Shake up your workouts with the best vibration platform machines! These innovative devices offer a unique approach to exercise, promising enhanced results and a more effective workout experience. We’ll explore the different types of vibration platforms, their various mechanisms, and the potential benefits for different fitness levels. From beginner routines to advanced programs, we’ll delve into the world of vibration training, highlighting its potential to improve flexibility, strength, and balance.
This comprehensive guide covers everything from choosing the right machine to understanding safety precautions and integrating vibration platform workouts into your existing fitness regime. We’ll also examine the specific benefits and considerations for various user groups, including athletes, seniors, and individuals with injuries. Get ready to discover how vibration platforms can transform your workout routine and unlock new levels of fitness.
Introduction to Vibration Platform Machines
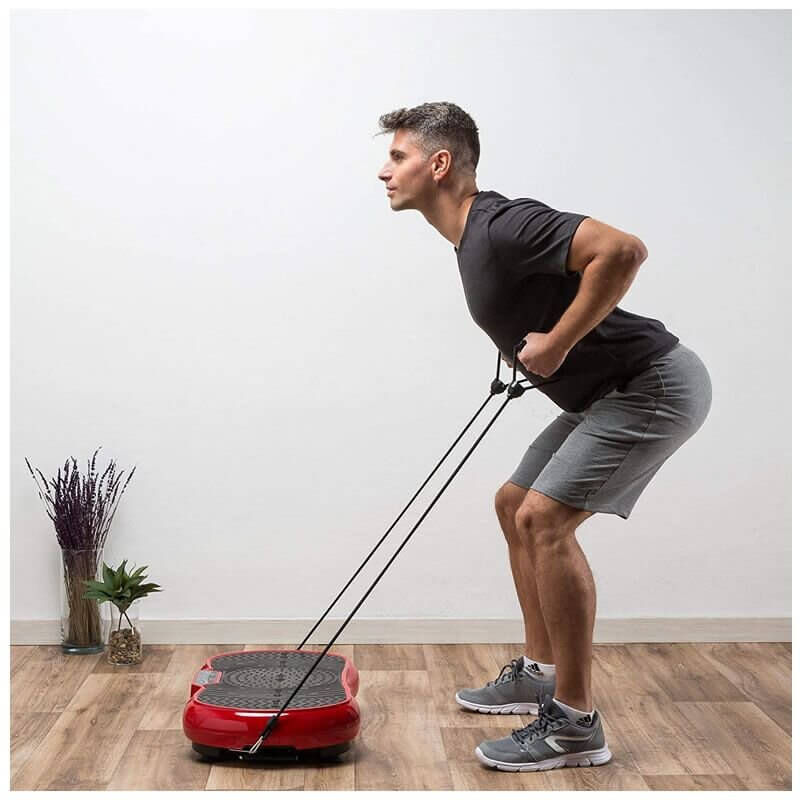
Vibration platform machines, also known as whole-body vibration (WBV) platforms, are a relatively new form of exercise equipment. These platforms utilize controlled vibrations to stimulate muscles and the body’s nervous system, promoting a variety of physical benefits. They come in various forms, from simple, home-use models to more sophisticated commercial-grade equipment.These machines work by inducing a rhythmic shaking motion, which can be tailored to different intensities and frequencies.
This controlled movement engages various muscle groups and stimulates the body’s natural responses to physical activity, leading to potentially significant improvements in fitness, posture, and overall well-being. The effectiveness of these platforms depends on proper usage and adherence to recommended protocols.
Types of Vibration Platform Machines
Vibration platforms are categorized by the type of vibration they produce. The most common types include linear vibration and rotational vibration. Linear vibration platforms primarily shake the platform back and forth, while rotational vibration platforms spin or rotate around an axis. The specific mechanism of vibration impacts the type of exercise and muscle activation.
Mechanisms of Vibration
Vibration platforms employ different vibration mechanisms to produce a variety of effects. These platforms induce vibrations, which stimulate proprioceptors – specialized sensory receptors in muscles and joints. These receptors relay information to the nervous system, leading to muscle contractions and improved coordination. The frequency and amplitude of vibration are key factors in determining the intensity and impact on the body.
Benefits of Vibration Platforms
Vibration platforms offer a range of potential benefits for users. These platforms are becoming increasingly popular for their ability to enhance muscle strength and endurance, potentially aiding in bone density maintenance and promoting improved balance and coordination. Proper usage and appropriate duration are essential for maximizing the benefits and minimizing potential risks.
Whole-Body Vibration vs. Other Vibration Training, Shake up your workouts with the best vibration platform machines
Whole-body vibration (WBV) training is distinct from other types of vibration training. While some vibration training devices might focus on specific body parts or muscle groups, WBV platforms affect the entire body simultaneously. This simultaneous engagement of muscles and the nervous system contributes to its unique benefits, potentially leading to a more comprehensive workout experience.
Comparison of Vibration Platform Machines
Model | Price | Features | User Reviews |
---|---|---|---|
Vibrating Fitness Platform | $300-$500 | Adjustable intensity, portable, simple design | Mixed reviews, some users report positive results, others find the machine ineffective. |
Advanced Vibration Platform Pro | $800-$1500 | Multiple vibration modes, advanced features, larger platform | Generally positive reviews, users praise the versatility and noticeable improvements in muscle strength and balance. |
Commercial Vibration Platform | $2000+ | High-intensity settings, multiple users, advanced control panel | Positive reviews from gyms and fitness professionals, users report significant improvements in muscle strength and overall fitness. |
Workout Enhancements with Vibration Platforms
Vibration platforms are revolutionizing the fitness landscape, offering a unique way to enhance workouts and achieve fitness goals. These platforms utilize vibrations to stimulate muscles, potentially leading to improved strength, flexibility, and overall well-being. They’re particularly beneficial for individuals seeking alternative or supplementary exercise methods, or those with limitations that hinder traditional workouts.Vibration platforms provide a powerful stimulus for muscles, effectively increasing muscle activation and promoting faster recovery times.
This can translate into quicker progress in strength training, improved balance, and potentially reduced recovery time between workouts. The platform’s subtle yet effective vibrations can target specific muscle groups and enhance overall fitness.
Exercises on Vibration Platforms
Vibration platforms allow for a wide variety of exercises, adapting to different fitness levels and goals. The platform’s controlled vibrations provide a unique training experience, enhancing the effectiveness of exercises. This is especially useful for individuals who want to challenge their muscles in new ways or who might find traditional exercises difficult or uncomfortable.
Looking for a way to shake up your workouts? Investing in the best vibration platform machines is a game-changer! They offer a unique, effective way to sculpt and tone your muscles. Plus, recent sports news, like the impressive victory of Christopher’s team in the San Jose match against Santa Teresa, highlighted the importance of a powerful, focused approach.
Check out the details on this incredible win to see how the team dominated the game, and then discover how you can similarly dominate your fitness goals with the right vibration platform equipment. These machines are a fantastic addition to any home gym routine!
- Calisthenics: Push-ups, squats, lunges, and rows can be performed on vibration platforms, often increasing the intensity and muscle engagement due to the added vibration. This can be particularly useful for individuals looking to challenge their strength and endurance. The vibrations can create a more dynamic and challenging workout, promoting muscle activation and growth.
- Strength Training: Dumbbell exercises, weightlifting, and resistance band exercises can be performed on the platform to increase intensity and challenge different muscle groups. The vibrations can help in muscle activation, and the overall training effect can be greater than traditional exercises for specific muscle groups.
- Cardiovascular Training: Activities like jumping jacks, high knees, and burpees can be done on vibration platforms to boost cardiovascular fitness and improve endurance. The added vibration can intensify the workout, providing a more efficient cardiovascular exercise.
Effectiveness Compared to Traditional Exercises
Vibration platform exercises often offer a more efficient way to target specific muscle groups, leading to improved results. The vibrations can enhance muscle contraction and relaxation, resulting in faster gains in strength and flexibility.
- Increased Muscle Activation: Studies suggest vibration platforms can significantly increase muscle activation compared to traditional exercises, leading to faster gains in strength and power. The vibrations create a more dynamic workout, potentially leading to more significant improvements in strength than traditional exercises.
- Improved Flexibility and Range of Motion: Vibration therapy can improve flexibility and range of motion. The vibrations can gently stretch muscles and increase joint mobility, potentially reducing stiffness and improving overall flexibility.
- Reduced Recovery Time: Vibration platforms are known for aiding in muscle recovery by promoting blood circulation and lymphatic drainage. This can lead to faster recovery between workouts and potentially prevent delayed-onset muscle soreness (DOMS).
Impact on Flexibility, Strength, and Balance
Vibration platforms can improve flexibility by promoting muscle relaxation and stretching. The vibrations can stimulate muscle spindles and Golgi tendon organs, contributing to improved joint mobility.
- Improved Flexibility: The vibrations help stretch muscles more effectively, improving flexibility and range of motion. This is particularly beneficial for individuals who are less flexible or have limited range of motion.
- Enhanced Strength: The platform can increase muscle activation, leading to greater strength gains. By creating a more dynamic workout, it can effectively target specific muscle groups, improving strength and power.
- Enhanced Balance: Vibration platforms stimulate the vestibular system, which is crucial for balance. Regular use can improve balance and coordination, reducing the risk of falls, especially for older adults or individuals with balance issues.
Muscle Activation and Recovery
Vibration platforms stimulate muscles and enhance blood circulation, potentially speeding up recovery. The mechanical vibrations can trigger a cascade of physiological responses, including increased muscle activation and blood flow to the muscles.
- Muscle Activation: The vibration stimulates muscle fibers to contract and relax, which promotes increased muscle activation and greater engagement during workouts.
- Muscle Recovery: Vibration therapy aids in recovery by increasing blood flow and lymphatic drainage, removing waste products from muscles and reducing inflammation. This is beneficial for recovery between workouts.
Injury Prevention and Rehabilitation
Vibration platforms can be used for injury prevention and rehabilitation. The platform’s controlled vibrations can help improve muscle strength and flexibility, reducing the risk of injury.
- Injury Prevention: By strengthening muscles and improving flexibility, vibration platforms can help prevent injuries in various activities. The controlled vibrations can be adjusted to target specific muscle groups, reducing the risk of strain or tears.
- Rehabilitation: Vibration therapy can be used to help rehabilitate injured muscles and joints. The controlled vibrations can aid in restoring strength, flexibility, and range of motion in injured areas.
Workout Routines for Different Fitness Levels
Routine Name | Duration | Equipment | Exercises |
---|---|---|---|
Beginner | 20 minutes | Vibration platform, light weights | Squats, lunges, arm circles, torso twists |
Intermediate | 30 minutes | Vibration platform, resistance bands | Push-ups, planks, burpees, dumbbell rows |
Advanced | 45 minutes | Vibration platform, free weights | Deadlifts, overhead presses, pull-ups, plyometrics |
Rehabilitation | 15-20 minutes | Vibration platform, light weights/resistance bands | Targeted muscle strengthening exercises, flexibility stretches, balance exercises |
Benefits and Considerations for Different Users
Vibration platform machines offer a versatile way to enhance fitness and well-being, but their effectiveness varies depending on the user. Understanding the specific benefits and considerations for different demographics is crucial for maximizing the platform’s potential and minimizing risks. Individual needs and limitations should always be prioritized when incorporating vibration platforms into a fitness routine.The benefits of vibration platforms extend beyond just general exercise.
They can be particularly beneficial for various age groups and individuals with specific physical needs. Proper form and technique are paramount to avoid injury and maximize results. Choosing the right machine and understanding potential contraindications are also vital.
Benefits for Different Age Groups
Vibration platforms provide a unique training modality suitable for a broad spectrum of ages. For younger individuals, they can aid in building muscle strength and improving bone density. As people age, vibration platforms can help maintain mobility and balance, potentially reducing the risk of falls. These platforms can be a beneficial tool for seniors, providing a low-impact way to maintain strength and flexibility.
Looking for a way to shake up your workouts? Investing in the best vibration platform machines can totally transform your routine. While tragic events like the recent Christmas shooting at Phoenix airport, which sadly left 3 people wounded and 1 stabbed , highlight the importance of prioritizing safety, focusing on your fitness goals can be a great way to stay positive and healthy.
These vibration platforms are a fantastic way to boost your workout routine and help you reach your fitness goals, so get ready to feel the difference!
Specific benefits for each age group vary, and the intensity and duration of workouts should be adjusted accordingly.
Suitability for Individuals with Physical Limitations
Vibration platforms can be highly beneficial for individuals with specific physical limitations or conditions. For those with limited mobility or joint pain, vibration platforms can provide a low-impact workout, promoting muscle activation and range of motion without excessive stress on joints. Individuals with balance issues can use the platform to improve proprioception and stability. The adjustable intensity and customization options allow for personalized workout routines that are safe and effective for a variety of conditions.
However, it’s crucial to consult with a healthcare professional before using vibration platforms if you have any pre-existing medical conditions.
Importance of Proper Form and Technique
Proper form and technique are crucial for maximizing the benefits and minimizing the risks associated with vibration platform use. Maintaining a neutral spine and stable posture during exercises is essential. Using the platform’s handles or support bars can provide added stability and control. It’s important to avoid leaning too far forward or backward, and to maintain a controlled movement throughout the workout.
Consistent form and technique are vital for preventing injuries.
Choosing the Right Vibration Platform Machine
Several factors influence the choice of a suitable vibration platform machine. Consider the intended use, the user’s weight and fitness level, and the space available. Machines with adjustable settings for intensity and frequency are generally more versatile. Features like built-in programs and safety mechanisms are also worth considering. Look for reputable brands known for quality and safety.
It is crucial to read reviews and compare models before making a purchase.
Potential Contraindications
- Individuals with certain medical conditions, such as uncontrolled hypertension, osteoporosis, or spinal instability, should consult with their doctor before using vibration platforms.
- Pregnant women should avoid using vibration platforms unless advised by a healthcare professional.
- People with recent injuries or surgeries should exercise caution and consult with their physician before using vibration platforms.
- Individuals with pacemakers or other implanted medical devices should avoid using vibration platforms.
- Individuals with severe arthritis or other conditions that cause joint pain should use caution and adjust intensity as needed.
Careful consideration of these factors can help ensure a safe and effective workout.
Comparison of Benefits for Different User Groups
User Group | Enhanced Physical Aspects | Considerations | Precautions |
---|---|---|---|
Athletes | Increased muscle strength, improved power output, enhanced neuromuscular coordination, and potentially faster recovery. | Intensity and frequency should be tailored to their specific training goals and current fitness level. | Proper warm-up and cool-down routines are essential. Monitor for signs of overexertion. |
Seniors | Improved balance, reduced risk of falls, increased muscle strength, and improved bone density. | Lower intensity and shorter durations of workouts are generally recommended. Close supervision or assistance may be necessary. | Consult with a physician before starting a vibration platform program. Ensure proper support and stability. |
Individuals with Injuries | Reduced pain, improved range of motion, and increased muscle activation. | Intensity should be adjusted based on the specific injury and the recommendations of a healthcare professional. | Avoid any movements that exacerbate pain. Listen to your body and discontinue use if discomfort arises. |
Safety and Precautions When Using Vibration Platforms
Vibration platforms offer a convenient way to enhance workouts, but understanding the potential risks and taking necessary precautions is crucial for a safe and effective experience. Ignoring these precautions can lead to injuries, especially for individuals with underlying health conditions. This section details the potential risks, safety guidelines, and recommendations for minimizing those risks, ensuring a positive and injury-free experience.
Potential Risks Associated with Vibration Platforms
Vibration platforms, while generally safe, can pose risks if not used properly. These include musculoskeletal issues like sprains, strains, and joint pain. Improper form, excessive intensity, or lack of adequate warm-up can exacerbate these risks. Furthermore, individuals with certain pre-existing conditions may experience adverse reactions or exacerbate existing problems. The vibrations can also cause discomfort or pain in sensitive areas.
Users must be mindful of their bodies’ responses and adjust intensity or stop use if discomfort arises.
Minimizing Risks of Injury
To minimize the risks of injury, users must adhere to specific guidelines. A crucial step is proper form and technique. Maintaining a stable posture throughout the workout is essential to prevent falls or injuries. Users should avoid sudden movements or jarring actions while on the platform. It’s also important to gradually increase the intensity and duration of workouts.
Starting with shorter sessions at lower settings allows the body to adapt to the vibrations, reducing the risk of strain or injury.
Safety Guidelines and Precautions for Different Physical Conditions
Safety guidelines for users with various physical conditions are paramount. For instance, pregnant women should consult their doctor before using vibration platforms, as the vibrations might affect the developing fetus. Similarly, individuals with joint problems, such as arthritis or osteoporosis, should proceed with caution and consult a doctor to assess their suitability for vibration platform workouts. People with cardiovascular issues or blood pressure concerns should also consult their physician.
The intensity and duration of workouts should be adjusted according to individual tolerance and limitations.
Warm-up and Cool-down Routines
Proper warm-up and cool-down routines are essential before and after using vibration platforms. A 5-10 minute warm-up, involving dynamic stretches like arm circles, leg swings, and torso twists, prepares the muscles for the vibrations. This helps to prevent muscle strains and soreness. A cool-down period of similar duration, focusing on static stretches, helps the body recover from the workout and prevents stiffness.
Examples of suitable stretches include holding hamstring stretches, quadriceps stretches, and calf stretches.
Importance of Consulting a Healthcare Professional
Before starting any new workout routine, including one involving vibration platforms, consulting a healthcare professional is crucial. This is especially true for individuals with pre-existing medical conditions or those who are unsure about their fitness level. A doctor can assess individual needs and provide tailored advice on suitable intensity levels, duration, and safety precautions. This personalized approach is vital for maximizing benefits while minimizing risks.
Safety Precautions and Contraindications Table
Condition | Precautions | Symptoms to Watch For | Recommendations |
---|---|---|---|
Pregnancy | Consult a doctor before use. Avoid high-intensity workouts. | Discomfort, pain, or unusual sensations. | Lower intensity, shorter durations. |
Joint Pain/Arthritis | Start with low intensity and gradually increase. | Increased pain, swelling, or stiffness in joints. | Consult a physician for personalized recommendations. |
Cardiovascular Issues | Monitor heart rate closely. Stop if experiencing chest pain or dizziness. | Shortness of breath, chest pain, dizziness, or lightheadedness. | Consult a cardiologist before use. |
Blood Pressure Concerns | Monitor blood pressure before, during, and after use. | Significant fluctuations in blood pressure, dizziness, or lightheadedness. | Consult a doctor for personalized recommendations. |
Epilepsy | Avoid use due to potential triggers. | Seizures or any other neurological symptoms. | Seek alternative workout options. |
Examples of Effective Workout Routines
Unlocking the full potential of vibration platform machines requires tailored workout routines. These routines are not just about exercising; they’re about maximizing the unique benefits of vibration training, ensuring safety, and achieving personalized fitness goals. This section will detail beginner, intermediate, advanced, and senior-specific routines, offering clear instructions and schedules to guide you.Understanding the principles behind each routine and adhering to the suggested intensity and duration is crucial for effective results.
Remember to consult your physician before starting any new exercise program.
Beginner Workout Routine
This routine focuses on building a foundation of strength and endurance, introducing your body to the vibrations and movements. It’s designed for individuals new to vibration training or returning to exercise after a break.
- Warm-up (5 minutes): Light cardio like walking or jumping jacks, followed by dynamic stretching (arm circles, leg swings). This prepares your muscles for the vibration platform workout.
- Vibration Platform Exercises (20-30 minutes):
- Standing calf raises (3 sets of 10-12 repetitions). Stand on the platform with your feet shoulder-width apart, and lift your heels off the ground, engaging your calf muscles.
- Quadriceps extensions (3 sets of 10-12 repetitions). Stand on the platform, engaging your core, and extend your legs in a controlled motion, targeting your quadriceps.
- Glute bridges (3 sets of 10-12 repetitions). Lie on the platform with your knees bent and feet flat on the platform. Engage your glutes to lift your hips off the ground, holding for a brief moment.
- Cool-down (5 minutes): Static stretching, holding each stretch for 20-30 seconds. Focus on the muscles worked during the workout, such as hamstrings, quads, and calves.
Ideal intensity: Low to moderate vibration intensity, focusing on controlled movements. Duration: 2-3 times per week.
Intermediate Workout Routine
This routine targets specific muscle groups, building upon the foundation established in the beginner routine. It’s ideal for individuals seeking to enhance muscle definition and strength.
- Warm-up (5 minutes): Dynamic stretches targeting major muscle groups (legs, arms, torso).
- Vibration Platform Exercises (30-45 minutes):
- Targeted Muscle Groups: Chest presses (3 sets of 12-15 repetitions), shoulder presses (3 sets of 12-15 repetitions), triceps extensions (3 sets of 12-15 repetitions). Use higher vibration intensities to challenge your muscles.
- Leg exercises: Lunges (3 sets of 10-12 repetitions per leg) with added resistance band for extra challenge.
- Cool-down (5 minutes): Static stretches for all major muscle groups.
Ideal intensity: Moderate to high vibration intensity, focusing on controlled movements. Duration: 3-4 times per week.
Advanced Workout Routine
This routine focuses on specific fitness goals, such as increasing power, endurance, and overall athleticism. It is for individuals with a strong fitness base and experience with vibration training.
- Warm-up (10 minutes): Dynamic stretching, plyometrics (jump squats, box jumps). Focus on explosive movements and increased heart rate.
- Vibration Platform Exercises (45-60 minutes):
- Full body circuits: Combine exercises like push-ups, squats, and rows, performed with varying vibration intensities to maximize muscle engagement. Incorporate plyometric exercises for enhanced power development.
- High-intensity interval training (HIIT): Alternate between high-intensity vibration exercises and rest periods for improved cardiovascular fitness and calorie burn.
- Cool-down (10 minutes): Static stretching, foam rolling for muscle recovery.
Ideal intensity: High vibration intensity, focusing on explosive movements and maximum muscle engagement. Duration: 4-5 times per week.
Sample Workout Schedule
Day | Time | Routine | Equipment |
---|---|---|---|
Monday | 6:00 PM | Beginner | Vibration platform |
Wednesday | 6:00 PM | Intermediate | Vibration platform, resistance bands |
Friday | 6:00 PM | Advanced | Vibration platform, resistance bands, weights |
Saturday | 9:00 AM | Beginner | Vibration platform |
Workout Routine for Seniors
This routine is designed with the specific needs of seniors in mind, prioritizing safety and gradual progression.
Exercise | Description | Sets/Reps | Duration |
---|---|---|---|
Standing Calf Raises | Stand on the platform, lift heels off the ground | 2 sets of 8-10 repetitions | 1 minute |
Seated Glute Bridges | Sit on the platform, engage glutes to lift hips off the platform | 2 sets of 8-10 repetitions | 1 minute |
Arm Circles | Perform forward and backward arm circles on the platform | 3 sets of 10-12 repetitions | 1 minute |
Gentle Leg Swings | Perform gentle leg swings on the platform, targeting hip flexors | 2 sets of 10-12 repetitions | 1 minute |
Ideal intensity: Low to moderate vibration intensity, focusing on controlled movements. Duration: 2-3 times per week.
Looking to shake up your workouts? Top-notch vibration platform machines are a game-changer! They’re perfect for targeting specific muscle groups and boosting your overall fitness. Speaking of game-changers, did you hear about Sabrina Ionescu joining the unrivaled 3 on 3 league? Sabrina Ionescu joins unrivaled 3 on 3 league That’s incredible! Regardless of your athletic goals, these platforms can enhance your workout routine and help you achieve your fitness objectives.
Integration into Existing Fitness Regimens
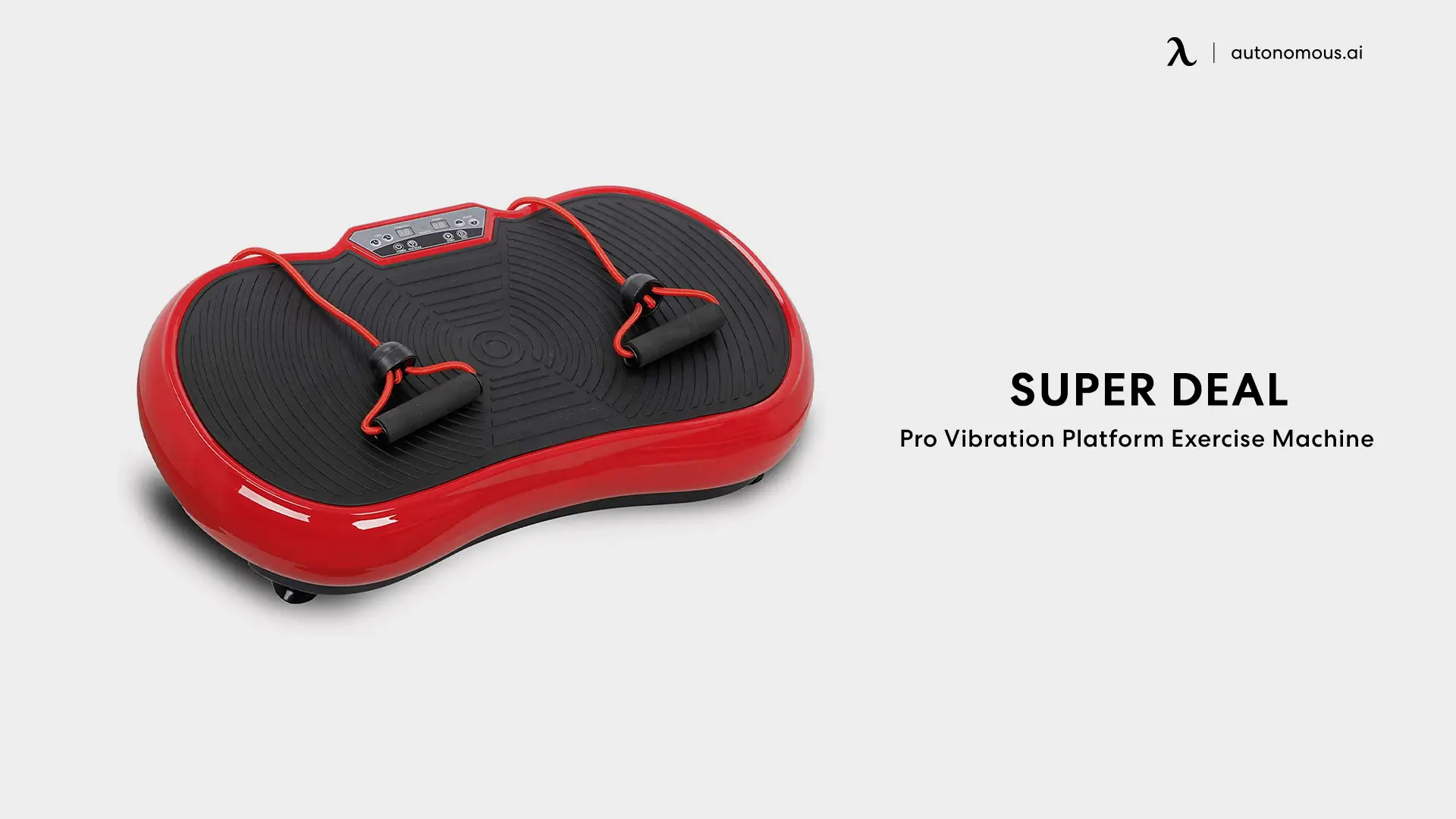
Integrating vibration platform machines into your existing fitness routine can significantly enhance your results and make your workouts more enjoyable. By strategically incorporating vibration platform workouts, you can optimize your training program and achieve a more well-rounded approach to physical fitness. This approach allows you to target specific muscle groups more effectively, while also maximizing the benefits of your existing routines.The key to successful integration lies in understanding how vibration platforms can complement, rather than replace, your current workout regime.
Careful planning and gradual progression are crucial for preventing injuries and maximizing the benefits. This involves adjusting the intensity, duration, and frequency of your workouts to fit your body’s response and overall fitness goals.
Methods for Incorporating Vibration Platforms
Vibration platforms can be seamlessly integrated into existing routines. Start with short, low-intensity sessions and gradually increase the duration and intensity as your body adapts. Incorporate vibration platform exercises as a warm-up, cool-down, or as a standalone segment within your workout.
Maximizing Benefits with Other Fitness Activities
Combining vibration platform workouts with other exercises like yoga or Pilates can create a synergistic effect. For example, incorporating vibration platform exercises before a yoga session can enhance muscle activation and improve flexibility. Similarly, using vibration platforms after Pilates can aid in muscle recovery and reduce soreness.
Gradual Intensity and Duration Increase
Begin with short sessions (5-10 minutes) at a low intensity setting. Gradually increase the duration of your sessions (up to 20-30 minutes) and the intensity level as your body adapts. Listen to your body and adjust your workout based on your individual response. Never force yourself to do more than you are comfortable with.
Rest and Recovery in Vibration Platform Workouts
Adequate rest and recovery are essential for preventing injuries and optimizing results. Allow for rest days between vibration platform workouts, similar to your other training sessions. Incorporate active recovery techniques like light cardio or stretching on rest days. Remember, rest is not a sign of weakness, but rather a vital component of any successful fitness program.
Combining Vibration Platform Workouts with Other Exercises
- Cardiovascular Training: Use vibration platforms as a warm-up or cool-down before or after running, cycling, or swimming. The increased blood flow and muscle activation can enhance your performance and recovery.
- Strength Training: Integrate vibration platform exercises between sets of weights or resistance training to improve muscle activation and reduce fatigue.
- Yoga and Pilates: Use vibration platforms as a preparatory exercise before a yoga or Pilates session to enhance flexibility and improve muscle activation. Post-workout, use vibration platforms to aid in muscle recovery and reduce soreness.
- Core Workouts: Incorporate vibration platform exercises into your core training routine to target deep abdominal muscles and improve stability.
- Bodyweight Exercises: Combine vibration platform exercises with bodyweight exercises to maximize muscle engagement and improve strength.
Visual Representation and Illustrations
Bringing vibration platform workouts into your routine can be a game-changer. Understanding the equipment and how it works is crucial for safe and effective use. Visual representations can make the process much clearer. From the platform’s mechanics to the exercises and safety considerations, visualization aids comprehension and empowers users to make informed decisions.
Vibration Platform Machine Description
A vibration platform machine is a stable, flat platform that oscillates at a specific frequency and amplitude. It typically has a motor-driven mechanism that produces these vibrations. The platform itself is usually made of a sturdy material, like steel or reinforced plastic, to withstand the vibrations and user weight. The design is often compact and allows for easy setup and storage.
Parts of a Vibration Platform Machine and Their Functions
- Platform: The base of the machine, responsible for the vibration transmission. It is typically constructed with a high-quality material for durability and safety.
- Motor/Mechanism: The component that generates the vibration. The motor’s power and frequency settings are critical for customized workouts.
- Control Panel: Allows users to adjust the intensity, frequency, and amplitude of vibrations. This panel usually displays the current settings for easy monitoring.
- Support Structure (if applicable): Some models have a supporting frame that surrounds the platform, offering additional stability.
The platform’s design and material selection directly impact the quality and effectiveness of the vibration. The control panel allows for tailored workouts to meet individual needs.
Examples of Exercises on a Vibration Platform
- Cardiovascular Exercises: Jumping jacks, high knees, butt kicks, and step-ups can be performed on the platform to increase the intensity and effectiveness of cardiovascular workouts. The vibrations can provide additional stimulus for the heart and circulatory system.
- Strength Training: Push-ups, squats, lunges, and rows can be done with added resistance bands or weights to enhance muscle engagement and strength gains. The vibrations can improve the range of motion and reduce the risk of injury.
- Flexibility and Balance Exercises: Stretching and balance exercises are particularly beneficial on a vibration platform. The vibrations can improve flexibility and enhance stability.
- Rehabilitation: Post-injury rehabilitation exercises can also be performed on a vibration platform. The vibrations can aid in reducing muscle soreness, improving blood circulation, and increasing range of motion.
These exercises, combined with appropriate vibration settings, can help build strength, improve cardiovascular health, and enhance flexibility.
Comparison of Vibration Platform Machines
Feature | Machine A | Machine B | Machine C |
---|---|---|---|
Platform Size | Large (suitable for multiple users) | Medium (suitable for single or two users) | Small (suitable for single user) |
Frequency Range | High (up to 50 Hz) | Medium (20-40 Hz) | Low (up to 30 Hz) |
Amplitude | Moderate | High | Low |
Weight Capacity | High (up to 300 lbs) | Medium (up to 250 lbs) | Low (up to 200 lbs) |
Price | High | Medium | Low |
Choosing the right machine depends on factors like your budget, the intended use, and the number of people using the machine.
Effects of Vibration Platform Workouts on the Body
- Increased Muscle Tone: The vibrations stimulate muscle fibers, leading to increased muscle tone and strength. This can improve posture and athletic performance.
- Improved Bone Density: Vibration training can stimulate bone cells, potentially increasing bone density and reducing the risk of osteoporosis. The impact on bone density is still under research and further studies are needed.
- Enhanced Blood Circulation: The vibrations can improve blood flow, potentially leading to reduced muscle soreness and quicker recovery times.
- Reduced Muscle Soreness: Vibrations can reduce inflammation and promote blood circulation, potentially reducing muscle soreness post-workout.
These effects, although promising, should be viewed in conjunction with a balanced diet and regular exercise regimen.
Safety Equipment for Vibration Platform Workouts
- Proper Footwear: Comfortable, supportive shoes are essential for stability and to prevent injuries.
- Non-Slip Mat: A non-slip mat underneath the platform can enhance safety and stability. This is crucial for preventing slips and falls.
- Appropriate Clothing: Comfortable, athletic clothing is recommended for maximum comfort and freedom of movement.
Proper safety precautions are paramount when using vibration platform machines. Always prioritize your safety and well-being during workouts.
Final Thoughts: Shake Up Your Workouts With The Best Vibration Platform Machines
In conclusion, vibration platform machines offer a dynamic and potentially transformative approach to exercise. We’ve explored the science behind vibration, the diverse workout routines possible, and the safety precautions to consider. From beginners to seasoned athletes, incorporating vibration platforms into your fitness journey can lead to improved strength, balance, and flexibility. Remember to consult with a healthcare professional before starting any new exercise program, especially if you have pre-existing conditions.
Ultimately, this guide provides the tools to make informed decisions about incorporating vibration platform machines into your workout routine, enhancing your overall fitness journey.