume for each product category in each region. Cells with high values will be darker, indicating strong sales performance in that region for that product. Conversely, lighter cells represent lower sales. This visualization allows quick identification of high-performing product-region combinations and areas requiring attention.
Creating heatmaps in Python is surprisingly straightforward. You essentially visualize data density with varying colors, which is super useful for spotting patterns. For example, when analyzing player performance in hockey, a heatmap could show where a player like Jacob Slavin, Henry Thrun, Mario Ferraro, Jake Walman, and Mike Sullivan from the San Jose Sharks are most effective on the ice.
Python libraries like Seaborn make this process a breeze, perfect for visualizing any data.
This analysis can guide strategic decisions on product placement, marketing campaigns, and resource allocation.
Key Python Libraries for Heatmaps
The following table Artikels the key Python libraries frequently used for creating heatmaps:
Library | Description | Strengths |
---|---|---|
Matplotlib | A fundamental plotting library | Versatile plotting capabilities, customization options, great for creating basic heatmaps. |
Seaborn | Built on Matplotlib, with enhanced statistical visualizations | Provides aesthetically pleasing heatmaps with automatic color scaling and annotations, easier to use for more complex analysis. |
Data Preparation for Heatmap Creation
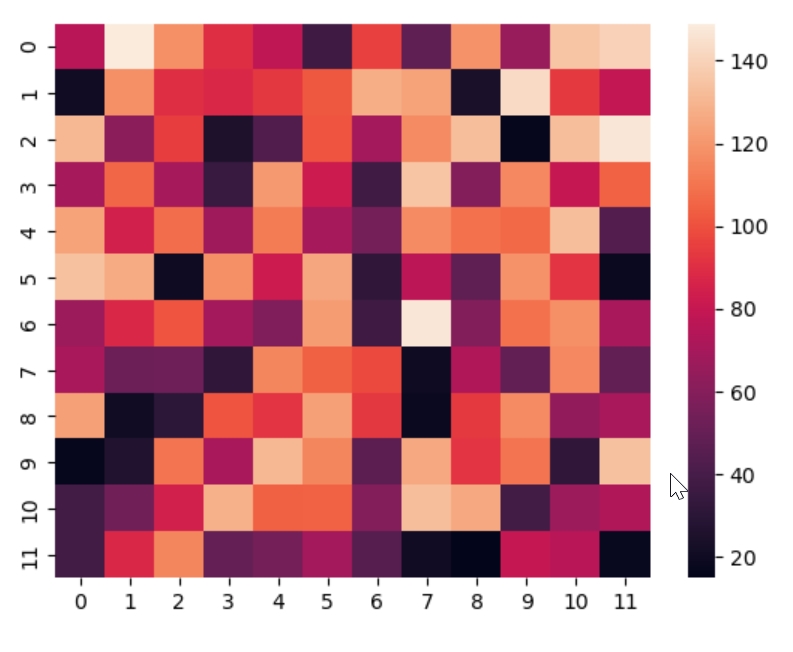
Creating effective heatmaps requires meticulous data preparation. Raw data often contains inconsistencies, missing values, and outliers that can significantly distort the visualization and lead to misleading interpretations. Thorough cleaning and preprocessing steps are crucial to ensure the heatmap accurately reflects the underlying patterns and relationships in the data. This section details the importance of data preparation, suitable data formats, and practical techniques for transforming your data into a usable format for heatmap visualization in Python.
Importance of Data Cleaning and Preprocessing
Data cleaning and preprocessing are essential for producing reliable and insightful heatmaps. Uncleaned data can obscure underlying trends, leading to inaccurate conclusions. Inconsistencies, errors, missing values, and outliers can skew the visualization, providing a distorted view of the relationships within the dataset. A well-prepared dataset ensures the heatmap accurately reflects the true patterns and correlations, leading to more reliable and actionable insights.
Common Data Formats
Various data formats are suitable for heatmap creation in Python. The most common formats include CSV (Comma Separated Values), Excel spreadsheets, and databases (like SQL). These formats provide a structured way to store and manage the data required for creating a heatmap. Choosing the right format depends on the source of the data and the tools you’re using.
Loading and Transforming Data
Loading and transforming data into a suitable format for heatmap visualization is a critical step. Libraries like Pandas in Python offer powerful tools for efficiently handling and manipulating data. The process typically involves reading the data from its source format (e.g., CSV), inspecting its structure, and performing necessary transformations to ensure it aligns with the requirements of the heatmap visualization library.
- Reading Data: Using Pandas’ `read_csv()` function, you can easily load data from a CSV file into a DataFrame. Similar functions exist for other formats like Excel and databases.
- Inspecting Data: Inspecting the DataFrame for potential issues like missing values, inconsistent data types, or outliers is crucial. Pandas provides methods like `isnull().sum()` to quickly identify missing values and `describe()` to get statistical summaries of the data.
- Data Transformation: Transforming the data to meet the heatmap’s needs is vital. This may involve reshaping the data, changing data types, or creating new variables. Pandas offers flexibility for data manipulation using functions like `pivot()`, `melt()`, and various other methods for reshaping and cleaning data.
Handling Missing Values
Missing values can significantly affect the accuracy of a heatmap. There are several strategies to address missing data. Common methods include imputation, deletion, or using special values (e.g., NaN).
- Imputation: Imputation involves replacing missing values with estimated values. Methods like mean imputation, median imputation, or more sophisticated techniques like K-Nearest Neighbors (KNN) imputation can be employed, depending on the nature of the missing data.
- Deletion: Deleting rows or columns with missing values is another approach. However, this can lead to data loss, especially if the missing values are not randomly distributed.
Handling Outliers, How to create heatmap in python
Outliers can skew the visualization of relationships in a heatmap. Identifying and handling outliers is crucial for producing a reliable heatmap. Methods for handling outliers include:
- Identification: Visualizing the data (e.g., box plots, scatter plots) or using statistical methods (e.g., Z-score, IQR) can help identify potential outliers.
- Treatment: Outliers can be removed, capped, or transformed to reduce their impact on the heatmap. The best approach depends on the specific context and the nature of the outliers.
Example: Data Manipulation with Pandas
Consider a dataset containing sales figures for different products over several months. The following code snippet demonstrates how to load the data, handle potential missing values, and transform it for heatmap creation using Pandas.“`pythonimport pandas as pd# Load data from a CSV filedata = pd.read_csv(‘sales_data.csv’)# Check for missing valuesprint(data.isnull().sum())# Impute missing values with the mean of each columndata = data.fillna(data.mean())# Create a pivot table for the heatmapheatmap_data = data.pivot_table(index=’Product’, columns=’Month’, values=’Sales’)# Display the heatmap dataprint(heatmap_data)“`This example demonstrates the basic steps for loading, cleaning, and transforming data for heatmap creation.
Remember to adapt the code and techniques based on the specific structure and characteristics of your dataset.
Creating Basic Heatmaps with Matplotlib
Heatmaps are powerful visual tools for representing data relationships. They effectively convey the intensity or magnitude of data points through color variations, making it easier to identify patterns, clusters, and outliers. Matplotlib, a popular Python plotting library, offers versatile functions for creating and customizing heatmaps, providing excellent control over the visualization process.
Generating a Simple Heatmap
A basic heatmap in Matplotlib uses a 2D array of data, where each cell’s color corresponds to its value. The following code snippet demonstrates how to create a heatmap using a sample dataset:
“`pythonimport matplotlib.pyplot as pltimport numpy as np# Sample data (replace with your data)data = np.random.rand(10, 10)# Create the heatmapplt.imshow(data, cmap=’viridis’, interpolation=’nearest’)# Add colorbarplt.colorbar()# Add labels and titleplt.xlabel(‘X-axis’)plt.ylabel(‘Y-axis’)plt.title(‘Simple Heatmap Example’)# Display the plotplt.show()“`
This code first imports necessary libraries, `matplotlib.pyplot` for plotting and `numpy` for numerical operations. It generates sample data using `np.random.rand()`. `plt.imshow()` creates the heatmap, using ‘viridis’ as the colormap, which is a perceptually uniform colormap. The `interpolation=’nearest’` argument ensures sharp edges in the heatmap. A colorbar is added to provide a visual scale for the color intensity.
Finally, axis labels and a title enhance clarity.
Customizing Color Scale and Color Map
The color scale and color map significantly impact the interpretability of a heatmap. Matplotlib provides various colormaps, each with distinct color gradients. The ‘viridis’ colormap is a good default, but others like ‘magma’, ‘plasma’, or ‘inferno’ might be more suitable depending on the data and desired aesthetic.
“`pythonimport matplotlib.pyplot as pltimport numpy as np# Sample data (replace with your data)data = np.random.rand(10, 10)# Create the heatmap with a different colormapplt.imshow(data, cmap=’magma’, interpolation=’nearest’)# Add colorbarplt.colorbar()# Add labels and titleplt.xlabel(‘X-axis’)plt.ylabel(‘Y-axis’)plt.title(‘Heatmap with Magma Colormap’)# Display the plotplt.show()“`
Modifying the `cmap` argument to ‘magma’ in the `plt.imshow()` function changes the color scheme. Adjusting the color scale, through normalization techniques, can be vital for emphasizing subtle differences in the data. This example showcases the simple modification for colormap.
Creating heatmaps in Python is surprisingly straightforward, using libraries like Seaborn. It’s all about visualizing data density, which is useful for all sorts of analyses. Speaking of visualizations, imagine a similar frustration to a passenger who paid extra for an early flight, only to end up with a later one instead. This situation, detailed in the article travel troubleshooter passenger pays extra for early flight but gets a later one instead , highlights the importance of clear data representation in travel, and similarly, how heatmaps visually display data density.
You can apply similar principles to analyzing your own data sets, using heatmaps to show trends and patterns.
Using Different Plotting Styles
Matplotlib offers various plotting styles for heatmaps beyond the basic `imshow` function. These options allow for more nuanced visualizations. For example, using `pcolor` or `pcolormesh` can offer different visual effects, suitable for various types of data.
- `pcolor` is ideal when dealing with irregular grids or when you need finer control over the edges of the heatmap cells.
- `pcolormesh` is another alternative for creating heatmaps, offering similar flexibility to `pcolor` in handling irregular grids and providing greater control over the appearance of the heatmap.
Annotating the Heatmap
Adding data values to the heatmap cells (annotations) improves clarity and provides context. This is particularly useful when dealing with categorical data or when you want to explicitly show the numerical value associated with each cell.
“`pythonimport matplotlib.pyplot as pltimport numpy as np# Sample datadata = np.random.randint(1, 10, size=(5, 5))# Create the heatmapplt.imshow(data, cmap=’viridis’, interpolation=’nearest’)# Annotate the heatmapfor i in range(data.shape[0]): for j in range(data.shape[1]): plt.text(j, i, data[i, j], ha=’center’, va=’center’, color=’black’)# Add colorbarplt.colorbar()# Add labels and titleplt.xlabel(‘X-axis’)plt.ylabel(‘Y-axis’)plt.title(‘Heatmap with Annotations’)# Display the plotplt.show()“`
This code adds annotations to each cell of the heatmap. The `plt.text()` function places the data value within each cell, enhancing the visualization.
Controlling Visualization Aesthetics
Function | Description |
---|---|
plt.imshow() |
Creates the heatmap using a given colormap. |
cmap |
Specifies the colormap (e.g., ‘viridis’, ‘magma’). |
interpolation |
Controls the interpolation method for smoother or sharper edges (e.g., ‘nearest’). |
plt.colorbar() |
Adds a colorbar to the heatmap. |
plt.xlabel(), plt.ylabel(), plt.title() |
Sets labels and title for the heatmap. |
plt.text() |
Adds text annotations to heatmap cells. |
Advanced Heatmap Creation with Seaborn
Seaborn, built on top of Matplotlib, provides a higher-level interface for creating visually appealing and informative heatmaps. It simplifies the customization process, allowing for a more sophisticated presentation of data relationships. This section will delve into Seaborn’s capabilities for heatmap creation, customization, and comparison with Matplotlib.Seaborn streamlines the creation of heatmaps by offering a concise syntax and built-in functionalities for aesthetics and data manipulation.
This approach allows data analysts to focus on the insights derived from the visualization rather than getting bogged down in low-level plotting details. The following sections explore how Seaborn excels in producing sophisticated heatmaps.
Seaborn’s Enhanced Heatmap Functionality
Seaborn provides a powerful set of tools for customizing heatmaps, making them more informative and visually appealing. These include options for controlling color scales, annotating data points, and modifying the visual aesthetics of the plot.
- Color Scales: Seaborn offers various color palettes, enabling the selection of schemes that effectively highlight data patterns. For example, diverging color palettes are suitable for visualizing data with positive and negative values, while sequential palettes work well for data with a range of values.
- Annotation Customization: Seaborn allows for detailed annotation of data points on the heatmap, providing additional context to the visualized relationships. Annotations can include values, labels, or other relevant information directly on the heatmap cells. This makes it easy to identify specific relationships in the data.
- Data Normalization: Seaborn’s built-in functionalities include options for normalizing the data, which can be useful when dealing with datasets with varying scales. This ensures that different variables contribute equally to the heatmap’s visualization, avoiding misleading conclusions.
Creating a Customized Heatmap with Annotations
Seaborn’s annotation capabilities allow for highly customized heatmaps. This example shows how to create a heatmap with annotated values, emphasizing specific relationships within the data.“`pythonimport seaborn as snsimport matplotlib.pyplot as pltimport numpy as np# Sample data (replace with your data)data = np.random.rand(10, 10)# Create the heatmapsns.heatmap(data, annot=True, fmt=”.2f”, cmap=”viridis”, linewidths=.5)# Set title and labelsplt.title(‘Correlation Matrix Heatmap’)plt.xlabel(‘Variables’)plt.ylabel(‘Variables’)# Display the plotplt.show()“`This code snippet generates a heatmap using Seaborn’s `heatmap` function.
The `annot=True` parameter displays the values within each cell. `fmt=”.2f”` formats the annotations to two decimal places. The `cmap=”viridis”` argument selects a color scheme, and `linewidths=.5` adds a thin separation between cells for better readability. The title and labels are set for clarity.
Seaborn vs. Matplotlib for Heatmaps
Seaborn offers several advantages over Matplotlib for creating heatmaps. It simplifies the process, allowing users to focus on insights rather than the technical aspects of plotting.
Feature | Matplotlib | Seaborn |
---|---|---|
Syntax Complexity | More complex, requiring more lines of code | More concise and user-friendly |
Customization Options | Limited | Extensive and intuitive |
Aesthetics | Less sophisticated | More visually appealing |
Data Handling | More manual | More integrated and automatic |
Seaborn’s integrated data handling and visualization features, combined with its enhanced aesthetics, make it a more effective choice for generating high-quality heatmaps compared to Matplotlib. This is especially true when the focus is on extracting insights from the data rather than meticulously controlling every aspect of the plot.
Handling Different Data Types
Heatmaps are incredibly versatile visualization tools, but their effectiveness hinges on how well they represent the underlying data. This section delves into creating heatmaps for various data types, emphasizing the importance of choosing appropriate color schemes and methods for handling different data characteristics. We’ll explore numerical, categorical, and mixed data, along with techniques for visualizing correlation matrices and time series data using heatmaps.Handling diverse data types in heatmaps requires careful consideration.
Different data types demand different approaches to ensure the visualization accurately reflects the data’s properties and relationships. Categorical data, for example, needs specialized treatment to avoid misinterpretations. This section will guide you through these considerations, providing practical examples and best practices.
Numerical Data
Numerical data is the most straightforward type for heatmaps. The values directly influence the color intensity of the cells in the heatmap. A higher numerical value usually corresponds to a darker shade. For instance, a heatmap visualizing sales figures across different regions would show higher sales with darker shades in the corresponding cells. A simple example in Python, using Matplotlib, would be:“`pythonimport matplotlib.pyplot as pltimport numpy as npdata = np.random.rand(5, 5)plt.imshow(data, cmap=’viridis’)plt.colorbar()plt.show()“`This code generates a 5×5 matrix of random numbers and displays it as a heatmap, using the ‘viridis’ colormap, which effectively shows the numerical values’ distribution.
Categorical Data
Categorical data, such as different product types or customer segments, needs special handling. Directly using numerical values for categorical data can lead to misleading visualizations. A common approach is to create a numerical encoding for the categories. For example, if you have categories ‘A’, ‘B’, and ‘C’, you could assign them numerical values 1, 2, and 3, respectively.
This allows for heatmap creation using the encoded numerical values. A more sophisticated approach uses a specific color scale to represent each category, like using different colors for each category, instead of shading intensities.“`pythonimport seaborn as snsimport pandas as pdimport matplotlib.pyplot as pltdata = pd.DataFrame(‘Category’: [‘A’, ‘B’, ‘C’, ‘A’, ‘B’], ‘Value’: [10, 15, 12, 8, 18])# Using a numerical encodingencoded_data = pd.get_dummies(data, columns=[‘Category’])sns.heatmap(encoded_data.corr(), annot=True, cmap=’coolwarm’)plt.show()“`This example demonstrates encoding categorical data (‘Category’) into numerical representations, then uses Seaborn to create a correlation heatmap.
Mixed Data
Heatmaps can handle mixed data types, combining numerical and categorical data. The key is to appropriately process and prepare the data. Consider a scenario where you want to visualize customer preferences (categorical) against product ratings (numerical). You could use different color scales or shading intensities for the numerical and categorical data.“`pythonimport seaborn as snsimport pandas as pdimport matplotlib.pyplot as pltdata = pd.DataFrame(‘Product’: [‘A’, ‘B’, ‘C’, ‘A’, ‘B’], ‘Rating’: [4, 5, 3, 4, 5], ‘Preference’: [‘High’, ‘Medium’, ‘Low’, ‘High’, ‘Medium’])plt.figure(figsize=(10, 6))sns.heatmap(data.corr(), annot=True, cmap=’coolwarm’)plt.show()“`This example demonstrates using both numerical and categorical data to generate a correlation heatmap.
Correlation Matrices
Correlation matrices are a special case where heatmaps are highly effective. A correlation matrix shows the correlation coefficients between different variables. A heatmap visually represents the strength and direction (positive or negative) of these correlations. Strong positive correlations appear in dark red, while strong negative correlations appear in dark blue. The intensity of the color represents the strength of the correlation.
Time Series Data
Visualizing time series data with heatmaps requires reshaping the data into a matrix-like structure. For example, you might have daily stock prices over several months. The rows could represent days, and the columns could represent different stocks. The heatmap would then visualize the price fluctuations over time for each stock.
Interactive Heatmaps: How To Create Heatmap In Python
Interactive heatmaps elevate static visualizations by enabling users to explore data dynamically. They provide a powerful way to delve into complex datasets, allowing for interactive manipulation and analysis. This approach transforms data exploration from a passive experience to an active engagement with the underlying information.
Interactive Heatmap Libraries
Interactive heatmaps leverage libraries like Plotly and Bokeh to provide dynamic visualizations. These libraries excel in creating interactive plots that respond to user actions, such as hovering, zooming, and clicking. The choice between Plotly and Bokeh depends on the specific needs and desired level of interactivity.
Interactive Elements
Interactive heatmaps offer a range of features for enhanced data exploration. Tooltips are a valuable addition, providing context about specific data points when the user hovers over them. Zooming capabilities allow for detailed examination of specific regions of the heatmap. Clickable elements transform the heatmap into a data exploration tool, enabling direct interaction with individual data points.
Creating heatmaps in Python is surprisingly straightforward. You essentially map data points to colors, visualizing patterns and trends. For instance, if you’re analyzing customer behavior data, a heatmap could show which parts of a website are most popular. Similarly, JC Penney is attempting a significant transformation, as detailed in this article about jcpenney trying reinvent itself , likely using similar data analysis techniques to identify areas for improvement.
Python libraries like Seaborn make heatmap creation a breeze, and offer various customization options for diverse datasets.
These features foster deeper insights and understanding of the data’s nuances.
Interactive Heatmaps with Clickable Elements
Clickable elements on an interactive heatmap allow users to directly access detailed information about the data point represented by the clicked cell. This approach provides an intuitive way to drill down into the underlying data. When a user clicks a specific cell, additional information, such as associated values or descriptive labels, can be displayed. This facilitates deeper investigation and understanding of the data patterns.
Advantages of Interactive Heatmaps
Interactive heatmaps offer several advantages for data exploration:
- Enhanced User Engagement: Interactive elements encourage users to actively explore the data, leading to a deeper understanding of patterns and trends.
- Improved Data Analysis: Zooming and tooltips enable users to focus on specific regions and obtain immediate context about data points, improving analytical capabilities.
- Intuitive Data Exploration: Clickable elements transform the heatmap into an intuitive tool for data exploration, facilitating quick access to detailed information about specific data points.
- Facilitated Data Storytelling: Interactive elements can be designed to tell a story by guiding the user through the data, revealing insights that might not be readily apparent from static visualizations.
Example: Interactive Heatmap with Plotly
This example demonstrates creating an interactive heatmap with Plotly, including tooltips and clickable elements.“`pythonimport plotly.graph_objects as goimport pandas as pd# Sample Data (replace with your data)data = ‘Category’: [‘A’, ‘A’, ‘B’, ‘B’, ‘C’, ‘C’], ‘Subcategory’: [‘X’, ‘Y’, ‘X’, ‘Y’, ‘X’, ‘Y’], ‘Value’: [10, 15, 12, 18, 14, 20]df = pd.DataFrame(data)# Create a pivot table for the heatmappivot_table = pd.pivot_table(df, values=’Value’, index=’Category’, columns=’Subcategory’)# Create the interactive heatmap using Plotlyfig = go.Figure(data=go.Heatmap( z=pivot_table.values, x=pivot_table.columns, y=pivot_table.index, hovertemplate=’Category: %y
Subcategory: %x
Value: %z
Then, it utilizes `pd.pivot_table` to organize the data into a suitable format for a heatmap. Crucially, it defines a `hovertemplate` for tooltips, which display category, subcategory, and value upon hovering. The `fig.show()` command renders the interactive heatmap in a web browser, allowing for interactive exploration. Customization options include adjusting the color scale, adding titles, and modifying the layout.
Advanced Techniques and Applications
Heatmaps, beyond their basic visual representation of data, can be powerful tools for uncovering deeper insights and relationships within datasets. This section delves into more advanced techniques, demonstrating how heatmaps can reveal hidden clusters, hierarchical structures, and even contribute to statistical analysis. We’ll explore applications in diverse fields, showing how heatmaps can be leveraged for problem-solving and informed decision-making.Advanced heatmap techniques often go beyond simple color gradients to incorporate clustering and hierarchical relationships, revealing patterns that might be obscured in raw data.
These techniques provide a more nuanced understanding of the data, leading to more accurate interpretations and actionable insights.
Clustering and Dendrograms
Heatmaps, when combined with clustering algorithms, become powerful tools for identifying groups or clusters within data. Clustering algorithms group similar data points together, revealing hidden structures in the data. Dendrograms, visual representations of hierarchical clustering, further illustrate these relationships. By observing the branching patterns in the dendrogram, one can understand the hierarchical structure of the clusters.
- Clustering algorithms, such as hierarchical clustering (agglomerative or divisive) or k-means clustering, can be integrated with heatmaps to identify natural groupings within the data. These algorithms group data points based on similarity, often measured by distance metrics. Hierarchical clustering results in a dendrogram, a tree-like diagram that displays the hierarchy of clusters. This visual representation can help to understand the relationships between clusters and the overall structure of the data.
- The dendrogram’s branching structure indicates the hierarchical relationship between clusters. Longer branches indicate greater distance or dissimilarity between clusters, while shorter branches signify closer relationships. This hierarchical view can reveal hidden patterns and relationships that might not be apparent in a simple heatmap alone. For example, in customer segmentation, clusters of customers with similar purchasing patterns can be identified, providing insights into marketing strategies.
Heatmaps for Identifying Clusters in Data
Heatmaps can effectively visualize the results of clustering algorithms. By coloring cells based on cluster membership, heatmaps provide a clear visual representation of how data points are grouped. This visualization aids in understanding the characteristics of each cluster and their relationships.
- Imagine a dataset of customer demographics. By clustering customers based on age, income, and location, a heatmap can highlight distinct customer segments. Different colors in the heatmap would represent different customer segments, allowing for easy identification of characteristics common to each segment. This enables targeted marketing strategies to appeal to each segment.
Visualizing Hierarchical Relationships
Heatmaps, combined with dendrograms, provide a powerful method for visualizing hierarchical relationships within data. Dendrograms, often presented alongside heatmaps, depict the hierarchical structure of clusters, revealing how data points are related to each other.
- In gene expression analysis, heatmaps can display the expression levels of genes across different samples. A dendrogram alongside the heatmap shows how genes are grouped based on their expression patterns, highlighting potential relationships between genes and diseases. This helps researchers understand gene regulatory networks and identify potential biomarkers for diseases.
Statistical Analysis and Data Mining
Heatmaps are not limited to visual exploration. They can be utilized in statistical analysis and data mining tasks. Correlation matrices, for instance, are frequently visualized as heatmaps to understand relationships between variables.
- In finance, a heatmap can visualize the correlation between stock prices. Strong positive correlations (highlighted by warmer colors) indicate that the prices tend to move in the same direction, while negative correlations (cooler colors) suggest an inverse relationship. This visual representation can aid in portfolio diversification strategies.
Applications in Machine Learning, Finance, and Scientific Research
Heatmaps are versatile tools with applications spanning diverse fields. Their ability to visualize complex relationships makes them valuable in various contexts.
- In machine learning, heatmaps can be used to analyze the importance of features in a model. By visualizing feature importance scores, one can gain insights into which features contribute most to the model’s predictions. This is particularly useful for model interpretability.
- In financial modeling, heatmaps can help analyze risk profiles by visualizing correlations between assets. This helps in understanding the diversification potential and potential risks in a portfolio.
- In scientific research, heatmaps can be used to visualize gene expression data, protein-protein interaction networks, or even climate patterns. This visual exploration aids in identifying patterns and relationships that might not be obvious from raw data.
Closing Notes
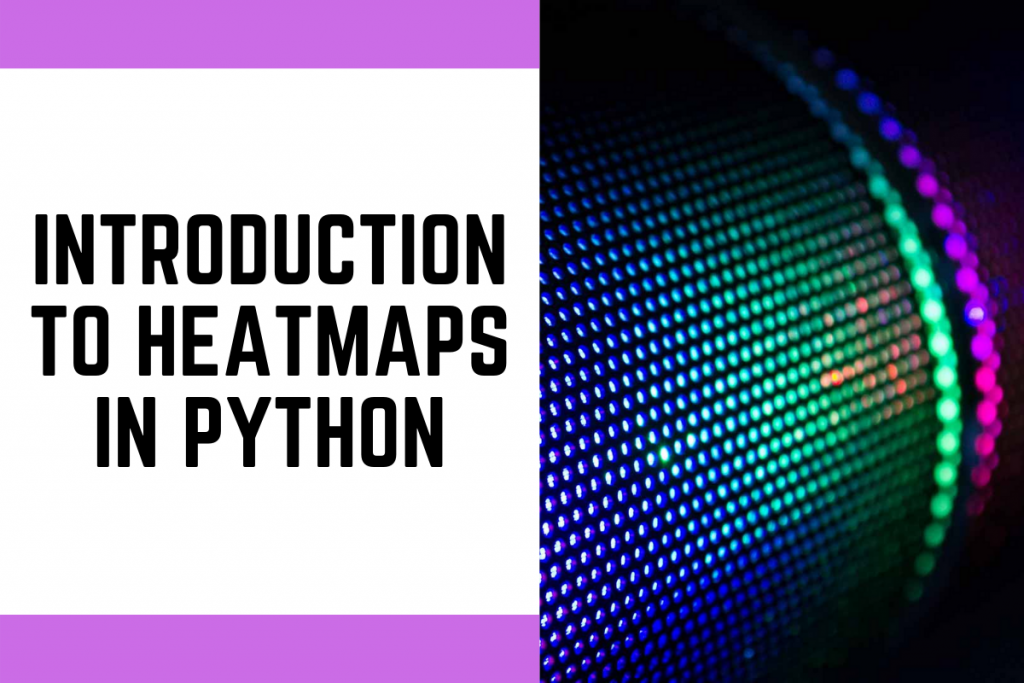
In conclusion, creating heatmaps in Python is a versatile technique for visualizing data patterns and relationships. We’ve covered the essentials, from data preparation to interactive visualizations, equipping you with the knowledge to create informative and insightful heatmaps for your projects. By mastering these techniques, you can gain valuable insights into your data and present your findings effectively.