MS Office 365 alternatives offer a wealth of options for users seeking different features, pricing models, and functionalities. From cloud-based suites to open-source solutions and specialized tools, exploring the alternatives allows for a deeper understanding of the diverse landscape beyond the familiar Microsoft ecosystem. This exploration considers factors like ease of use, security, cost, and integration, providing a comprehensive overview to empower informed decisions.
This comprehensive guide dives into the various types of alternatives to Microsoft 365, examining their strengths and weaknesses to help you choose the best fit for your needs. We’ll look at cloud-based options, open-source alternatives, and specialized software, comparing their features, pricing, and security. We’ll also touch upon deployment and implementation strategies, and the crucial importance of a well-defined plan.
Introduction to Alternatives: Ms Office 365 Alternatives
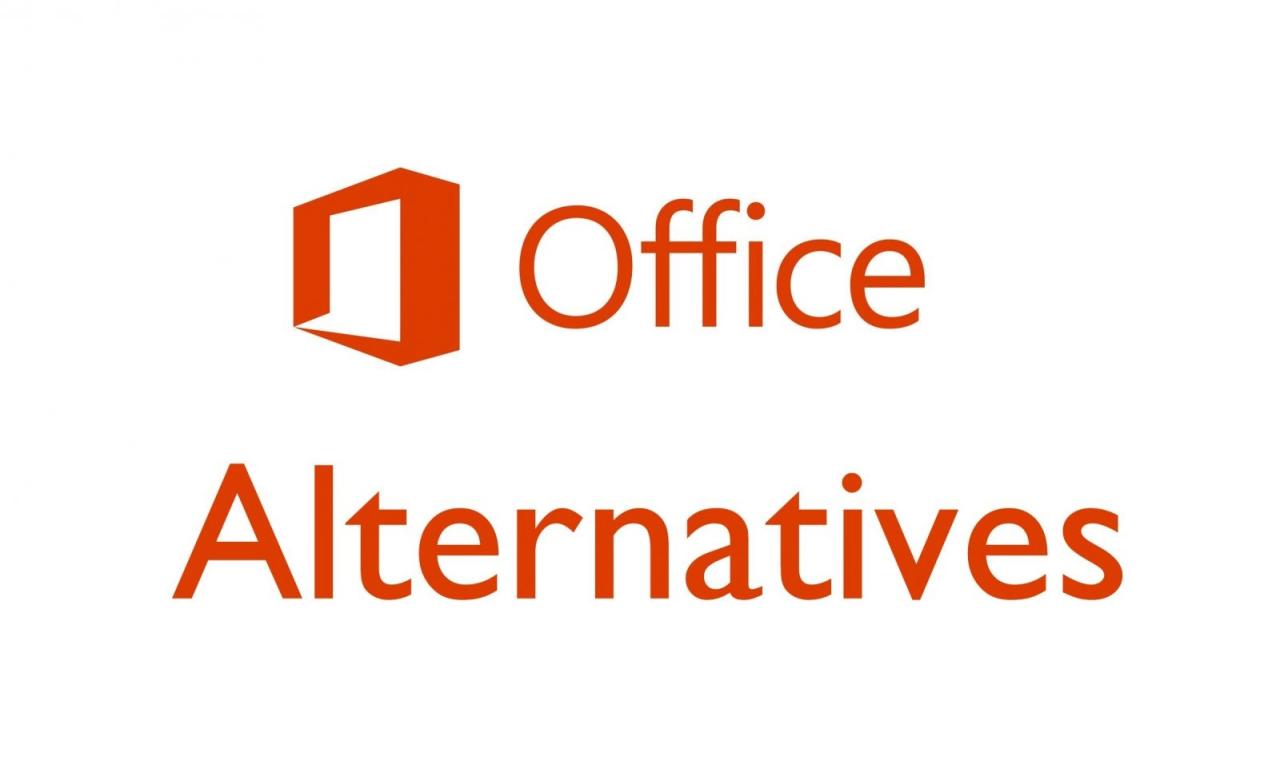
Microsoft 365 is a suite of productivity applications, encompassing word processing, spreadsheet, presentation, email, and cloud storage. It’s a ubiquitous platform for businesses and individuals, offering a comprehensive ecosystem for various tasks, from creating documents to collaborating on projects. Its centralized cloud-based architecture simplifies file sharing and access, enabling seamless teamwork.Common use cases for Microsoft 365 span a wide range of applications.
From individual writers and students creating documents and presentations to large corporations managing complex projects and communications, the versatility of the platform is apparent. It’s commonly used for business communication, project management, document creation, and data analysis.
Motivations for Seeking Alternatives
Users may seek alternatives to Microsoft 365 due to a variety of factors. Cost is a frequent concern, especially for individuals or small businesses operating on tighter budgets. Concerns about data privacy and security, as well as the perceived lack of control over data within Microsoft’s ecosystem, also motivate some users to explore alternatives. Furthermore, some users find the interface or functionality of Microsoft 365 to be less intuitive or efficient than their preferred alternatives.
Finally, some may be looking for a specific feature or integration that Microsoft 365 does not offer, driving the search for alternatives that better align with their needs.
Key Factors in Choosing an Alternative
When evaluating alternatives to Microsoft 365, users consider several key factors. Price and pricing models are paramount; some alternatives offer a variety of pricing plans, while others may be completely free. Ease of use and integration with existing workflows and tools are critical considerations. The level of security and data privacy features provided by the alternative is also a key factor, as is the degree of control the user maintains over their data.
Compatibility with existing devices and operating systems is essential. Finally, the level of support and customer service offered by the provider plays a crucial role in the decision-making process.
Looking for MS Office 365 alternatives? Well, real estate prices are soaring, just like the demand for robust productivity suites. A recent headline about a five bedroom home in San Jose selling for $1.9 million caught my eye. five bedroom home in san jose sells for 1 9 million 2 It’s a reminder that options like Google Workspace or LibreOffice are becoming increasingly attractive for their affordability and powerful features, giving a more budget-friendly alternative for those looking for MS Office 365 replacements.
Comparison of Core Features
This table provides a comparative overview of Microsoft 365’s core features versus a hypothetical alternative, “Office 365 Pro.” Note that “Office 365 Pro” is a fictional alternative for demonstration purposes only.
Feature | Microsoft 365 | Office 365 Pro |
---|---|---|
Word Processing | Microsoft Word with extensive features, cloud integration, and collaboration tools. | WordPro, a powerful word processor with a user-friendly interface, similar cloud integration, and collaborative editing capabilities. |
Spreadsheet | Microsoft Excel with robust formulas, charting tools, and data analysis capabilities. | SpreadsheetPro, a spreadsheet application with a comprehensive formula library, advanced charting options, and data visualization tools. |
Presentation | Microsoft PowerPoint with design templates, animations, and transitions. | PresentationPro, a presentation software with a vast collection of templates, intuitive animation options, and interactive elements. |
Cloud-Based Alternatives
Cloud-based office suites have become increasingly popular, offering accessibility and collaboration features that were previously unavailable or less accessible. These services often come with flexible pricing models and robust security features, appealing to businesses of all sizes. This section delves into the characteristics of popular cloud-based office suites, providing examples, comparing pricing, and highlighting their security measures.Cloud-based office suites offer a compelling alternative to traditional desktop software.
The ease of access, the ability to work collaboratively in real-time, and the scalability that cloud services offer make them an attractive option for individuals and organizations.
Popular Cloud-Based Office Suites
Several cloud-based office suites compete with Microsoft 365. Their strengths lie in different areas, from robust collaboration features to unique pricing models. These suites often provide a variety of tools to support productivity, including word processing, spreadsheets, presentations, and more.
- Google Workspace: Known for its integration with other Google services, Google Workspace offers a comprehensive suite of tools, including Docs, Sheets, Slides, and Gmail. It’s a strong contender, especially for users already familiar with the Google ecosystem. It also provides a free tier for individuals and small businesses, making it a cost-effective option for those on a budget.
- Microsoft 365 (with its cloud-based offering): While often considered a traditional desktop suite, Microsoft 365 also offers a cloud-based version. This allows for a seamless transition for existing users and provides a powerful suite of tools.
- Zoho Office Suite: Zoho Office Suite provides a comprehensive set of tools for individuals and businesses. It’s a reliable choice, with features like CRM integration, and a range of pricing plans to accommodate different needs.
- LibreOffice Online: A powerful, open-source alternative, LibreOffice Online is a viable choice for those seeking a free and open-source solution. Its features often mirror those of other suites, providing a comparable functionality, especially if you are already familiar with the desktop version of LibreOffice.
Pricing Models
Pricing models for cloud-based office suites vary significantly. Many offer tiered subscription plans based on the number of users, storage capacity, and required features. Free tiers are also common, though they often come with limited features and storage. Businesses need to carefully consider their needs and budget when selecting a cloud-based office suite.
- Google Workspace: Offers free tiers for individuals and small teams, with progressively more comprehensive plans for larger organizations and higher storage needs.
- Microsoft 365: Typically has a tiered structure with pricing increasing based on the number of users, features, and storage.
- Zoho Office Suite: Offers a variety of plans, ranging from free options to enterprise-level solutions with specific pricing models that can be adapted to different budgets and sizes of businesses.
- LibreOffice Online: Free and open-source, making it a cost-effective option for individuals and small businesses.
Security Features Comparison
The security of cloud-based data is crucial. Different suites employ various security measures to protect user data. A comparison of key security features is presented below.
Feature | Alternative A (Google Workspace) | Alternative B (Microsoft 365) | Alternative C (Zoho Office Suite) |
---|---|---|---|
Data Encryption | End-to-end encryption for certain features, like Gmail | Robust encryption protocols for data at rest and in transit | Data encryption protocols implemented across the suite |
Access Control | Fine-grained access controls with various permission levels | Extensive access controls, including multi-factor authentication | User roles and permissions to control data access |
Compliance Features | GDPR and CCPA compliance features | Extensive compliance features for HIPAA and other industry standards | Compliance features tailored to specific industry needs |
Open-Source Alternatives
Open-source office suites offer a compelling alternative to proprietary software like Microsoft 365, especially for budget-conscious users and organizations. They often provide powerful functionality, similar to their commercial counterparts, but without the subscription fees. This accessibility and flexibility have made open-source options increasingly popular, especially in educational and non-profit settings.These open-source suites typically rely on a community of developers and users for support, maintenance, and feature enhancements.
Looking for MS Office 365 alternatives? Plenty of options are out there, but the recent situation in San Diego, where Mexico is demanding a thorough investigation into the police shooting of a teenager, highlights the importance of strong accountability in tech, too. Mexico demands thorough investigation into san diego police shooting of teen brings into focus the need for transparency and fair processes, which should be reflected in the software we use.
Ultimately, finding a robust and reliable alternative to MS Office 365 is crucial for any business or individual.
This collaborative model fosters a dynamic environment, leading to continuous improvements and adaptability to evolving needs. However, the level of support and the speed of development can vary among different suites.
Advantages of Open-Source Suites
Open-source office suites boast numerous advantages. Their free nature is a significant draw, eliminating ongoing subscription costs. This cost-effectiveness is particularly beneficial for individuals, small businesses, and educational institutions. Furthermore, the open-source nature allows users to scrutinize the code, ensuring transparency and trust in the software’s operations. This transparent codebase facilitates customization to meet specific needs, allowing users to adapt the software to their unique workflow.
Disadvantages of Open-Source Suites
While open-source alternatives are attractive, they do present certain disadvantages. The level of support and features can vary between suites, and sometimes may not match the feature set of commercial options. Moreover, the user community’s size and responsiveness can influence the availability of resources and the speed of problem resolution. Lastly, maintaining open-source software can require technical expertise, which may be a barrier for some users.
Reputable Open-Source Office Suites
Several reputable open-source office suites provide compelling alternatives to commercial solutions. LibreOffice, a popular choice, offers a comprehensive suite of applications, including word processing, spreadsheets, presentations, and database management. Another notable option is Apache OpenOffice, which provides similar functionality. These options often have extensive documentation and a vast user community.
Community Support
The vibrant open-source community provides valuable support for these office suites. Online forums, documentation, and user groups offer avenues for troubleshooting, feature requests, and collaborative problem-solving. This active community ensures that users have access to assistance and resources to resolve issues.
Looking for MS Office 365 alternatives? Plenty of options are out there, but security is key. Consider the potential risks of using less-secure software, especially when dealing with sensitive data. For instance, fixing vulnerabilities in GraphQL systems, like fix graphql security vulnerabilities , is crucial. Ultimately, choosing the right alternative to MS Office 365 depends on your specific needs and security requirements.
Customization Options
Open-source solutions provide extensive customization options. The open nature of the code allows users to modify the software to fit their unique needs and workflows. This flexibility can extend to themes, templates, and even the underlying functionality, tailoring the software to specific industry requirements or personal preferences.
Compatibility with Different Operating Systems
The compatibility of open-source office suites across different operating systems is a key consideration. The table below highlights the compatibility of some popular open-source suites with common platforms:
OS | LibreOffice | Apache OpenOffice | Onlyoffice |
---|---|---|---|
Windows | Yes | Yes | Yes |
macOS | Yes | Yes | Yes |
Linux | Yes | Yes | Yes |
Specialized Alternatives
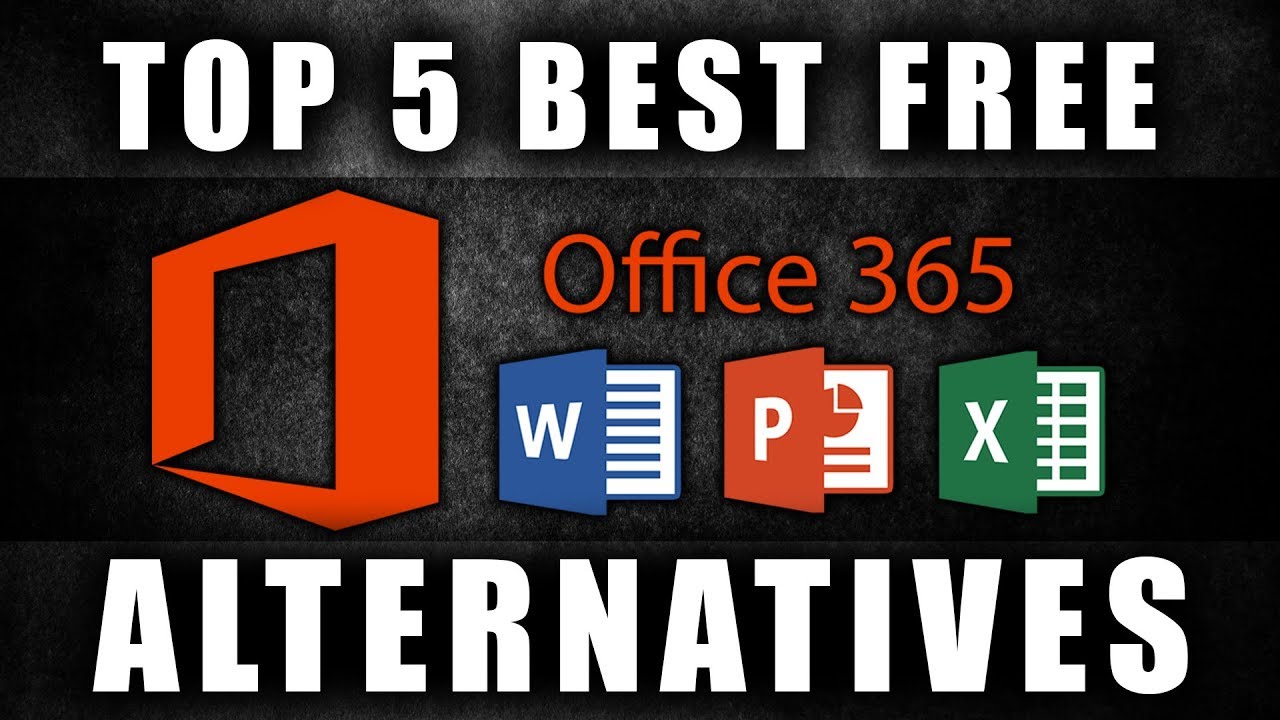
Microsoft 365 offers a comprehensive suite of tools, but sometimes, specialized software is better suited for specific tasks. Specialized alternatives excel in particular niches, providing advanced features and dedicated workflows for project management, graphic design, or other specific needs. This section explores these alternatives, comparing their strengths and weaknesses against their Microsoft 365 counterparts.Specialized software often offers superior functionality in their focused area, providing tools and workflows optimized for a specific task.
They can replace or supplement Microsoft 365 features, offering more efficient and effective solutions for particular project types.
Project Management Alternatives
Project management software plays a crucial role in organizing and executing projects. Specialized tools offer detailed task management, collaboration features, and progress tracking capabilities, often surpassing the project management features within Microsoft 365.
- Asana: Asana is a popular project management platform known for its user-friendly interface and customizable workflows. It excels in task assignment, progress tracking, and collaboration, making it a strong alternative to Microsoft To Do or even the project management aspects of Microsoft Teams.
- Jira: Jira, often favored by software development teams, is a powerful project management tool. Its robust issue tracking and agile methodologies make it a strong contender for project management, but it often comes with a steeper learning curve than Asana.
- Trello: Trello’s visual board structure and Kanban methodology provide a flexible and intuitive approach to project management. It’s ideal for visualizing tasks and tracking progress in a visual format, which can be an effective alternative to some of the more structured project management aspects of Microsoft 365.
Graphic Design Alternatives
Specialized graphic design software offers powerful tools for creating and editing visual content. These tools often surpass the basic image editing capabilities within Microsoft 365, offering more precision and advanced features.
- Adobe Photoshop: Photoshop remains the industry standard for image editing and manipulation. Its extensive features and precise control make it a powerful alternative to basic image editing in Microsoft Office.
- GIMP (GNU Image Manipulation Program): A free and open-source alternative to Photoshop, GIMP offers a comparable set of tools for image editing, making it a cost-effective choice for users looking for a free alternative.
- Canva: Canva provides a user-friendly interface for creating various visual content, including social media graphics, presentations, and posters. It’s an excellent option for non-designers or those needing quick visual assets, offering a simpler alternative to some of the more complex design tools.
Integration Capabilities
The ability to integrate with other software is crucial for workflow efficiency. Specialized alternatives often offer various integrations, enabling seamless data flow between tools.
Tool | Supported Integrations | Pricing Model | User Reviews |
---|---|---|---|
Asana | Google Calendar, Slack, Microsoft Teams, Dropbox, and many others | Various pricing tiers | Generally positive, emphasizing ease of use and flexibility |
Jira | Confluence, Bitbucket, Slack, and other development tools | Various pricing tiers | Generally positive, but with a steeper learning curve for some users |
Trello | Gmail, Slack, and other common business tools | Various pricing tiers | Generally positive, praised for its simplicity and visual approach |
Adobe Photoshop | Various plugins and integrations, but less integrated into general business workflows | Subscription-based | Generally positive, highly regarded for its power and features |
GIMP | Open-source integrations are common and extensive | Free | Positive, praised for its feature set and cost-effectiveness |
Canva | Various social media platforms and other design tools | Various pricing tiers | Positive, often praised for ease of use and accessibility |
Feature Comparison and Evaluation
Choosing an alternative to Microsoft Office 365 requires a thorough understanding of the features offered by each option. This section delves into the core functionalities of various alternatives, their ease of use, and the learning curve associated with each. Understanding the comparative strengths and weaknesses of different platforms is crucial for making an informed decision.A key consideration when evaluating alternatives is the alignment of features with specific needs.
Some alternatives might excel in collaboration tools, while others might prioritize document creation and editing. Careful assessment of feature availability and their functionality is paramount for a successful transition.
Key Features in Each Category
Various alternatives offer a wide range of features, spanning from basic word processing and spreadsheet capabilities to advanced collaboration and cloud storage. A clear understanding of the functionalities of each alternative is essential to determine the optimal fit for specific use cases.
- Cloud-Based Alternatives: These alternatives primarily focus on online document editing, collaboration tools, and cloud storage. Key features often include real-time co-authoring, version history, and integrated communication tools. Examples include Google Workspace, which offers a suite of applications like Docs, Sheets, Slides, and Meet; and Microsoft 365, itself a cloud-based solution with its own strengths and weaknesses.
- Open-Source Alternatives: Open-source alternatives often prioritize flexibility and customization. Key features include free access, open-source licensing, and the ability to modify software to meet unique requirements. Examples include LibreOffice, a popular suite of office applications offering compatibility with Microsoft Office file formats.
- Specialized Alternatives: Specialized alternatives target niche markets and cater to specific needs, such as graphic design, project management, or scientific data analysis. These tools often come with specialized features and functionalities tailored for particular tasks. Examples include Adobe Creative Cloud for graphic design, and specialized project management tools like Asana.
Feature Availability Comparison
A comprehensive comparison table helps visualize the availability of key features across different alternatives. This allows users to quickly identify which alternative best meets their specific requirements.
Feature | Google Workspace | LibreOffice | Adobe Creative Cloud | Specialized Project Management Tools |
---|---|---|---|---|
Word Processing | Yes | Yes | Limited (focus on design) | Limited (focus on project management) |
Spreadsheet | Yes | Yes | Limited (focus on design) | Limited (focus on project management) |
Presentation | Yes | Yes | Limited (focus on design) | Limited (focus on project management) |
Collaboration Tools | Excellent | Basic | Variable | Excellent |
Cloud Storage | Yes | Limited | Yes | Variable |
Ease of Use and User Experience
The ease of use and user experience of each alternative vary significantly. Intuitive interfaces and well-designed workflows contribute to a positive user experience. This factor directly impacts user adoption and satisfaction.
- Google Workspace: Known for its clean and intuitive interface, Google Workspace offers a user-friendly experience for most users, particularly those familiar with web-based applications. Learning the platform is straightforward.
- LibreOffice: LibreOffice, while functional, might present a slightly steeper learning curve for users accustomed to Microsoft Office’s interface. Familiarity with the basic functionalities is key to a positive user experience.
- Specialized Alternatives: Specialized alternatives often require a more in-depth understanding of the specific field they address. Ease of use depends on the user’s familiarity with the domain.
Learning Curve
The learning curve associated with adopting an alternative varies based on the user’s existing familiarity with similar software. Prior experience with comparable applications can significantly reduce the time needed to master new tools.
- Google Workspace: The learning curve is generally considered low, due to the platform’s intuitive design, particularly for those already using web-based applications.
- LibreOffice: The learning curve might be moderate for users accustomed to Microsoft Office, as there are some interface differences. However, the software’s compatibility with Microsoft Office files often shortens the transition period.
- Specialized Alternatives: Learning curves for specialized alternatives can vary greatly depending on the user’s familiarity with the relevant domain and industry terminology.
User Reviews
User reviews provide valuable insights into the practical experience of using different alternatives. Positive feedback often highlights the strengths of the application, while negative reviews point out areas for improvement.
- Google Workspace: User reviews often praise the ease of use and seamless collaboration features, but some users have reported occasional technical issues.
- LibreOffice: Reviews often highlight the functionality and free access, but some users miss certain features of Microsoft Office.
- Specialized Alternatives: Reviews for specialized alternatives tend to be highly specific to the particular industry or use case, providing valuable insights for targeted users.
Deployment and Implementation
Choosing an alternative to Microsoft Office 365 requires careful consideration of deployment and implementation strategies. Different solutions have varying complexities, technical requirements, and support structures. Understanding these factors is critical to ensuring a smooth transition and maximizing the benefits of the chosen alternative.Implementing a new office suite often involves more than just downloading software. Careful planning and execution are essential for minimizing disruption and maximizing user adoption.
This includes assessing current infrastructure, training staff, and managing potential data migration challenges.
Deployment Steps for Various Alternatives
The steps for deploying and implementing alternative office suites vary based on the chosen solution. Cloud-based alternatives typically involve setting up accounts, configuring access permissions, and migrating data. Open-source solutions may require installation on servers and potentially custom configuration. Specialized alternatives often require specific hardware or software dependencies. Careful consideration of these differences is vital to a successful deployment.
- Cloud-based alternatives: Typically involve creating user accounts, configuring access permissions, and migrating existing documents and data. Detailed instructions are often provided by the vendor, but the migration process may need specific attention based on the volume and type of data.
- Open-source alternatives: Frequently require installation on servers or workstations, and often involve configuring network settings and installing required packages. Specific instructions will vary depending on the chosen open-source solution.
- Specialized alternatives: Deployment may involve configuring specialized hardware or software components. Specific technical requirements and integration processes are often detailed by the vendor.
Technical Requirements for Different Solutions
The necessary technical requirements vary significantly depending on the chosen alternative. Cloud-based solutions may require a stable internet connection and suitable bandwidth, while open-source alternatives might need specific server configurations. Specialized solutions often demand specific hardware or software. Assessing these requirements in advance is crucial to avoid compatibility issues.
- Cloud-based alternatives: A stable internet connection and sufficient bandwidth are typically required. Data storage capacity and processing power in the cloud are factors to consider.
- Open-source alternatives: Server hardware, operating systems, and necessary software packages are often required. The specific requirements are often Artikeld in the documentation for each software.
- Specialized alternatives: These often necessitate specific hardware or software configurations and may require specialized skills for implementation and maintenance.
Support Resources for Each Alternative
The availability and quality of support resources can significantly impact the success of an implementation. Cloud-based alternatives typically offer extensive online documentation and user forums. Open-source solutions rely heavily on community support and forums, while specialized solutions may provide dedicated support contracts. Understanding the type and availability of support is essential.
- Cloud-based alternatives: Frequently offer comprehensive online documentation, tutorials, and community forums for assistance. Vendor support is usually readily available through various channels, such as phone support or email.
- Open-source alternatives: Rely heavily on online forums, user communities, and documentation. Finding solutions to problems may require research and engagement with the community.
- Specialized alternatives: Often provide dedicated support contracts or subscriptions to ensure timely assistance and problem resolution.
Migration Challenges and Strategies
Migrating data and workflows from an existing system to a new office suite can pose significant challenges. Data incompatibility, workflow adjustments, and user training are common issues. Effective migration strategies include thorough data analysis, careful planning, and phased implementation. Training users in advance can minimize disruption.
- Data incompatibility: Differences in file formats or data structures can lead to issues during migration. Using tools for data conversion and validation is crucial.
- Workflow adjustments: New systems require adjustments to existing workflows. Thorough documentation and training are essential to minimize disruption.
- User training: Users need training to adapt to the new system and maximize its functionalities. Phased rollouts and targeted training sessions can improve user adoption.
Planning for a Smooth Transition
Thorough planning is crucial for a smooth transition to a new office suite.
Planning includes detailed assessments of current infrastructure, data analysis, and user training. A phased implementation strategy can mitigate risks and minimize disruption. Documentation and communication are essential for a successful transition.
Cost Analysis and Return on Investment
Migrating to an alternative to Microsoft Office 365 hinges heavily on a thorough cost analysis. Understanding the pricing models, total cost of ownership (TCO), and potential return on investment (ROI) is crucial for informed decision-making. This section delves into these aspects, providing a framework for evaluating the financial viability of various alternatives.A well-defined cost analysis allows businesses to compare different options based on more than just features.
This comparative analysis considers not only initial licensing costs but also ongoing maintenance, support, and potential future upgrades. Predicting the ROI requires a realistic assessment of savings and increased efficiency, factors that are discussed in detail below.
Pricing Models
Different alternatives employ various pricing models. Some use a subscription-based model, similar to Microsoft 365, offering monthly or annual fees. Others might offer perpetual licenses, a one-time purchase. Still others use a tiered approach, with different packages catering to varying needs and user counts. Understanding these differences is key to aligning the chosen alternative with the budget and anticipated usage.
Total Cost of Ownership (TCO)
The TCO encompasses all expenses related to an alternative, extending beyond the initial purchase price. This includes ongoing support, training costs for staff, potential maintenance fees, hardware requirements, and the cost of data migration. An in-depth TCO analysis factors in the entire lifecycle of the software, ensuring a comprehensive view of the long-term financial commitment.
Return on Investment (ROI)
Calculating the ROI of adopting an alternative involves evaluating the potential savings and increased efficiency it brings. This includes cost savings from reduced licensing fees, increased productivity, and minimized IT support needs. Accurate ROI projections often rely on realistic estimations of user adoption, training time, and ongoing support costs.
Case Studies
Several businesses have successfully migrated to alternatives to Microsoft 365. One example is a small-sized company that replaced its expensive Microsoft 365 licenses with an open-source suite. The migration resulted in significant cost savings, allowing the company to reinvest funds in other growth initiatives. Another case involves a large corporation that switched to a cloud-based alternative, which streamlined its operations and improved collaboration across teams.
These real-world examples highlight the potential benefits and the importance of a thorough cost analysis.
Cost and ROI Comparison, Ms office 365 alternatives
Alternative | Licensing Cost | Total Cost of Ownership | ROI Potential |
---|---|---|---|
Alternative A (Cloud-based) | $100/user/month (variable based on features) | $1200/user/year (includes support, storage, and training) | 15-25% (estimated based on reduced licensing fees and increased productivity) |
Alternative B (Open-Source) | Free (initial download) | $500/user/year (includes customization, training, and hosting) | 20-30% (estimated based on cost savings and increased productivity) |
Alternative C (Specialized) | $200/user/month (variable based on specific features) | $2400/user/year (includes specialized support and customization) | 10-20% (estimated based on cost savings and increased efficiency in specific tasks) |
Note: The figures in the table are illustrative and may vary depending on the specific features, user needs, and implementation strategy. The ROI potential is an estimate and can vary greatly based on specific business contexts.
Ending Remarks
In conclusion, the options for replacing Microsoft 365 are extensive and varied. From the familiar cloud-based solutions to the powerful open-source alternatives and niche specialized tools, there’s a perfect fit for every user and budget. Careful consideration of features, costs, and long-term needs is key to finding the right alternative that best supports your workflow. Ultimately, the decision depends on your specific requirements and priorities.