Node js bundler and build tools – Node.js bundler and build tools are essential for modern JavaScript development, streamlining the process of packaging and optimizing code. This guide explores the core concepts, popular choices, and practical applications of these tools, providing a comprehensive overview of their roles in creating efficient and scalable Node.js applications.
From understanding module bundling to mastering performance optimization techniques, we’ll uncover the secrets behind building robust JavaScript projects using Node.js. We’ll compare popular tools, discuss configuration strategies, and examine real-world use cases. Prepare to dive deep into the world of Node.js development!
Introduction to Node.js Bundlers and Build Tools
Node.js, a JavaScript runtime environment, empowers developers to build server-side applications. However, as projects grow, managing JavaScript codebases becomes increasingly complex. Bundlers and build tools are crucial components that streamline the development process by optimizing, packaging, and transforming code for efficient execution. They automate tasks like code transpilation, module bundling, and asset optimization, ultimately leading to faster development cycles and improved application performance.Modern JavaScript development often involves numerous modules and dependencies.
Without proper tooling, managing these dependencies and ensuring smooth integration can be challenging. Bundlers and build tools address this by handling the complexities of module resolution, dependency management, and code transformation, freeing developers to focus on application logic.
Typical Workflow in a Node.js Project Using Bundlers and Build Tools
The typical workflow involves several stages, each facilitated by the chosen bundler and build tools. First, developers write code using various modules and dependencies. Then, the bundler takes these modules and dependencies and combines them into a single, optimized file. Next, the build tools handle any necessary transformations of the code, like transpiling newer JavaScript syntax to older versions or optimizing for browser compatibility.
Finally, the optimized code is deployed and ready for use. This automation dramatically reduces manual steps, allowing developers to concentrate on building features rather than repetitive tasks.
Benefits of Using Bundlers and Build Tools for Large-Scale Applications
Large-scale Node.js applications often involve hundreds or even thousands of modules. Without bundlers and build tools, managing these dependencies would be extremely complex and time-consuming. These tools automate the process of combining and optimizing these modules, leading to significant benefits:
- Improved Performance: Bundlers optimize code for efficient execution by combining multiple files into a single file and minimizing redundant code.
- Enhanced Maintainability: Bundlers and build tools help manage dependencies, making it easier to update and maintain the application as it grows.
- Simplified Development: Automation of tasks like transpilation and asset management speeds up development cycles and allows developers to focus on building features.
- Reduced Errors: Automated dependency management helps to prevent errors associated with missing or conflicting dependencies.
Comparison of Bundlers and Build Tools
Different tools cater to varying needs and preferences. The following table provides a concise overview:
Name | Key Features | Typical Use Cases |
---|---|---|
Webpack | Highly configurable, supports various loaders and plugins, excellent for complex projects | Large-scale applications, front-end projects with many dependencies |
Rollup | Fast, smaller bundle sizes, suitable for libraries and smaller projects | Creating reusable libraries, applications with performance needs |
Parcel | Simple to set up, fast build times, ideal for rapid prototyping | Quick prototyping, smaller projects, front-end development |
Gulp | Task runner, allows customization of build processes through plugins | Custom build processes, complex automation workflows |
Grunt | Similar to Gulp, task runner for automating build tasks | Custom build processes, automation of repetitive tasks |
Popular Bundlers and Build Tools: Node Js Bundler And Build Tools
Node.js bundlers and build tools are crucial for modern web application development. They streamline the process of packaging JavaScript code into optimized modules for efficient execution in the browser. These tools significantly improve developer productivity and application performance by handling complex tasks like code transformation, dependency management, and asset optimization. Choosing the right bundler is essential for building robust and scalable applications.Understanding the diverse capabilities and limitations of various bundlers allows developers to select the most suitable tool for their project needs.
This section delves into the top contenders, highlighting their key features, strengths, and weaknesses, along with a comparative analysis of their configuration options.
Top 3-5 Popular Bundlers
The JavaScript ecosystem boasts a rich array of bundlers and build tools, each with its own strengths and weaknesses. Top choices often include Webpack, Parcel, Rollup, and Vite. These tools simplify the complexities of managing JavaScript modules, enabling developers to focus on the core logic of their applications.
Webpack
Webpack is a widely adopted bundler known for its versatility and extensibility. It excels at handling complex projects with numerous dependencies. Webpack’s modular architecture allows developers to configure and customize almost every aspect of the bundling process, enabling sophisticated build pipelines. Its strengths lie in its comprehensive configuration options, which empower developers to tailor the build process to specific needs.
However, its extensive configuration can be a learning curve for beginners, potentially increasing the complexity of the setup.
Node.js bundlers and build tools are crucial for streamlining development workflows. Thinking about how Los Gatos residents are prioritizing emergency preparedness and financial management, as highlighted in this recent article about emergency preparedness financial management among priorities for los gatos , reminds me of the importance of efficient tools in handling complex tasks. Ultimately, these tools are key to creating robust and maintainable applications, just like effective financial planning is key to a secure future.
Parcel
Parcel is a faster and more streamlined bundler that offers an easier-to-use experience compared to Webpack. It automatically handles many tasks, reducing the configuration overhead for developers. This simplicity is particularly valuable for smaller projects or teams that prioritize rapid development cycles. Parcel’s strengths lie in its ease of use and speed. However, its automatic approach might limit customization options for projects with intricate dependencies or complex build requirements.
Rollup
Rollup is a bundler focused on performance and code size optimization. It’s particularly well-suited for projects that demand small bundle sizes and fast initial load times. Rollup excels in creating optimized bundles, making it ideal for applications with demanding performance requirements. A key strength is its focus on minimizing the final bundle size, improving the user experience. However, Rollup’s configuration can be more involved than Parcel’s, potentially requiring more detailed knowledge for complex projects.
Vite
Vite is a newer bundler designed for faster development cycles. It leverages native ES modules for exceptional performance during development. This results in near-instantaneous module updates, boosting developer productivity. Vite’s strengths are its blazing-fast development experience and its support for native ES modules. A potential weakness is its relatively newer status, which may result in a smaller community support base compared to established bundlers like Webpack.
Node.js bundlers and build tools are essential for modern web development. They streamline the process of packaging up code for deployment, which is a crucial part of any developer’s workflow. Speaking of workflows, I was really impressed by the performance of Giani Clark, the Bay Area News Group Boys Athlete of the Week at Washington Fremont High School basketball, bay area news group boys athlete of the week gianni clark washington fremont basketball.
His dedication and skill are a great example of how hard work can pay off. Ultimately, these tools, like those used in web development, require similar commitment and effort to get the best results.
Supported JavaScript Syntax and Features
Bundler | ES Modules | CommonJS | JSX | TypeScript | Other Features |
---|---|---|---|---|---|
Webpack | Yes | Yes | Yes | Yes | Plugin ecosystem, loaders |
Parcel | Yes | Yes | Yes | Yes | Automatic dependency resolution |
Rollup | Yes | Yes | Yes | Yes | Optimized bundle size, excellent performance |
Vite | Yes | No | Yes | Yes | Native ES modules, fast development |
The table above details the supported JavaScript syntax and features of the mentioned bundlers. ES Modules are supported by all, and each bundler has its strengths and weaknesses in terms of how it handles various JavaScript features.
Configuration Options
The configuration options offered by these bundlers vary significantly. Webpack offers extensive customization, allowing developers to precisely control every step of the bundling process. Parcel, on the other hand, provides a more streamlined approach, automating many tasks. Rollup provides a balance between customization and ease of use. Vite prioritizes speed during development and often relies on simpler configurations.
Core Concepts and Features
Node.js bundlers and build tools are essential for modern JavaScript development. They streamline the process of packaging code for deployment, optimizing performance, and managing dependencies. Understanding the core concepts behind these tools is crucial for efficient and effective development.
Module Bundling
Module bundling is the process of taking multiple JavaScript modules and combining them into a single, optimized file. This simplifies the process of deploying and running applications. Without bundling, each module would need to be loaded and executed individually, leading to slower initial load times and increased complexity in managing dependencies. Bundlers handle the complex task of resolving dependencies between modules, creating a single, optimized file that the browser or Node.js runtime can load and execute efficiently.
Code Splitting
Code splitting is a technique that allows bundlers to divide an application’s code into smaller chunks. This approach significantly improves initial load time. Users only download the code necessary for the specific feature or page they’re currently interacting with, delaying the loading of unnecessary code. This approach is crucial for applications with extensive functionalities, enabling faster initial rendering and a smoother user experience.
Node.js bundlers and build tools are crucial for streamlining the development process, especially when dealing with complex projects. They automate tasks like combining code, optimizing performance, and managing dependencies. This kind of efficiency is something you’d expect to see when a luxurious four bedroom home sells in Saratoga for 2 7 million four bedroom home sells in saratoga for 2 7 million , reflecting the precision and planning behind such significant investments.
Ultimately, these tools ensure your applications are robust and scalable, mirroring the quality of high-end real estate transactions.
Code Optimization Techniques
Bundlers employ various code optimization techniques to improve performance. These include minification, which removes unnecessary characters from the code, and tree-shaking, which eliminates unused code from the bundle. Minification reduces the file size, resulting in faster download times. Tree-shaking improves performance by reducing the size of the final bundle, and optimizing for only the code actually used by the application.
These optimizations contribute significantly to improved application speed and resource usage.
Loaders
Build tools often utilize loaders to handle different file types, such as images, CSS, and other assets. These loaders convert these files into a format that can be integrated into the JavaScript bundle. This ensures that the entire project, including non-JavaScript assets, can be bundled and deployed effectively. The ability to manage various file types without needing separate tools for each file type is crucial for modern web application development.
Plugins
Plugins extend the functionality of build tools by adding specific tasks and configurations. They allow developers to customize the build process, enabling tailored features and actions. For example, a plugin can be used to integrate a linting tool to ensure code quality, or to generate a production-ready bundle for deployment. This customization and flexibility empower developers to adapt the build process to their specific needs.
Dependency Handling and Package Management
Bundlers and build tools are tightly integrated with package managers like npm or yarn. They automatically resolve dependencies, ensuring that all necessary modules are included in the bundle. This process is crucial for maintaining consistency across different development environments. Tools handle the complexity of dependency management, allowing developers to focus on the application logic rather than the details of module installation and configuration.
Common Configuration Options
Configuration Option | Description |
---|---|
Entry Point | Specifies the initial module to be processed by the bundler. |
Output Directory | Specifies the location where the bundled files will be placed. |
Module Resolution | Defines how the bundler will locate and resolve dependencies. |
Plugins | Allows the addition of custom tasks and configurations to the build process. |
Loaders | Enables the handling of different file types (e.g., images, CSS). |
Optimization Options | Controls code minification, tree-shaking, and other performance improvements. |
Performance Considerations
Bundlers and build tools significantly impact application performance, influencing everything from initial load times to subsequent execution speed. Efficient bundling and building processes are crucial for delivering fast, responsive applications to users. This section delves into the performance implications of these tools and strategies to optimize them.Modern web applications often rely on complex JavaScript codebases. Bundlers and build tools consolidate these disparate files into optimized, manageable bundles.
This process, however, can introduce performance bottlenecks if not handled carefully. Careful consideration of bundle size, code splitting, and runtime optimization is essential for creating performant applications.
Impact on Initial Load Time
Bundled JavaScript files, especially large ones, contribute directly to initial load times. A larger bundle means more data to download, increasing the time it takes for the application to fully render and become interactive. Optimization techniques, such as minimizing the bundle size, are therefore crucial.
Impact on Subsequent Execution Speed
Beyond initial load time, bundlers and build tools can also influence the execution speed of the application. Large bundles can lead to increased memory usage and slower runtime performance, especially when dealing with frequent updates or complex operations. Efficient bundling practices are vital for maintaining smooth and rapid application responsiveness.
Optimizing Bundle Size
Several strategies can minimize bundle size without sacrificing functionality. These include using tree shaking to remove unused code and minification techniques to reduce file size.
- Tree Shaking: Tree shaking is a powerful technique that automatically removes unused code from the final bundle. This significantly reduces the bundle size without affecting the application’s functionality. It identifies and removes code paths that are not reachable or used within the application’s execution flow.
- Minification: Minification involves removing unnecessary whitespace, comments, and formatting from the code. While this doesn’t reduce the actual code, it compresses the file size, leading to faster downloads.
- Code Splitting: Code splitting breaks down the application into smaller, more manageable chunks. Only the necessary code is loaded when needed, reducing the initial load time.
Code Splitting and Performance Enhancement
Code splitting is a crucial technique for improving application performance. It divides the application’s code into smaller, more manageable modules, loading only the necessary modules as the user navigates the application. This drastically reduces the initial load time.
- Dynamic Imports: Dynamic imports allow the loading of code modules only when they are needed. This is particularly useful for features that are not immediately required on page load, or for features that are specific to a user’s interaction with the application. This is a significant performance improvement in comparison to loading everything at once.
Examples of Code Splitting in Different Bundlers
Different bundlers employ slightly different approaches to code splitting, each with its own implications for performance.
- Webpack: Webpack uses entry points and chunks to divide the application into smaller bundles. It allows for the configuration of loading different modules based on user interactions or routes.
- Rollup: Rollup is known for its efficient code splitting mechanisms. It can create smaller and more optimized bundles, leading to faster loading times for applications.
Techniques for Improving Application Loading and Execution
Various techniques can enhance both the loading and execution speeds of applications.
- Lazy Loading: Loading resources only when they are needed, reducing initial load time and improving subsequent performance. This is especially useful for loading components that are only visible after a user action or scrolling.
- Caching: Caching frequently used assets and modules reduces the need to download them each time the application is accessed. This can significantly speed up loading times for returning users.
Modern Practices and Trends
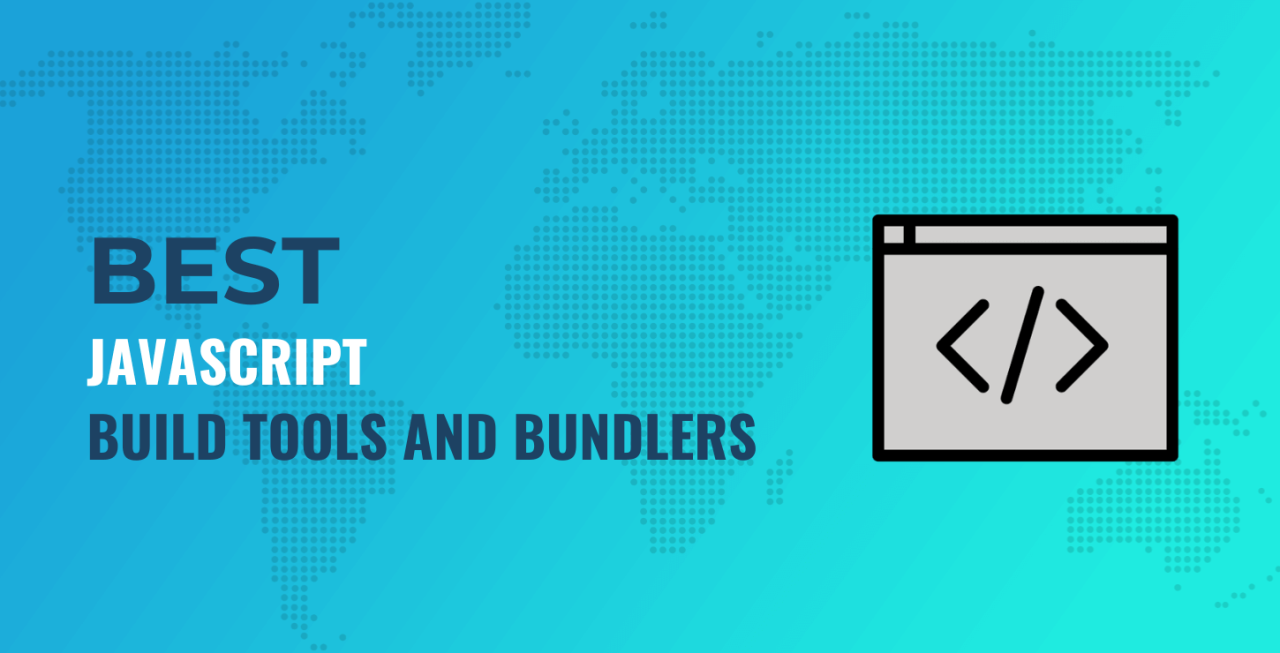
Node.js bundlers and build tools are constantly evolving to meet the demands of increasingly complex JavaScript applications. Modern practices emphasize performance optimization, efficient code management, and streamlined workflows. This evolution reflects the shift towards larger, more intricate projects and the need for robust tools to handle them effectively. Asynchronous and concurrent operations are key to unlocking the potential of these tools, allowing for faster build times and improved developer productivity.Emerging trends focus on reducing build times, improving developer experience, and enhancing the ability to manage complex projects.
These advancements are crucial for keeping pace with the rapid development cycles in modern software engineering. This section explores the latest advancements and best practices in leveraging these tools for modern JavaScript projects.
Latest Trends in Bundlers and Build Tools
Modern bundlers and build tools are adopting advanced techniques to enhance performance and maintainability. Key trends include leveraging the power of asynchronous and concurrent operations to speed up the build process. This is particularly important for large projects with numerous dependencies and modules. Also, a focus on smaller bundles is evident, reducing the size of the final application and improving loading times.
Further, features promoting modularity and maintainability are becoming standard, allowing developers to work on separate components concurrently and efficiently.
Asynchronous and Concurrent Operations
The use of asynchronous and concurrent operations in bundlers and build tools is crucial for performance. These operations allow tasks to run independently, without waiting for each other to complete. For example, if a bundler can process multiple files concurrently, it drastically reduces the build time, as each file’s processing is independent. Tools often utilize worker threads or promise-based operations to achieve this parallelism.
Maintaining Large-Scale JavaScript Projects
Managing large-scale JavaScript projects presents unique challenges, requiring tools that can handle complexity effectively. Modern bundlers and build tools are addressing these challenges by employing advanced techniques for code splitting, tree-shaking, and optimized module resolution. These mechanisms ensure that only the necessary code is bundled into the final application, reducing bundle size and improving performance. Tools like Webpack, Parcel, and Rollup have robust mechanisms for managing complex dependencies and modules, enabling seamless handling of large-scale projects.
Modern Bundlers and Build Tools
Tool | Description | Key Features |
---|---|---|
Webpack | A widely used bundler known for its flexibility and configuration options. | Module bundling, code splitting, loaders for various file types, hot module replacement (HMR), advanced configuration system. |
Parcel | A fast and simple bundler, focusing on ease of use and performance. | Fast build times, minimal configuration, supports various frameworks (React, Vue, etc.), excellent out-of-the-box support for common tasks. |
Rollup | A versatile bundler that excels in creating optimized bundles. | Highly configurable, excellent for creating small and optimized bundles, supports various formats (ES modules, CommonJS), excellent for libraries. |
Vite | A highly performant bundler that uses native ES modules and a development server. | Extremely fast development server, instant module updates, supports modern JavaScript features, ideal for web applications and frameworks. |
Real-World Use Cases and Examples
Node.js bundlers and build tools are essential for modern web application development. They automate tedious tasks, optimize code for production, and streamline the deployment process. These tools are crucial for scaling applications, ensuring performance, and maintaining a smooth development workflow. By leveraging these tools, developers can focus on building robust features instead of wrestling with complex configurations and dependencies.Employing these tools in various application types, from single-page applications (SPAs) to server-side rendering (SSR) architectures, significantly enhances the development and deployment processes.
The ability to bundle and optimize JavaScript code is critical for delivering high-performance web applications to end-users. This is especially important in today’s landscape where users expect instant responsiveness and seamless experiences.
Single-Page Applications (SPAs)
Bundlers like Webpack are indispensable for SPAs. They handle the complexities of managing multiple JavaScript files, CSS, and other assets. This allows developers to create modular, maintainable codebases. The process of combining all these resources into a few optimized files is streamlined, making the application load faster. For instance, a large SPA with numerous components and external libraries will greatly benefit from a bundler that optimizes code and reduces the number of requests to the server.
Server-Side Rendering (SSR)
SSR applications benefit from bundlers by efficiently managing the server-side rendering process. These tools handle the complex process of compiling and optimizing code for both the client and server. This approach ensures that the initial page load is faster, and that the user experience is smoother, especially for applications requiring complex data rendering or user interactions.
Large-Scale Applications, Node js bundler and build tools
In large-scale applications, build tools like Grunt or Gulp can be used to automate repetitive tasks like compiling Sass, running linters, or testing code. They significantly reduce the time and effort needed to maintain and update the application. This allows developers to focus on building features and functionalities rather than on managing build processes manually. Automated testing and code quality checks, performed during the build process, prevent issues from propagating to production and improve the overall codebase quality.
Example Project Setup with Webpack
Consider a simple React application. Webpack can bundle all the JavaScript, CSS, and images into optimized files for production. The configuration file (webpack.config.js) would define the entry point, output location, and various loaders for different asset types.“`javascript// webpack.config.jsmodule.exports = entry: ‘./src/index.js’, output: filename: ‘bundle.js’, path: path.resolve(__dirname, ‘dist’) , module: rules: [ test: /\.css$/, use: ‘css-loader’ , test: /\.(png|svg|jpg|jpeg|gif)$/i, type: ‘asset/resource’ ] ;“`This configuration would take the entry point (`src/index.js`), bundle all necessary modules, and generate the `bundle.js` file in the `dist` folder.
The `css-loader` and image loaders handle CSS and image assets, respectively, enabling Webpack to manage different file types seamlessly.
Different Steps and Output
1. Development
Webpack runs in watch mode, automatically rebuilding the `bundle.js` file whenever a source file changes. This allows for real-time feedback and rapid development.
2. Production
Webpack optimizes the `bundle.js` file further for production, reducing file size and improving performance. This process might include minification, tree shaking, and code splitting.
3. Output
The final `bundle.js` file, along with other optimized assets, is deployed to a web server. This optimized file is ready for users to access and interact with.
Choosing the Right Tool
Picking the right Node.js bundler and build tool is crucial for project success. It significantly impacts development speed, maintainability, and ultimately, the quality of the final product. Choosing the wrong tool can lead to wasted time and frustration, while a well-suited tool empowers developers to focus on the core logic of their application. A proper evaluation process considers project needs, performance requirements, and the long-term vision for the project.Understanding the nuances of each tool is vital.
This involves evaluating not just features, but also community support, learning curve, and the overall ecosystem surrounding the chosen technology. This selection process is paramount to optimizing development workflows and delivering a high-quality product.
Bundler and Build Tool Comparison
Selecting a bundler or build tool is a critical decision in any Node.js project. Different tools excel in different situations. A comprehensive comparison can help developers make an informed choice.
Feature | Webpack | Rollup | Parcel | Vite |
---|---|---|---|---|
Speed | Generally fast, but can be slower for smaller projects due to its comprehensive nature. | Very fast, especially for smaller to medium-sized projects. | Extremely fast, ideal for smaller and medium-sized projects due to its focused nature. | Exceptionally fast, especially for modern JavaScript projects, leveraging native ES modules and features. |
Features | Extremely versatile, supporting a wide range of features like code splitting, module resolution, and various loaders. | Excellent for code optimization and minimizing bundle size, particularly well-suited for libraries and components. | Simple and straightforward, focusing on speed and ease of use. | Focuses on speed and ease of use, integrating with modern tooling. |
Community Support | Extensive and active, providing abundant resources and support. | Growing rapidly and gaining significant traction in the community. | Growing community, but smaller than Webpack or Rollup. | Large and rapidly growing community, particularly strong among modern JavaScript developers. |
Learning Curve | Steep, requiring a deeper understanding of configuration options and underlying mechanisms. | Moderate, requiring familiarity with the principles of bundling. | Gentle, easily approachable by developers new to bundlers. | Relatively gentle, focusing on a straightforward approach. |
Ideal Use Cases | Complex projects, large applications with extensive dependencies. | Libraries, components, and projects focused on code size optimization. | Small to medium-sized projects, prototypes, and applications requiring rapid development. | Modern JavaScript projects, single-page applications (SPAs), and projects leveraging modern web standards. |
Factors to Consider When Choosing a Bundler
Several factors influence the optimal choice for a project.
- Project Size and Complexity: Larger, more complex projects may benefit from the comprehensive features of Webpack. Smaller projects might find Parcel or Vite more manageable.
- Performance Requirements: Projects with strict performance goals might favor Rollup or Vite for their optimized bundling.
- Team Expertise: Consider the team’s familiarity with different tools. A tool with a gentler learning curve might be more practical if the team is new to bundlers.
- Future Scalability: The chosen tool should be able to adapt to the project’s anticipated growth.
- Community Support and Ecosystem: A strong community ensures readily available support and resources for troubleshooting and learning.
Evaluating Tool Suitability
Assessing suitability depends on project requirements.
- Project Scope: A large application with diverse dependencies might demand a versatile tool like Webpack.
- Development Speed: Projects prioritizing rapid development could benefit from a tool with a user-friendly approach.
- Maintainability: Tools with well-documented configurations can simplify long-term maintenance.
Conclusive Thoughts
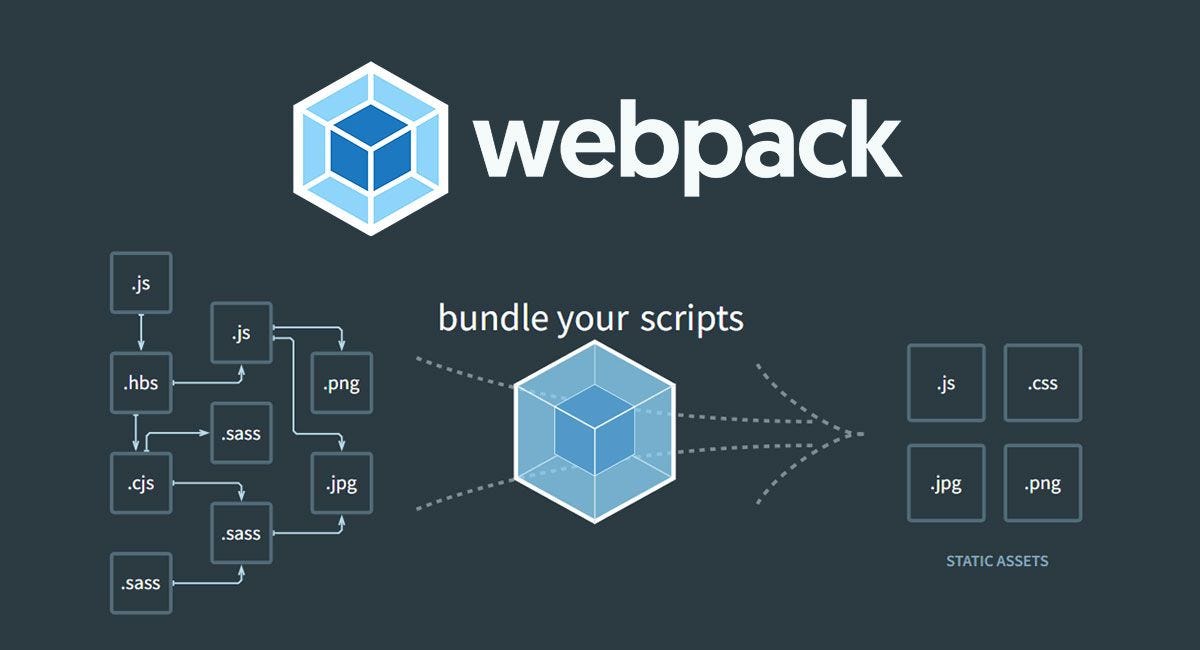
In conclusion, Node.js bundlers and build tools are powerful tools that significantly enhance the development process. They enable efficient code management, optimization, and deployment, leading to faster, more robust, and maintainable applications. By understanding the core concepts and exploring the available options, developers can leverage these tools to build high-quality, performant Node.js projects.