Everything You Can Do With Python: From crafting elegant web apps to crunching complex data, Python’s versatility shines. This exploration delves into the diverse capabilities of Python, uncovering its potential in various domains. This comprehensive guide explores Python’s core programming concepts, fundamental data structures, and practical applications, comparing it to other popular languages.
Python’s adaptability is truly remarkable. It’s not just a programming language; it’s a toolbox brimming with libraries and frameworks perfect for data science, web development, automation, and even game creation. We’ll explore the key aspects of each application, providing practical examples and insights into how Python powers these different fields.
Introduction to Python Capabilities
Python stands out as a remarkably versatile programming language, transcending its initial adoption in scripting and automating tasks. Its clear syntax and extensive libraries have propelled it into various domains, from data science and machine learning to web development and scientific computing. This versatility stems from Python’s robust core concepts and powerful data structures, allowing developers to tackle complex problems with relative ease.Python’s design prioritizes readability and code maintainability.
This emphasis on clean syntax significantly reduces the cognitive load on developers, enabling them to focus on the logic and problem-solving aspects of their projects rather than wrestling with convoluted code. This, combined with a vast ecosystem of third-party libraries, equips developers with ready-made tools for a wide array of applications.
Core Programming Concepts
Python facilitates fundamental programming concepts like variables, data types, operators, control flow, and functions. These elements form the building blocks for crafting sophisticated programs. Variables store data, data types define the nature of this data (e.g., integers, strings, floats), operators perform calculations and comparisons, control flow structures (if-else statements, loops) dictate the program’s execution path, and functions encapsulate reusable blocks of code.
Fundamental Data Structures
Python offers a collection of powerful data structures to organize and manage data effectively. These include lists, tuples, dictionaries, and sets. Lists are ordered collections of items, while tuples are immutable sequences. Dictionaries provide key-value pairs for associating data, and sets store unique elements. These data structures are crucial for handling various types of data in programming tasks, from simple lists of items to complex relational databases.
Comparison with Other Languages
The table below provides a comparative overview of Python against other popular languages. The examples illustrate how Python, despite its relatively higher level of abstraction, can often achieve similar results in a more concise and readable manner.
Feature | Python | JavaScript | Java | C++ |
---|---|---|---|---|
Example (printing “Hello, World!”) | print("Hello, World!") |
console.log("Hello, World!"); |
System.out.println("Hello, World!"); |
#include |
Example (Calculating Factorial) | ```python def factorial(n): if n == 0: return 1 else: return n
``` |
```javascript function factorial(n) if (n === 0) return 1; else return n
Python's versatility is truly amazing! From automating tasks to creating complex data visualizations, the possibilities are endless. Speaking of impressive milestones, I was reading about the Cupertino's Inner Wheel celebrating their 40th anniversary, cupertinos inner wheel celebrates 40th anniversary , which reminded me how much Python can be used for analyzing trends and patterns in data, something that could potentially help with future events like this one. Python's wide range of libraries makes it perfect for a variety of projects.
``` |
```java class Factorial public static int factorial(int n) if (n == 0) return 1; else return n
public static void main(String[] args) System.out.println(factorial(5)); ``` |
```C++ #include
int main() std::cout << factorial(5) << std::endl; return 0; ``` |
Data Science and Analysis with Python
Python's versatility extends significantly into the realm of data science and analysis. Its robust ecosystem of libraries empowers users to manipulate, analyze, and visualize data effectively.
This allows for extracting meaningful insights and driving informed decisions from complex datasets. From simple calculations to sophisticated statistical modeling, Python facilitates a comprehensive data-driven approach.Python's libraries, such as Pandas and NumPy, excel at data manipulation and numerical computation, respectively. This fundamental capability forms the bedrock for more advanced analytical techniques. Statistical analysis tools, often integrated within these libraries, allow for hypothesis testing, correlation studies, and other valuable insights.
Visualizations further enhance the understanding of data trends, patterns, and relationships, making it easier to convey complex information to a wider audience.
Data Manipulation Libraries
Python offers a powerful suite of libraries for manipulating data, including Pandas. Pandas provides DataFrame objects, enabling efficient data organization, filtering, and transformation. Data cleaning and preparation, crucial steps in any data analysis project, are significantly streamlined with Pandas. Furthermore, NumPy, another essential library, excels in numerical computation. Its array structures optimize performance for complex calculations, essential for scientific computing and data analysis.
Statistical Analysis Techniques
Python provides access to a wide range of statistical methods through libraries like SciPy and Statsmodels. These libraries offer functions for hypothesis testing, regression analysis, and statistical modeling. For instance, linear regression models can be used to understand the relationship between variables, enabling predictions based on observed data.
Visualization Techniques
Python's visualization capabilities, spearheaded by Matplotlib and Seaborn, offer a diverse array of plot types. Histograms, scatter plots, and box plots can reveal insights into data distributions and relationships between variables. Seaborn, built on top of Matplotlib, simplifies the creation of visually appealing and informative visualizations. These tools make it easy to transform raw data into compelling visual representations.
Data Analysis Tasks
Python facilitates a wide spectrum of data analysis tasks. These include, but are not limited to, exploratory data analysis (EDA), where trends and patterns are identified within data sets. Descriptive statistics, providing summaries of key characteristics of data, are also readily achievable. Predictive modeling, where algorithms predict future outcomes based on historical data, is another important area supported by Python.
Further, Python can perform diagnostic analysis to identify the root cause of problems within data. Finally, prescriptive analysis suggests actions to optimize outcomes based on analyzed data.
Popular Python Libraries for Data Science
Library | Purpose |
---|---|
Pandas | Data manipulation |
NumPy | Numerical computing |
Matplotlib | Data visualization |
Scikit-learn | Machine learning |
Web Development with Python: You Can Do With Python
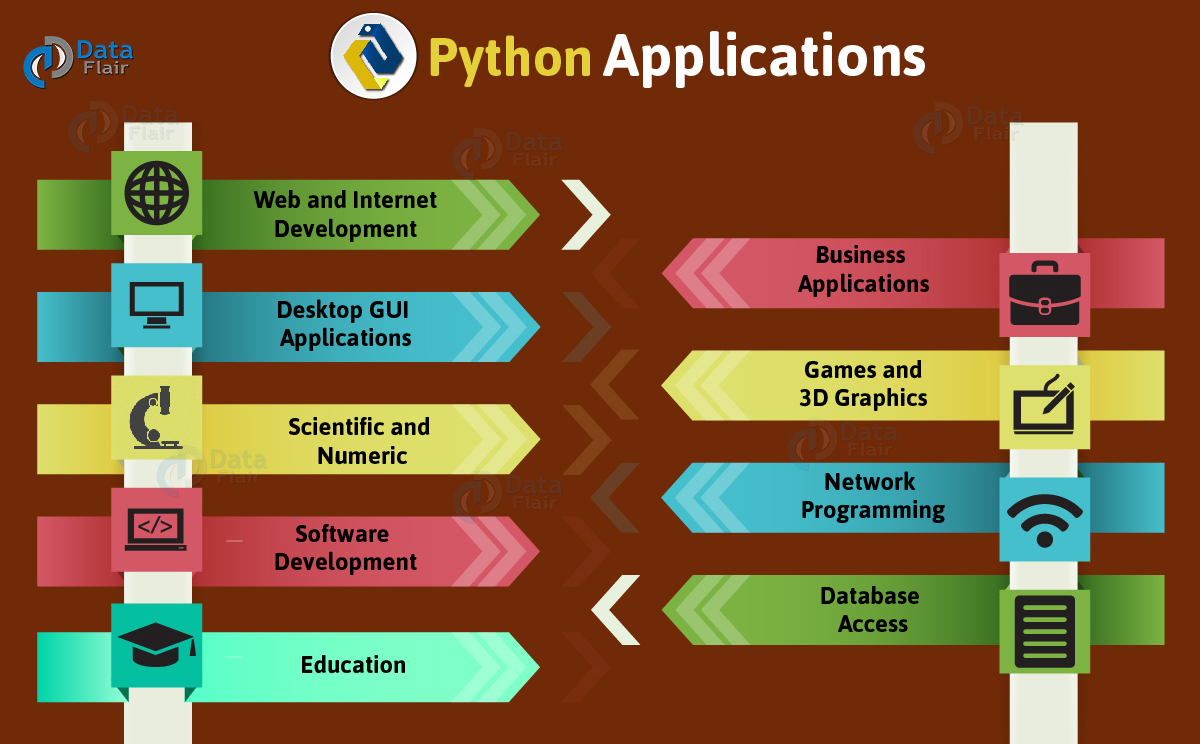
Python, renowned for its versatility, extends its reach into the realm of web development with a powerful set of frameworks. These frameworks provide a structured approach to building dynamic web applications, automating tasks, and handling complex interactions between the server and client. They simplify the development process by offering pre-built components and functionalities, enabling developers to focus on application logic rather than low-level details.Python's web frameworks excel in handling user requests, processing data, and generating responses.
They form the backbone of modern web applications, supporting diverse functionalities from simple static pages to intricate interactive platforms. Understanding these frameworks is crucial for crafting effective and scalable web solutions.
Python Web Frameworks
Python boasts a plethora of robust web frameworks, each with its own strengths and characteristics. Popular choices include Django and Flask. Django, a high-level framework, provides a comprehensive structure with built-in features like authentication, templating, and database interaction. Flask, on the other hand, is a microframework, offering more flexibility and control over the application's structure. Choosing the right framework depends on the project's scale and requirements.
Creating a Basic Web Application
Building a basic web application using Python involves several steps. First, a framework is selected, such as Flask. Next, the necessary dependencies are installed using tools like pip. Then, the application's core logic is implemented, including routing, handling user requests, and processing data. Finally, the application is deployed to a web server, making it accessible to users.
The process typically involves defining routes, handling requests, and rendering responses.
Web Development Patterns
Python frameworks support various web development patterns, each tailored for specific use cases. Model-View-Controller (MVC) is a common pattern where the application's logic is divided into three interconnected components: the model (data), the view (presentation), and the controller (interaction). This pattern promotes modularity and maintainability. Other patterns include Template Engine and RESTful API, each contributing to specific functionalities.
Structure of a Typical Python Web Application
A typical Python web application follows a structured approach. It often comprises multiple files containing different functionalities, like controllers for handling requests, models for interacting with data, and views for rendering the user interface. The application's core logic is encapsulated in these modules, making the codebase organized and manageable. This structure allows for efficient collaboration and maintenance as the application grows.
Essential Components of a Web Application, You can do with python
Component | Description |
---|---|
Server | Acts as the intermediary between the client and the application, handling incoming requests and sending responses. |
Client | Represents the user's web browser, sending requests to the server and displaying the received responses. |
Database | Stores and manages the application's data, enabling persistent storage and retrieval of information. |
Framework | Provides a structure and tools for developing web applications, abstracting away low-level details and simplifying the process. |
Automation and Scripting with Python
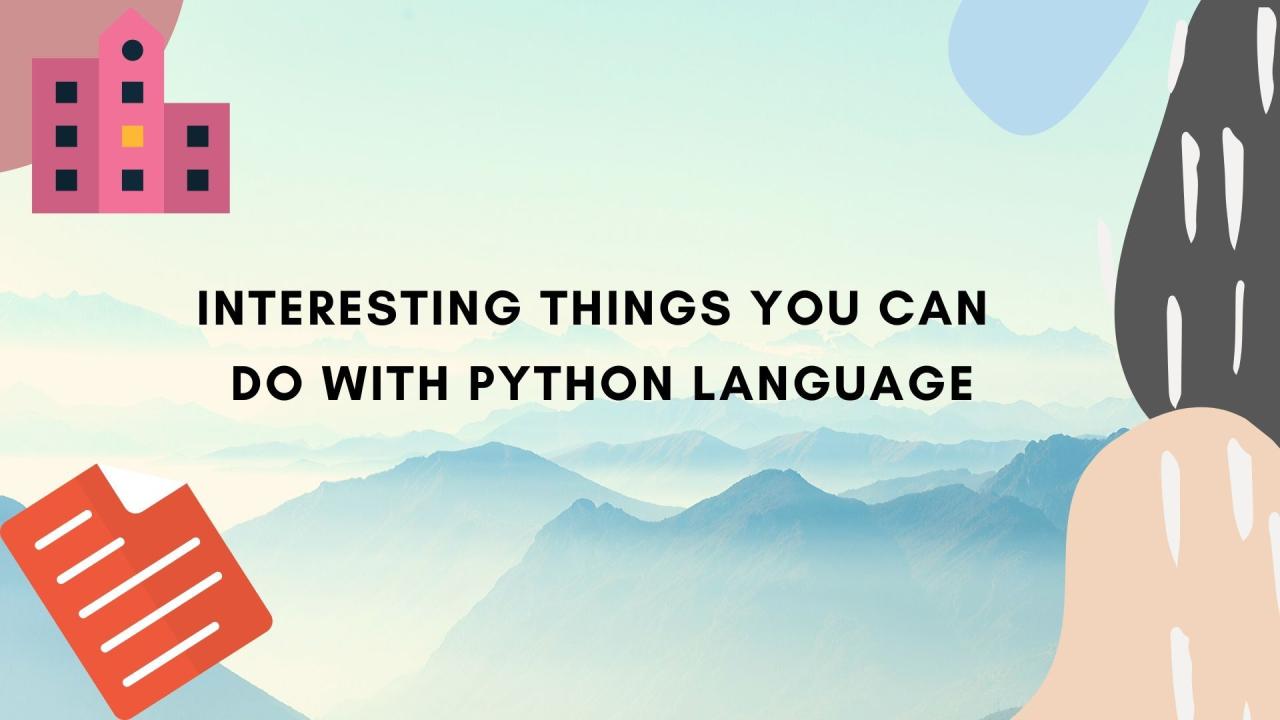
Python excels at automating repetitive tasks, freeing up human time for more creative and strategic endeavors. Its simple syntax and extensive libraries make it a powerful tool for system administration, data processing, and more. This section delves into the practical applications of Python for automation and scripting, demonstrating its capabilities and benefits.Python scripts can streamline numerous tasks, from simple file manipulations to complex system administration procedures.
This efficiency translates to significant time savings and reduced error rates in repetitive processes. The core strength lies in its ability to handle diverse tasks with concise and readable code.
Common Automation Tasks
Python scripts are highly versatile and can be tailored to address a wide array of automation needs. Common tasks include file management, data processing, system monitoring, and even web interaction. The flexibility of Python allows developers to build scripts that automate any process where repetition is involved.
Automating Repetitive Tasks with Python Scripts
A fundamental advantage of Python is its ability to execute a series of actions automatically. This can be achieved through concise code, making tasks that would normally require significant manual effort manageable. For instance, a script can be designed to automatically back up files, rename a set of files in a folder, or perform data transformations in a spreadsheet.
This efficiency is crucial for streamlining workflows and improving productivity.
Benefits of Using Python for Scripting
Python's versatility and readability are key advantages for scripting. Its clear syntax reduces the learning curve, allowing individuals to quickly write and understand scripts. This ease of use translates to faster development cycles and lower maintenance costs. Additionally, the extensive libraries provide pre-built functions for numerous tasks, reducing the need for extensive coding.
Automating System Administration Tasks
Python scripts can significantly enhance system administration tasks. Imagine automating the creation of user accounts, the management of network configurations, or the monitoring of system logs. Python scripts can perform these tasks reliably and consistently, minimizing human error and freeing up administrators for more complex tasks. Examples include scripting the deployment of software packages across multiple servers, monitoring server resource usage, or triggering automated responses to system events.
Python Libraries for Automation
The following table highlights some of the most commonly used Python libraries for automation, outlining their purpose and illustrative use cases.
Library | Purpose | Example Use Case |
---|---|---|
os |
Operating system interactions | File manipulation, directory creation, and file deletion. |
shutil |
High-level file operations | Moving directories, copying files, and archiving files. |
subprocess |
Running external commands | Launching a program, executing shell commands, and interacting with other applications. |
Game Development with Python
Python's versatility extends beyond web development, data science, and automation. Its use in game development is becoming increasingly popular, offering a powerful yet accessible approach for creating both simple and complex games. Python's rich ecosystem of libraries and frameworks allows developers to focus on game logic and design, rather than getting bogged down in low-level programming details.Python's readability and extensive libraries make it a great choice for game development, particularly for projects needing rapid prototyping or where code maintainability is crucial.
It allows for faster iteration cycles and easier collaboration among developers, a significant advantage in game development environments.
Python's versatility is amazing! You can build anything from simple scripts to complex web applications. Choosing the right web hosting is crucial for a smooth online experience, and finding a reliable provider like best web hosting services is key. After all, a well-structured website powered by Python code is just as much about the behind-the-scenes tech as the front-end presentation.
Python Libraries for Game Development
Python boasts several libraries specifically designed for game development, enabling the creation of visually appealing and interactive games. These libraries abstract away the complexities of graphics programming, enabling developers to concentrate on the game's core logic and features.
- Pygame is a widely used Python library for developing 2D games. It provides a set of functions and classes for handling graphics, sound, input, and other game-related tasks. Pygame simplifies the process of creating games with its straightforward API, allowing developers to focus on game logic rather than low-level graphics details. Its modular design enables developers to combine various components seamlessly to create complex game functionalities.
- PyOpenGL is a Python wrapper for OpenGL, a powerful cross-platform API for 3D graphics. It allows developers to create sophisticated 3D games, utilizing OpenGL's capabilities for rendering complex geometries, lighting effects, and textures. PyOpenGL provides the tools for handling 3D models, animations, and various rendering techniques crucial for immersive 3D game experiences. This choice is ideal for projects requiring advanced 3D graphics and realistic visuals.
Example of a Simple 2D Game using Pygame
A simple 2D game, such as a basic "ball bouncing" game, can be effectively created using Pygame. The core elements of the game would involve defining the ball's position, velocity, and direction. The game logic would handle the ball's movement and collisions with the screen boundaries. A simple event loop would continuously update the game state and redraw the screen, ensuring smooth gameplay.```pythonimport pygameimport random# Initialize Pygamepygame.init()# Set window dimensionswidth = 800height = 600screen = pygame.display.set_mode((width, height))# Ball propertiesball_radius = 20ball_x = random.randint(ball_radius, width - ball_radius)ball_y = random.randint(ball_radius, height - ball_radius)ball_speed_x = 5ball_speed_y = 5# Game looprunning = Truewhile running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # Update ball position ball_x += ball_speed_x ball_y += ball_speed_y # Bounce off walls if ball_x + ball_radius > width or ball_x - ball_radius < 0: ball_speed_x -= -1 if ball_y + ball_radius > height or ball_y - ball_radius < 0: ball_speed_y -= -1 # Clear the screen screen.fill((0, 0, 0)) # Draw the ball pygame.draw.circle(screen, (255, 255, 255), (ball_x, ball_y), ball_radius) # Update the display pygame.display.flip() # Quit Pygame pygame.quit() ```
Steps for Developing a 2D Game with Python
Developing a 2D game involves several key steps:
- Define Game Mechanics: Establish the rules and behavior of the game, outlining the interactions between objects, player actions, and outcomes. This involves planning the core game loop and the functionalities of each component.
- Design Game Elements: Plan the characters, environments, and other game components, creating a visual representation for the game elements. This includes determining the graphics and animations.
- Implement Game Logic: Translate the game's mechanics and elements into code, using libraries like Pygame to manage game objects, input handling, and graphics rendering. This step involves implementing game logic using Python code, incorporating the defined game mechanics and elements.
- Test and Debug: Thoroughly test the game to identify and resolve any errors or bugs, ensuring smooth and consistent gameplay. This step is crucial for identifying and resolving issues in the game's logic and functionality.
- Iterate and Improve: Refine the game based on testing and feedback, adjusting the game's mechanics and elements to improve the overall player experience. This iterative process allows for continuous improvement and enhancement of the game.
Game Development Libraries in Python
Library | Purpose |
---|---|
Pygame | 2D game development |
PyOpenGL | 3D graphics |
Other Applications of Python
Python's versatility extends far beyond the realms of web development, data science, and automation. Its elegant syntax and vast library ecosystem make it a powerful tool for tackling diverse problems across various fields. From scientific research to financial modeling, Python's adaptability allows it to be tailored to specific needs, fostering innovation and efficiency in numerous applications.Python's ability to integrate with other programming languages and systems makes it an ideal choice for complex projects.
Its readability and maintainability contribute to its widespread adoption in fields where collaboration and code longevity are paramount. This adaptability empowers developers and researchers to leverage Python's strength in different contexts.
Python in Scientific Research
Python's role in scientific research is increasingly prominent. Its extensive libraries, such as NumPy, SciPy, and Pandas, provide tools for numerical computation, scientific visualization, and data analysis. These libraries are crucial for handling large datasets, performing complex calculations, and generating insightful visualizations. The use of Python in scientific research allows researchers to focus on their core work, knowing that Python can efficiently manage the computational aspects of their experiments.
Python in Finance
Python is a popular choice for tasks in the financial industry. Its use in algorithmic trading allows for the creation of automated trading strategies. Python's ability to process large amounts of financial data and perform complex calculations efficiently makes it a valuable tool for developing sophisticated trading algorithms.
- Algorithmic Trading: Python enables the development of automated trading strategies based on various market factors and data analysis. This includes analyzing historical stock prices, identifying patterns, and executing trades automatically.
- Risk Management: Python libraries are used to model and analyze financial risks. This involves simulating potential scenarios, calculating probabilities of different outcomes, and creating strategies to mitigate risks.
- Fraud Detection: Python can be utilized for detecting anomalies and patterns indicative of fraudulent activities in financial transactions. This involves processing transaction data to identify suspicious activities.
Python in Education
Python's simple syntax and extensive libraries make it an excellent choice for teaching programming concepts. It's well-suited for introducing students to programming logic, data structures, and algorithms in a clear and engaging way. The availability of extensive online resources and interactive learning tools further enhances its use in educational settings.
- Teaching Programming Concepts: Python's readability and ease of use make it an ideal language for introducing fundamental programming concepts to students. Its beginner-friendly nature allows students to grasp core programming principles quickly and effectively.
- Data Analysis and Visualization: Python can be used to teach students how to analyze and visualize data, empowering them with practical skills applicable to various fields. This helps students develop critical thinking and problem-solving abilities.
- Interactive Learning Tools: Python's interactive nature allows for the development of tools and simulations to enhance the learning experience and make complex concepts more accessible to students.
Python in Other Fields
Python's applications extend beyond the examples already discussed. Its versatility enables its use in diverse fields, including:
Field | Application |
---|---|
Robotics | Python scripts can control robots, perform complex tasks, and analyze sensor data. |
Game Development | Python frameworks like Pygame provide tools for creating games and interactive simulations. |
Web Scraping | Python can be used to extract data from websites for various purposes, such as market research or data analysis. |
Bioinformatics | Python's libraries can be used for analyzing biological data, such as DNA sequences and protein structures. |
Closing Summary
In conclusion, Python's range of applications is truly impressive. Whether you're a seasoned developer or just starting your coding journey, Python offers a powerful and versatile platform for innovation. From streamlining tasks with automation to building sophisticated data science models, Python's ease of use and extensive libraries make it a go-to choice for diverse projects. This exploration only scratches the surface of what's possible, highlighting Python's potential across various industries and fields.