Coding challenges to sharpen thinking provide a unique pathway to enhance problem-solving skills. They offer a structured environment to hone critical thinking, logical reasoning, and data manipulation skills. This exploration delves into various types of coding challenges, from algorithmic puzzles to logic-based problems, and examines the best approaches to tackle them. We’ll also look at the essential role of data structures and algorithms, as well as the importance of learning from mistakes and consistently practicing.
This in-depth look at coding challenges goes beyond simply solving problems. It also examines the broader implications for real-world problem-solving, career development, and the transferability of these skills to other domains. We’ll unpack the different strategies involved, from breaking down complex issues into manageable parts to recognizing patterns and relationships. Understanding these techniques can unlock a powerful toolkit for tackling any challenge.
Introduction to Coding Challenges
Coding challenges are designed to test and enhance problem-solving abilities in a programming context. They often involve writing code to solve specific problems, usually focusing on algorithms, data structures, and logical reasoning. These challenges are a valuable tool for aspiring programmers and experienced developers alike, pushing them to think critically and efficiently.
Definition of Coding Challenges
Coding challenges are tasks that require a programmer to implement algorithms and/or data structures to solve a given problem. They evaluate a candidate’s ability to analyze problems, design efficient solutions, and implement them in a programming language. These challenges frequently appear in interviews, online platforms, and competitions.
Types of Coding Challenges
Understanding the different types of coding challenges helps in focusing on the specific skillset required to tackle them. There are several categories, including algorithmic, logic, and data structure challenges.
- Algorithmic Challenges: These challenges focus on the design and implementation of algorithms. They often require candidates to choose the most efficient algorithm for a given task, considering time and space complexity. Examples include sorting, searching, graph traversal, and dynamic programming problems.
- Logic Challenges: These problems primarily assess the candidate’s ability to think logically and solve puzzles. They often involve deductive reasoning, pattern recognition, and problem decomposition. These challenges may not necessarily require writing code but might involve solving problems through reasoning and strategic thinking.
- Data Structure Challenges: These challenges concentrate on the use and manipulation of data structures. Candidates need to understand how to choose the appropriate data structure for a specific problem and use it effectively. Examples include implementing stacks, queues, linked lists, trees, and hash tables.
Popular Coding Platforms
Many online platforms provide coding challenges to aid in skill development and competitive programming.
- LeetCode: A popular platform known for its diverse range of coding challenges, covering various data structures and algorithms. It hosts a vast library of problems, making it a great resource for practice and learning.
- Codeforces: This platform is popular for its competitive programming contests. The challenges are usually more complex and challenging than those on other platforms.
- HackerRank: Another well-known platform with a variety of coding challenges and contests. It offers problems from various categories and provides a good environment for practice and skill enhancement.
- Codewars: This platform focuses on a more collaborative approach to learning. Users can solve problems, and the platform also provides a ranking system based on performance.
Benefits of Participating in Coding Challenges
Participating in coding challenges helps individuals sharpen their thinking skills in several ways. It promotes critical thinking, analytical skills, and the ability to design efficient solutions. This process fosters an understanding of algorithmic design, which is crucial in many areas of computer science and software development.
Challenge Type | Description | Difficulty Level | Platform |
---|---|---|---|
Algorithmic | Focuses on the design and implementation of algorithms, considering efficiency. | Medium to Hard | LeetCode, Codeforces |
Logic | Tests logical reasoning, pattern recognition, and problem decomposition. | Easy to Medium | HackerRank, Codewars |
Data Structure | Evaluates the ability to select and utilize appropriate data structures. | Medium | LeetCode, Codewars |
Competitive | Challenges involving timed coding contests, often more complex. | Hard | Codeforces |
Problem-Solving Strategies
Mastering coding challenges isn’t just about knowing programming languages; it’s about developing a systematic approach to problem-solving. This involves breaking down complex problems into smaller, more manageable components, identifying patterns, and comparing various strategies to find the most effective solution. Efficient problem-solving is a crucial skill in the world of coding.Effective problem-solving in coding challenges requires a structured approach.
This involves recognizing the problem’s core elements, understanding the constraints, and developing a clear plan of action before diving into the code. By breaking down the problem, one can focus on smaller, more manageable parts, improving the likelihood of success.
Decomposition and Modularization, Coding challenges to sharpen thinking
Breaking down complex problems into smaller, more manageable modules is a fundamental strategy. This approach simplifies the task by isolating specific functionalities. Each module focuses on a single aspect of the problem, allowing for easier testing and debugging. Modularity fosters reusability and maintainability, essential attributes for larger and more intricate projects. By decomposing a problem, one gains a clearer understanding of the individual parts, and how they interact to form the complete solution.
This decomposition improves code readability and efficiency.
Pattern Recognition
Identifying patterns and relationships within coding challenges is crucial. This involves recognizing recurring structures, data transformations, or algorithmic approaches. By identifying these patterns, one can develop more efficient solutions. For example, recognizing a recursive pattern in a problem suggests the potential use of a recursive algorithm. Identifying common data structures, such as arrays, linked lists, or trees, can help leverage pre-existing knowledge and techniques.
Patterns can reduce the need to reinvent the wheel, saving time and effort.
Coding challenges are fantastic for honing critical thinking skills, pushing your problem-solving abilities to the max. While thinking about complex logic in code, it’s easy to draw parallels to real-world scenarios, like the recent legal proceedings surrounding the Ohtani ex-interpreter sentencing. This case highlights the importance of clear communication and logical reasoning, skills that directly translate to tackling complex coding problems effectively.
The need for meticulous analysis and logical deduction in both coding and legal situations is quite striking. Ultimately, tackling coding challenges is a great way to keep those mental gears turning.
Algorithmic Approaches
Different algorithms offer various solutions to similar problems. Understanding the trade-offs between different algorithms, such as time complexity and space complexity, is essential. For instance, choosing a linear search over a binary search when the dataset is small might seem logical. However, the complexity of the search algorithm grows significantly with increasing dataset size. Understanding these trade-offs is key to selecting the most appropriate algorithm for a given situation.
Comparison of Approaches
A critical step is comparing and contrasting different approaches. This involves evaluating the strengths and weaknesses of each method to determine the most effective solution. For example, comparing a brute-force approach to a more sophisticated algorithm helps determine the best fit for a given challenge. This comparison is essential for avoiding unnecessary complexity and optimizing performance.
Problem-Solving Strategy Applicability Table
Problem-Solving Strategy | Description | Applicable Challenge Types | Example |
---|---|---|---|
Decomposition and Modularization | Breaking down complex problems into smaller, manageable parts. | Problems with multiple interdependent steps, complex logic. | Creating a program to manage user accounts. |
Pattern Recognition | Identifying recurring structures or algorithmic patterns. | Problems involving data manipulation, string processing, or common algorithms. | Solving a sequence of Fibonacci numbers. |
Algorithmic Approaches | Utilizing various algorithms like sorting, searching, and graph traversals. | Problems requiring efficient solutions for large datasets or complex computations. | Finding the shortest path in a graph. |
Comparison of Approaches | Evaluating the efficiency and feasibility of different solutions. | All types of coding challenges, where optimization is key. | Choosing the most efficient sorting algorithm for a large dataset. |
Importance of Data Structures and Algorithms: Coding Challenges To Sharpen Thinking
Mastering data structures and algorithms is crucial for effective problem-solving in coding challenges. They form the foundation for writing efficient and optimized code. Understanding how data is organized and manipulated, and how algorithms operate, allows you to tackle complex problems with precision and speed. This knowledge empowers you to select the most suitable tools for each challenge, leading to cleaner, more performant solutions.Choosing the right data structure and algorithm is like selecting the perfect tools for a job.
A screwdriver isn’t ideal for hammering a nail, and similarly, an inefficient algorithm can hinder the performance of your code. A well-chosen combination of data structure and algorithm can dramatically improve the efficiency and speed of your solution, often resulting in a significant difference between a solution that works and one that excels.
Significance of Data Structures in Coding Challenges
Data structures are fundamental to organizing and managing data in a way that allows for efficient access and manipulation. They dictate how data is stored and retrieved, which directly impacts the speed and complexity of your algorithms. A well-chosen data structure can simplify complex operations and lead to faster execution times. For instance, searching for an element in a sorted array is significantly faster than searching in an unsorted list.
Roles of Algorithms in Optimizing Code Performance
Algorithms provide the step-by-step instructions for processing data within a given data structure. They define the logic and operations required to solve a problem. Efficient algorithms are crucial for reducing the time and resources needed to execute a program, especially in coding challenges where performance is often a key factor. For example, using a more efficient sorting algorithm can drastically reduce the time it takes to sort a large dataset.
Choosing Appropriate Data Structures and Algorithms
The selection of an appropriate data structure and algorithm depends heavily on the specific problem and the characteristics of the data involved. Consider factors like the type of data, the frequency of operations (insertions, deletions, searches, etc.), and the desired performance. For example, if you need to frequently search for elements, a sorted array or a hash table might be more suitable than a linked list.
Analyzing the problem and understanding the required operations is paramount in making informed decisions.
Common Data Structures and Their Use Cases
Understanding the strengths and weaknesses of various data structures is essential for making the right choices. This knowledge allows you to choose the best tool for the job, which is vital in coding challenges where efficiency is a primary concern. The examples below highlight how different data structures excel in various scenarios.
Data Structure | Description | Use Cases in Coding Challenges |
---|---|---|
Array | A contiguous block of memory storing elements of the same data type. | Storing and accessing elements sequentially, implementing simple algorithms like linear search, storing collections of related data. |
Linked List | A linear data structure where elements are not stored contiguously. Each element points to the next. | Implementing stacks and queues, performing insertions and deletions at any position, representing graphs. |
Stack | A LIFO (Last-In, First-Out) data structure. | Evaluating arithmetic expressions, backtracking algorithms, function call management. |
Queue | A FIFO (First-In, First-Out) data structure. | Managing tasks in a specific order, implementing breadth-first search, simulating real-world scenarios. |
Hash Table | A data structure that uses hash functions to map keys to values. | Fast lookups, insertions, and deletions, implementing caching mechanisms, solving problems involving key-value pairs. |
Tree | A hierarchical data structure with a root node and child nodes. | Representing hierarchical data, implementing sorting algorithms like heapsort, solving problems with tree-like structures. |
Logical Reasoning and Critical Thinking
Mastering coding challenges isn’t just about knowing syntax; it’s about effectively applying logical reasoning and critical thinking. These skills are crucial for identifying patterns, understanding problem constraints, and devising efficient solutions. Without them, even the most technically proficient coder might struggle with complex problems. A strong foundation in these areas can significantly enhance problem-solving abilities and lead to more innovative and elegant code.Critical thinking is essential for dissecting problems, identifying hidden assumptions, and exploring alternative approaches.
Logical reasoning, on the other hand, provides the framework for structuring solutions, drawing conclusions, and verifying the correctness of those solutions. Both are indispensable tools for success in coding challenges, allowing you to navigate complex scenarios with clarity and precision.
Relationship between Logical Reasoning and Coding Challenges
Logical reasoning forms the bedrock of effective problem-solving in coding challenges. It allows you to break down complex problems into smaller, manageable parts, identify the underlying logic, and develop algorithms that address the core requirements. Without a strong grasp of logical reasoning, even the simplest challenges can become daunting tasks. The ability to systematically analyze problems, identify key variables, and establish relationships between them is crucial for constructing efficient and accurate solutions.
Role of Critical Thinking in Identifying Issues and Solutions
Critical thinking empowers you to not only understand the problem statement but also to question its assumptions, evaluate different potential solutions, and anticipate potential issues. This proactive approach is vital in avoiding common pitfalls and designing robust code that handles unexpected scenarios gracefully. Critical thinking allows you to assess the feasibility and efficiency of various approaches, enabling you to make informed decisions that maximize the quality of the solution.
Coding challenges are a fantastic way to flex your mental muscles and improve problem-solving skills. It’s all about figuring out creative solutions to complex problems. Knowing how to code effectively is a valuable skill, no matter what your chosen career path may be. For example, knowing the net worth of a professional athlete like Dansby Swanson, can be interesting to consider alongside the mental rigor of coding challenges.
Dansby Swanson net worth is a fascinating aspect of sports economics, but these challenges still provide a sharper, more focused approach to problem-solving.
Techniques for Improving Logical Reasoning Skills
Improving logical reasoning skills is a continuous process that requires dedicated practice. One effective technique is to actively engage with coding challenges, focusing on the underlying logic rather than just the code itself. Furthermore, dissecting existing code, analyzing its logic, and identifying potential improvements significantly enhances your problem-solving skills. Another powerful strategy is to work through various types of logic puzzles and brain teasers.
These exercises can hone your ability to identify patterns, draw conclusions, and develop strategies for solving intricate problems.
Application of Deductive and Inductive Reasoning
Deductive reasoning involves applying general principles to specific situations, drawing conclusions based on established facts. In coding, this might involve using known algorithms or data structures to solve a particular problem. Inductive reasoning, conversely, involves drawing general conclusions from specific observations. This is useful when exploring patterns in data or when attempting to optimize code based on observed performance characteristics.
Understanding both forms of reasoning is essential for navigating the complexity of coding challenges.
Examples of Logical Reasoning in Coding Challenges
Logical Reasoning Skill | Examples in Coding Challenges |
---|---|
Identifying Patterns | Finding the next number in a sequence, recognizing recurring patterns in data, or implementing algorithms based on observed trends. |
Analyzing Constraints | Considering the input size, data types, and any limitations imposed by the problem statement. This is vital to choose appropriate data structures and algorithms to prevent runtime errors. |
Developing Algorithms | Designing step-by-step procedures to solve a problem, considering various scenarios and edge cases, such as sorting, searching, and graph traversal algorithms. |
Evaluating Solutions | Determining the efficiency and correctness of proposed solutions. This involves considering factors like time complexity, space complexity, and potential errors. |
Learning from Mistakes and Feedback
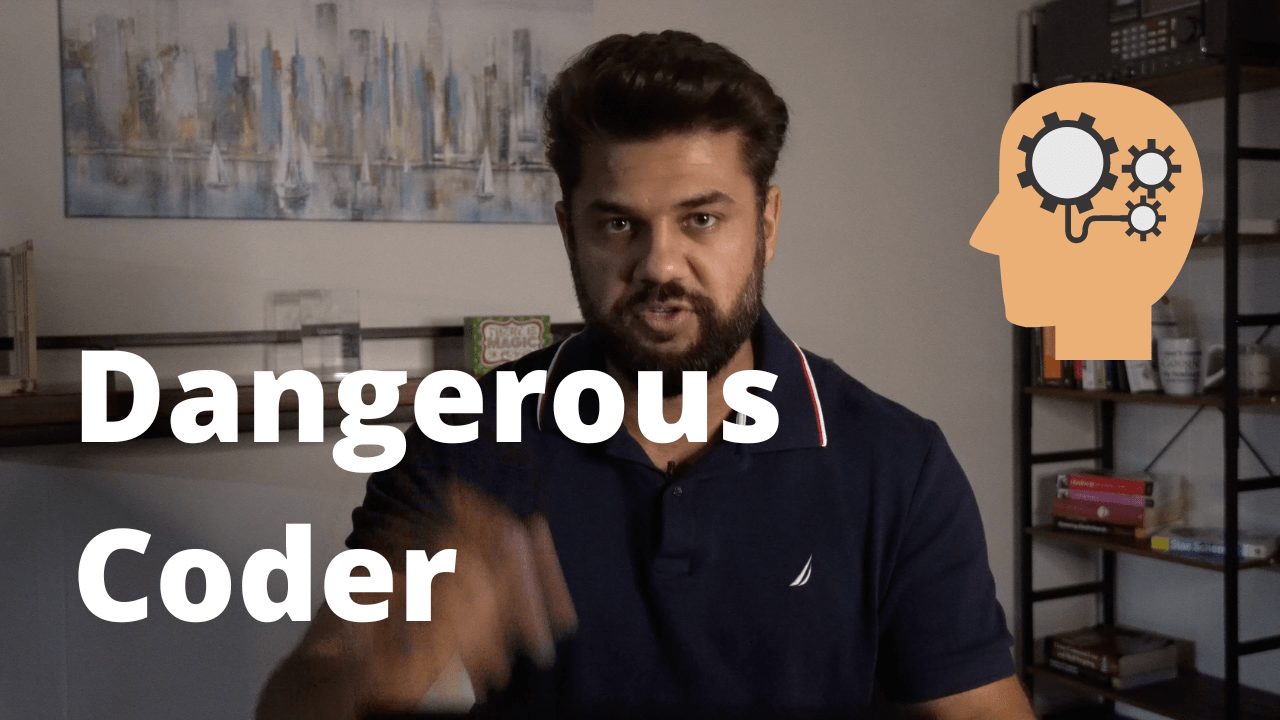
Coding challenges are valuable learning experiences, but the true power lies in how effectively we analyze our mistakes and leverage feedback. This process allows us to identify recurring patterns in our errors, pinpoint areas for improvement, and ultimately, enhance our problem-solving skills. Learning from these experiences is key to consistent progress in coding.Analyzing our mistakes is crucial for growth.
Each incorrect solution reveals insights into our thought processes and problem-solving approaches. By understanding the root cause of our errors, we can develop strategies to avoid repeating them in the future. This introspection is fundamental to becoming a more proficient programmer.
Importance of Analyzing Mistakes
Understanding the reasons behind coding errors is vital. Simply identifying a wrong answer is not enough. We need to delve deeper to understand why a particular approach failed. This involves examining our logic, data structures, algorithms, and overall problem-solving strategy. This proactive analysis prevents the repetition of similar errors.
Identifying Patterns in Mistakes
Keeping a record of coding challenges and the associated errors is highly beneficial. By noting recurring issues, we can identify common patterns in our mistakes. This pattern recognition helps in recognizing areas where our knowledge is weak or where our problem-solving strategies need refinement. A consistent pattern of errors, for instance, in handling input validation or using inappropriate data structures, signifies a need for targeted improvement.
Detailed documentation of the mistakes, including the problem statement, the attempted solution, the error, and the analysis of the error, is essential for identifying patterns.
Leveraging Feedback from Others
Constructive feedback from peers and mentors is invaluable in refining problem-solving skills. Different perspectives often reveal blind spots or alternative approaches that we might not have considered. Active listening and thoughtful consideration of feedback are crucial to gaining insights into potential improvements.
Using Constructive Criticism to Improve Performance
Constructive criticism, when approached with a growth mindset, can significantly boost our performance. It’s important to distinguish between criticism that is purely negative and feedback that focuses on improvement. By separating the constructive elements from the judgmental aspects, we can use the feedback to identify specific areas for enhancement. An example is a peer pointing out an inefficient algorithm used in a solution; this feedback allows for the adoption of a more optimized approach.
Example of Analyzing Mistakes
Common Mistakes | Causes | Solutions |
---|---|---|
Incorrect use of data structures | Insufficient understanding of data structures’ properties and suitability for specific problems | Review relevant data structure documentation and practice implementing them in various scenarios. Focus on understanding the trade-offs between different data structures. |
Logical errors in algorithms | Inaccurate reasoning or incomplete understanding of the problem statement | Thoroughly review the algorithm’s logic step-by-step. Use debugging tools to trace the flow of execution. Consider alternative problem-solving approaches or visualizations to gain a different perspective. |
Inefficient algorithms | Lack of optimization strategies and understanding of algorithm complexity | Study different algorithms and their time/space complexities. Learn about optimization techniques like memoization or dynamic programming. Practice implementing optimized algorithms for similar problems. |
Practice and Improvement
Consistent practice is crucial for sharpening your problem-solving skills in coding challenges. Just like any skill, coding requires dedicated effort and repetition to internalize concepts and develop efficient strategies. The more you practice, the more comfortable you become with various problem types and the quicker you can identify patterns and solutions.Regular practice, coupled with a strategic approach to selecting challenges and tracking progress, is essential for consistent improvement.
Coding challenges are a fantastic way to flex your problem-solving muscles, and it’s great to see athletes like George Kittle supporting fellow competitors. For instance, George Kittle is showing his support for his Swedish friend Filip Forsberg at the 4 Nations face off in Montreal, which is awesome. It’s inspiring to see how these challenges can sharpen our thinking, just like the dedication and support shown in sporting events like this one.
This translates well into the coding world where tackling complex problems becomes easier with practice.
This fosters a deeper understanding of algorithms, data structures, and logical reasoning, ultimately enhancing your problem-solving abilities.
Importance of Consistent Practice
Consistent practice is fundamental for developing coding proficiency and problem-solving skills. It allows you to internalize concepts, develop efficient problem-solving strategies, and improve your speed and accuracy. Each challenge you tackle reinforces your understanding, leading to a more robust skill set. Consistent engagement with coding challenges builds a strong foundation for tackling more complex problems in the future.
Setting Realistic Goals and Tracking Progress
Setting realistic goals is vital for maintaining motivation and tracking your progress effectively. Start with achievable targets and gradually increase the difficulty as you gain confidence and competence. Tracking your progress, whether through a journal, spreadsheet, or dedicated platform, allows you to monitor your improvement and identify areas needing further focus. This provides valuable feedback and helps you stay motivated.
Selecting Appropriate Challenges
Selecting appropriate coding challenges is crucial for skill development. Begin with problems aligned with your current skill level, gradually increasing the complexity as you progress. Focus on challenges that test your understanding of specific concepts, rather than overwhelming you with intricate details. Consider challenges that explore different data structures and algorithms, as this broadens your understanding of problem-solving techniques.
Maintaining Motivation During Practice
Maintaining motivation throughout your coding practice is essential. Find ways to make the process enjoyable, such as collaborating with peers, setting rewards for milestones, or exploring new areas of coding. Breaking down large challenges into smaller, manageable tasks can make the process less daunting and more motivating.
Practice Strategies for Improvement
Effective practice strategies are crucial for achieving consistent improvement. A structured approach helps you optimize your learning process and achieve tangible results.
Practice Strategy | Description | Time Commitment | Expected Results |
---|---|---|---|
Targeted Problem Solving | Focusing on specific problem types (e.g., array manipulation, graph traversal). | 1-2 hours per session, 2-3 sessions per week | Improved understanding of target concepts and efficient problem-solving approaches. |
Code Reviews | Reviewing and critiquing solutions to identify areas for improvement. | 1-2 hours per session, 2-3 sessions per week | Enhanced understanding of coding style, best practices, and potential optimization techniques. |
Algorithm Study | In-depth study of algorithms and their applications. | 1-2 hours per session, 1 session per week | Development of a broader understanding of algorithmic design principles. |
Coding Competitions | Participating in coding challenges and competitions. | Variable, depending on competition duration. | Increased problem-solving speed, adaptability, and experience with time-pressure environments. |
Coding Challenges and Real-World Applications
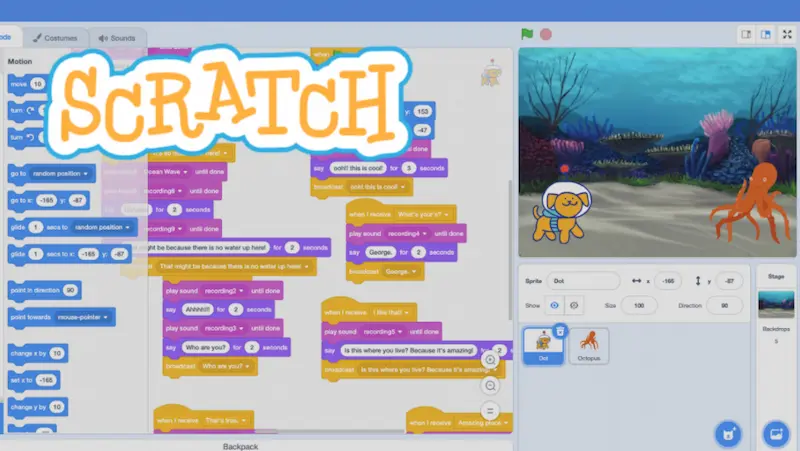
Coding challenges are more than just fun puzzles; they’re powerful tools for honing problem-solving skills applicable to diverse real-world scenarios. By tackling intricate coding problems, individuals develop critical thinking, logical reasoning, and a structured approach to problem decomposition – all highly valued skills in various professions. This exploration dives into the practical applications of coding challenges and their impact on career paths.Coding challenges, when approached strategically, provide a bridge between abstract concepts and tangible solutions.
The process of breaking down complex problems into smaller, manageable parts mirrors the approach needed in many real-world situations, fostering adaptability and resilience. The satisfaction of crafting elegant solutions to challenging problems directly translates to the fulfillment of solving real-world issues.
Real-World Problem-Solving Parallels
Coding challenges often involve simulating real-world scenarios. For example, optimizing algorithms to process large datasets mirrors the need to efficiently manage and analyze data in fields like finance, healthcare, and e-commerce. Challenges requiring the development of data structures for efficient searching and retrieval mirror the need for optimized database systems in various applications.
Transferability of Skills
The analytical and problem-solving skills honed through coding challenges are highly transferable. Logical reasoning, critical thinking, and the ability to identify patterns and formulate efficient solutions are applicable to non-technical domains. These skills are essential for tasks ranging from strategic planning and decision-making to effective communication and teamwork. For example, a programmer trained to think algorithmically can efficiently approach a project management problem by defining clear steps and prioritizing tasks.
Successful Applications of Coding Challenge-Driven Thinking
Coding challenges have fostered innovation and solutions in various fields. The development of machine learning algorithms for image recognition, a field driven by coding challenges, has revolutionized medical diagnosis, allowing for quicker and more accurate identification of diseases. Optimization algorithms developed through coding challenges are critical in supply chain management, leading to reduced costs and increased efficiency.
Impact on Career Prospects
Participating in coding challenges can significantly boost career prospects. Employers often view these challenges as a measure of problem-solving abilities, analytical skills, and technical aptitude. Demonstrating competence in tackling complex problems through coding challenges positions candidates as valuable assets. A strong coding challenge portfolio can increase visibility and marketability for software developers, data scientists, and other technical roles.
Coding Challenge Types and Real-World Applications
Coding Challenge Type | Real-World Application |
---|---|
Algorithm Optimization Challenges | Improving efficiency in data processing, logistics, and financial modeling. |
Data Structure Design Challenges | Developing efficient databases and storage systems for diverse applications, from e-commerce platforms to medical records management. |
Problem Solving Challenges with Constraints | Optimizing resource allocation in manufacturing, scheduling in project management, and traffic flow management. |
Competitive Programming Challenges | Developing high-performance systems in gaming, financial trading, and scientific simulations. |
Conclusion
In conclusion, engaging with coding challenges isn’t just about mastering code; it’s about cultivating a powerful problem-solving mindset. By understanding various problem-solving strategies, the importance of data structures and algorithms, and the value of learning from mistakes, we can significantly improve our ability to approach complex situations. Consistent practice and a willingness to adapt will allow us to transform coding challenges from exercises into invaluable tools for intellectual growth and career advancement.
This exploration has provided a comprehensive overview, leaving you with the knowledge and strategies to confidently tackle future challenges.